PHP is a scripting language widely used in Web development. It is good at processing HTTP requests and generating multimedia formats such as dynamic pages, images, and PDFs. In PHP, array is a very commonly used data type. The array function is a built-in function library for creating and manipulating arrays. This article will introduce the basic usage of php array function.
1. Create an array
Array is one of the most commonly used data structures in PHP. We can use the array function to create an array. The following is a common way to create an array:
//直接声明 $colors = array("red", "green", "blue"); //使用键值对 $age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43"); //空数组 $emptyArray = array();
2. Access the array
We have created the array, now let’s access the elements in it. In PHP, you can use array subscripts to access values within an array. The subscript can be a number or a string.
//访问数字下标 $colors = array("red", "green", "blue"); echo $colors[1]; //输出 "green" //访问字符串下标 $age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43"); echo $age["Ben"]; //输出 "37"
3. Modify and delete arrays
The next step is how to modify and delete array elements.
//修改数组元素 $colors = array("red", "green", "blue"); $colors[0] = "yellow"; //将第一个元素从"red"改为"yellow" print_r($colors); //输出 Array ( [0] => yellow [1] => green [2] => blue ) //删除数组元素 $age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43"); unset($age["Ben"]); //删除元素"Ben" print_r($age); //输出 Array ( [Peter] => 35 [Joe] => 43 )
4. Array traversal
When we need to operate on all elements in the array, we need to use a loop structure to traverse the array. PHP provides a variety of ways to traverse arrays, including for loops, foreach loops, while loops, etc.
//for循环遍历数组 $colors = array("red", "green", "blue"); for ($i = 0; $i "35", "Ben"=>"37", "Joe"=>"43"); foreach($age as $x => $x_value) { echo "Key=" . $x . ", Value=" . $x_value; } //while循环遍历数组 $colors = array("red", "green", "blue"); $i = 0; while($i <p><strong>5. Array sorting</strong></p><p>In PHP, we can use the built-in sort() function to sort the array. By default, the sort() function sorts the array in ascending order. If you need to sort in descending order, you can use the rsort() function. </p><pre class="brush:php;toolbar:false">//使用sort()函数升序排列数组 $numbers = array(4, 2, 8, 5, 1); sort($numbers); print_r($numbers); //输出 Array ( [0] => 1 [1] => 2 [2] => 4 [3] => 5 [4] => 8 ) //使用rsort()函数降序排列数组 $numbers = array(4, 2, 8, 5, 1); rsort($numbers); print_r($numbers); //输出 Array ( [0] => 8 [1] => 5 [2] => 4 [3] => 2 [4] => 1 )
6. Array merging
In PHP, we can use the built-in array_merge() function to merge multiple arrays into one array.
//使用array_merge()函数合并数组 $a1=array("red","green"); $a2=array("blue","yellow"); print_r(array_merge($a1,$a2)); //输出 Array ( [0] => red [1] => green [2] => blue [3] => yellow )
7. Array search
In PHP, we can use the in_array() function to determine whether an element exists in an array. This function returns true or false.
//使用in_array()函数查找数组 $colors = array("red", "green", "blue"); if(in_array("red",$colors)) { echo "found!"; } else { echo "not found!"; }
Generally speaking, the array function in PHP provides various practical methods to operate arrays, which can make us more convenient for web development. Proficient in these basic usages, you can get twice the result with half the effort in development.
The above is the detailed content of A brief analysis of how to use the array function in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
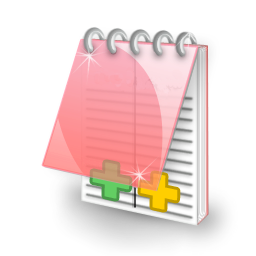
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
