With the development of the Internet and digital technology, music players have become an indispensable part of people's lives. PhongAPIOS technology based on JavaScript has gradually emerged in recent years and has become a viable option for developing music players.
PhongAPIOS is a front-end framework that can assist JavaScript developers to quickly build high-quality user interfaces and interactive effects. Using this framework, we can quickly implement a simple but fully functional music player through JavaScript. In this article, we will introduce how to use PhongAPIOS technology to implement a music player, and also introduce some common music player functions and application scenarios.
- Basic technologies and knowledge required to implement a music player
In order to implement a music player, we need to have the following basic technologies and knowledge:
- JavaScript basic syntax and data structure, including DOM operations, event processing, etc.;
- HTML5 audio tags, used to play music;
- CSS style sheets, to achieve the appearance of the player and interface effects.
Among them, JavaScript is the key technology to implement the music player. Using JavaScript, we can monitor user click events, control the status of the player, and implement some more complex functions, such as list looping, random play, etc.
- PhongAPIOS Framework Introduction
PhongAPIOS is a JavaScript-based front-end framework that can assist developers to quickly build high-quality user interfaces and interactive effects. Using PhongAPIOS, we can:
- Use rich UI components, such as buttons, input boxes and drop-down boxes;
- Achieve rich and flexible animation effects;
- Implement complex user interaction behaviors, such as dragging, sliding, etc.
PhongAPIOS also provides a powerful plug-in system that can easily enhance functions and extend the capabilities of the framework. At the same time, PhongAPIOS also has good documentation and community support, and you can get help and support quickly.
- Implementing music player based on PhongAPIOS
Based on the above technology and knowledge, we can start to implement the music player. Here are some common functions and implementation methods:
- Switch songs
Users can switch by clicking the song name in the list or the previous/next song button song. In the click event, we need to update the song name, cover and song source, and start playing a new song.
- Pause/Play Music
Users can control the play and pause of music by clicking the play/pause button. In the click event, we need to switch the button style according to the current state and update the song playing state.
- Adjust the volume
Users can adjust the volume of music by dragging the volume slider. In the slider drag event, we need to calculate the volume value based on the dragged position, and update the style of the volume bar and volume icon.
- Adjust progress
Users can adjust the music playback progress by dragging the progress bar. In the progress bar drag event, we need to calculate the current playback time based on the dragged position, and update the progress bar and time display.
- List loop/shuffle play
Users can switch playback modes by clicking the list loop and shuffle buttons. In the click event, we need to switch the button style according to the current state and update the player's play mode.
- Implementing a simple music player
The following is a sample code for a music player based on PhongAPIOS technology. You can refer to this example to implement your own player. :
nbsp;html> <meta> <title>PhongAPIOS 音乐播放器</title> <link> <link> <style> body {font-size: 18px;} .container {width: 300px; margin: 0 auto;} .now-playing {display: flex; align-items: center;} .cover {width: 80px; height: 80px; margin-right: 16px; border-radius: 50%;} .song-info {flex: 1;} .progress-bar {position: relative; height: 6px; background-color: #ddd; border-radius: 3px;} .progress {position: absolute; top: 0; left: 0; height: 100%; background-color: #f60; border-radius: 3px;} .controls {display: flex; justify-content: space-between; margin-top: 16px;} .btn {padding: 4px; font-size: 16px; cursor: pointer;} .btn:hover {background-color: #f60;} .btn.play i {transform: rotate(180deg);} .volume {position: relative; width: 100px; height: 6px; margin-top: 16px; background-color: #ddd; border-radius: 3px;} .volume-bar {position: absolute; top: 0; left: 0; width: 50%; height: 100%; background-color: #f60; border-radius: 3px;} .volume-icon {font-size: 16px; cursor: pointer;} .list-mode {text-align: center; margin-top: 16px;} .list-mode button {padding: 4px 12px; border-radius: 20px; cursor: pointer;} .list-mode button.active {background-color: #f60; color: #fff;} </style> <div> <h1 id="PhongAPIOS-音乐播放器">PhongAPIOS 音乐播放器</h1> <div> <img class="cover lazy" src="/static/imghwm/default1.png" data-src="https://via.placeholder.com/80x80.png?text=Cover" alt="How to implement a music player using PhongAPIOS technology" > <div> <div>歌曲名称</div> <div>歌手名称</div> </div> </div> <div> <div></div> </div> <div> <div><i></i></div> <div><i></i></div> <div><i></i></div> <div> <div></div> <i></i> </div> </div> <div> <button><i></i></button> <button><i></i></button> </div> </div> <script></script> <script> let audio = new Audio(); // 新建 Audio 对象 let playing = false; // 标记当前是否在播放 let playlist = [ {name: '歌曲 1', artist: '歌手 1', src: 'http://127.0.0.1:8000/song1.mp3', cover: 'https://via.placeholder.com/80x80.png?text=Cover 1'}, {name: '歌曲 2', artist: '歌手 2', src: 'http://127.0.0.1:8000/song2.mp3', cover: 'https://via.placeholder.com/80x80.png?text=Cover 2'}, {name: '歌曲 3', artist: '歌手 3', src: 'http://127.0.0.1:8000/song3.mp3', cover: 'https://via.placeholder.com/80x80.png?text=Cover 3'}, ]; // 播放列表 let current = 0; // 当前播放索引 let mode = 'cycle'; // 播放模式 let app = new PhongAPIOS({ el: '.container', methods: { togglePlay() { // 暂停/播放 if (playing) { audio.pause(); this.$refs.play.innerHTML = '<i class="fas fa-play">'; } else { audio.play(); this.$refs.play.innerHTML = '<i class="fas fa-pause">'; } playing = !playing; // 切换播放状态 this.startProgress(); // 开始更新进度 }, prevSong() { // 上一曲 current--; if (current < 0) current = playlist.length - 1; this.loadSong(playlist[current]); }, nextSong(random = false) { // 下一曲/随机 if (random) { // 随机播放 let index = Math.floor(Math.random() * playlist.length); this.loadSong(playlist[index]); } else { // 列表循环 current++; if (current >= playlist.length) current = 0; this.loadSong(playlist[current]); } }, loadSong(song) { // 加载歌曲 audio.src = song.src; this.$refs.cover.src = song.cover; this.$refs.name.innerHTML = song.name; this.$refs.artist.innerHTML = song.artist; this.startProgress(); if (playing) audio.play(); }, updateProgress() { // 更新进度 let progress = Math.floor((audio.currentTime / audio.duration) * 100); this.$refs.progress.style.width = progress + '%'; if (progress === 100) this.nextSong(); }, startProgress() { // 开始进度更新 this.stopProgress(); if (playing) this.timer = setInterval(() => this.updateProgress(), 500); }, stopProgress() { // 停止进度更新 clearInterval(this.timer); }, updateVolume(e) { // 更新音量 let x = e.pageX - this.$refs.volume.offsetLeft; let volume = x / this.$refs.volume.offsetWidth; audio.volume = volume; this.$refs.volumeBar.style.width = volume * 100 + '%'; }, toggleMode() { // 切换播放模式 let btnCycle = this.$refs.btnCycle; let btnRandom = this.$refs.btnRandom; if (mode === 'cycle') { // 切换为随机 mode = 'random'; btnCycle.classList.remove('active'); btnRandom.classList.add('active'); } else { // 切换为列表循环 mode = 'cycle'; btnCycle.classList.add('active'); btnRandom.classList.remove('active'); } }, }, mounted() { audio.addEventListener('ended', () => { // 播放结束自动切下一曲 if (mode === 'random') this.nextSong(true); else this.nextSong(false); }); this.loadSong(playlist[current]); // 加载第一首歌曲 this.$refs.volume.addEventListener('click', e => { // 点击音量条调整音量 this.updateVolume(e); }); this.$refs.volume.addEventListener('mousemove', e => { // 拖拽音量条调整音量 if (e.buttons !== 1) return; this.updateVolume(e); }); this.$refs.btnCycle.classList.add('active'); // 默认是列表循环 this.$refs.btnMode.forEach(btn => { // 绑定切换播放模式事件 btn.addEventListener('click', this.toggleMode); }); } }); </script>
- Conclusion
In this article, we introduced how to use PhongAPIOS technology to implement a simple music player. In fact, using PhongAPIOS makes it easier to implement other complex front-end applications, such as shopping carts, social applications, etc. Therefore, mastering PhongAPIOS technology will be an important step for you to advance in front-end development.
The above is the detailed content of How to implement a music player using PhongAPIOS technology. For more information, please follow other related articles on the PHP Chinese website!

React’s popularity includes its performance optimization, component reuse and a rich ecosystem. 1. Performance optimization achieves efficient updates through virtual DOM and diffing mechanisms. 2. Component Reuse Reduces duplicate code by reusable components. 3. Rich ecosystem and one-way data flow enhance the development experience.

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
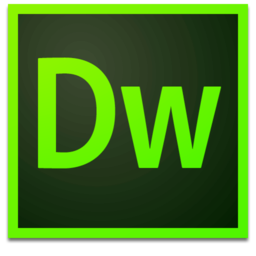
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.