1. Introduction
The main design goal of float and double types is for scientific calculations and engineering calculations. They perform binary floating-point operations, which are carefully designed to provide fast approximate calculations with high accuracy over a wide range of values. However, they do not provide completely accurate results and should not be used where accurate results are required. However, business calculations often require accurate results, and BigDecimal comes in handy at this time.
2. Introduction to knowledge points
1. Overview
2. Construction method
3. Operations of addition, subtraction, multiplication and division
4. Source code description
5. Summary
6. Refining exercises
Code demonstration:
package com.Test; import Test2.MyDate; import java.awt.*; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; import java.util.GregorianCalendar; import java.util.Properties; public class Main { private final static String name = "磊哥的java历险记-@51博客"; public static void main(String[] args) { System.out.println(0.2 + 0.1); System.out.println(0.3 - 0.1); System.out.println(0.2 * 0.1); System.out.println(0.3 / 0.1); System.out.println("============="+name+"============="); } }
- (1) public BigDecimal(double val): Convert double representation to BigDecimal (note: not recommended)
- (2) public BigDecimal(int val): Convert int representation to BigDecimal
- (3) public BigDecimal(String val): Convert String Convert the representation to BigDecimal
Code demonstration:
package com.Test; import Test2.MyDate; import java.awt.*; import java.math.BigDecimal; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; import java.util.GregorianCalendar; import java.util.Properties; public class Main { private final static String name = "磊哥的java历险记-@51博客"; public static void main(String[] args){ BigDecimal bigDecimal =new BigDecimal(2); BigDecimal bDouble = new BigDecimal(2.3); BigDecimal bString = new BigDecimal("2.3"); System.out.println("bigDecimal="+ bigDecimal); System.out.println("bDouble="+ bDouble); System.out.println("bString="+ bString); System.out.println("============="+name+"============="); } }
The running results are as follows:
of BigDecimal Code demonstration:
package com.Test; import Test2.MyDate; import java.math.BigDecimal; public class Main { private final static String name = "磊哥的java历险记-@51博客"; public static void main(String[] args) { BigDecimal bDouble1 =BigDecimal.valueOf(2.3); BigDecimal bDouble2 = new BigDecimal(Double.toString(2.3)); System.out.println("bDouble1="+ bDouble1); System.out.println("bDouble2="+ bDouble2); System.out.println("============="+name+"============="); } }
The results are as follows:
- (1) public BigDecimal add(BigDecimal value); addition
- (2) public BigDecimal subtract(BigDecimal value); //Subtraction
- (3) public BigDecimal multiply(BigDecimal value); //Multiplication (4) public BigDecimal divide(BigDecimal value); Division
Code demonstration:
package com.Test; import Test2.MyDate; import java.math.BigDecimal; public class Main { private final static String name = "磊哥的java历险记-@51博客"; public static void main(String[] args){ BigDecimal a = new BigDecimal("4.5"); BigDecimal b = new BigDecimal("1.5"); System.out.println("a+ b =" + a.add(b)); System.out.println("a- b =" + a.subtract(b)); System.out.println("a* b =" + a.multiply(b)); System.out.println("a/ b =" + a.divide(b)); System.out.println("============="+name+"============="); } }
In fact, the divide method can pass three parameters:
public BigDecimal divide(BigDecimal divisor, int scale, introundingMode) 第一参数表示除数, 第二个参数表示小数点后保留位数, 第三个参数表示舍入模式,只有在作除法运算或四舍五入时才用到舍入模式,有下面这几种
(1)ROUND_CEILING //向正无穷方向舍入
(2)ROUND_DOWN //向零方向舍入
(3)ROUND_FLOOR //向负无穷方向舍入
(4)ROUND_HALF_DOWN //向(距离)最近的一边舍入,除非两边(的距离)是相等,如果是这样,向下舍入, 例如1.55 保留一位小数结果为1.5
(5)ROUND_HALF_EVEN //向(距离)最近的一边舍入,除非两边(的距离)是相等,如果是这样,如果保留位数是奇数,使用ROUND_HALF_UP,如果是偶数,使用ROUND_HALF_DOWN
(6)ROUND_HALF_UP //向(距离)最近的一边舍入,除非两边(的距离)是相等,如果是这样,向上舍入, 1.55保留一位小数结果为1.6
(7)ROUND_UNNECESSARY //计算结果是精确的,不需要舍入模式
(8)ROUND_UP //向远离0的方向舍入
按照各自的需要,可传入合适的第三个参数。四舍五入采用 ROUND_HALF_UP
需要对BigDecimal进行截断和四舍五入可用setScale方法,例:
代码演示:
public static void main(String[] args) { BigDecimal a = newBigDecimal("4.5635"); //保留3位小数,且四舍五入 a = a.setScale(3,RoundingMode.HALF_UP); System.out.println(a); }
注:减乘除其实最终都返回的是一个新的BigDecimal对象,因为BigInteger与BigDecimal都是不可变的(immutable)的,在进行每一步运算时,都会产生一个新的对象
代码演示:
package com.Test; import Test2.MyDate; import java.math.BigDecimal; public class Main { private final static String name = "磊哥的java历险记-@51博客"; public static void main(String[] args){ BigDecimal a = new BigDecimal("4.5"); BigDecimal b = new BigDecimal("1.5"); a. add(b); System.out.println(a); //输出4.5. 加减乘除方法会返回一个新的 System.out.println("============="+name+"============="); } }
5、总结
(1)商业计算使用BigDecimal。
(2)尽量使用参数类型为String的构造函数。
(3)BigDecimal都是不可变的(immutable)的,在进行每一步运算时,都会产wf 所以在做加减乘除运算时千万要保存操作后的值。
(4)我们往往容易忽略JDK底层的一些实现细节,导致出现错误,需要多加注意。
6、精炼练习
在银行结算或支付中,我们经常会用到BigDecimal的相关方法。
6.1 题目
(1)使用BigDecimal创建出浮点类型的数字
(2)使用BigDecimal进行加减乘除运算
6.2 实验步骤
(1)声明一个类Test
(2)在Test类中,完成BigDecimal的构造和方法使用
代码演示:
package com.Test; import Test2.MyDate; import java.math.BigDecimal; public class Main { private final static String name = "磊哥的java历险记-@51博客"; public static void main(String[] args){ BigDecimal number = new BigDecimal("3.14"); //加法 System.out.println(number.add(new BigDecimal("1"))); //减法 System.out.println(number.subtract(new BigDecimal("1"))); //乘法 System.out.println(number.multiply(new BigDecimal("2"))); //除法 System.out.println(number.multiply(new BigDecimal("3.14"))); System.out.println("============="+name+"============="); } }
The above is the detailed content of Java BigDecimal class application example code analysis. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
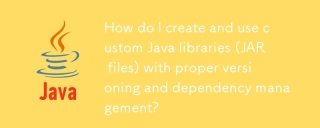
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
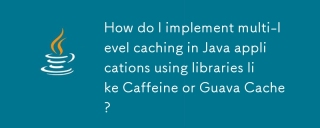
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
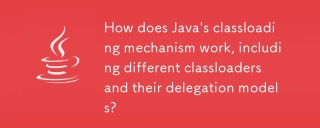
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
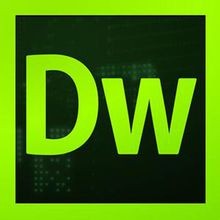
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.