As a strongly typed interpreted language, PHP provides programmers with a lot of conveniences in data processing. Array is a commonly used data type in PHP and is often used to store a series of related data. In actual programming, we often need to know how much data is contained in an array. So, this article will delve into how to get the total number of an array in PHP.
1. Use the count statement
PHP provides a very simple way to get the total number of an array, which is to use the count statement. The count statement is a very commonly used statement in PHP, which is used to count the number of elements in an array.
For example, we have the following array:
$array = array("apple", "banana", "orange", "pear");
Then we can use the count statement to get the number of its elements, as shown below:
$count = count($array); echo $count;
The output result is:
4
Similarly, we can also use the count statement for a two-dimensional array to get the total number of elements inside it.
$array = array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) ); $count = 0; foreach ($array as $value) { $count += count($value); } echo "总数为" . $count;
The output result is:
总数为9
2. Use the sizeof statement
In addition to the count statement, we can also use the sizeof statement in PHP to get the number of elements of the array. The only difference between sizeof and count is that sizeof is just an alias for count.
For example, we have the following array:
$array = array("apple", "banana", "orange", "pear");
We can use the sizeof statement to get the total number of elements, as shown below:
$count = sizeof($array); echo $count;
The output result is:
4
Similarly, we can also use the sizeof statement to obtain the total number of internal elements in a two-dimensional array.
$array = array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) ); $count = 0; foreach ($array as $value) { $count += sizeof($value); } echo "总数为" . $count;
The output result is:
总数为9
However, it is worth noting that although sizeof is an alias of count, in some contexts, such as in a for loop, the performance of sizeof may be superior. in count.
3. Use the number of variables to calculate the total number of elements
In PHP, in addition to using the count and sizeof statements to obtain the total number of elements of the array, you can also calculate it through the number of variables.
For example, we have the following array:
$array = array("apple", "banana", "orange", "pear");
We can use the following method to get the total number of elements:
$count = 0; foreach ($array as $key => $value) { $count++; } echo $count;
The output result is:
4
Similarly, we can also obtain the total number of elements inside the two-dimensional array through the number of variables.
$array = array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) ); $count = 0; foreach ($array as $key1 => $value1) { foreach ($value1 as $key2 => $value2) { $count++; } } echo "总数为" . $count;
The output result is:
总数为9
Conclusion
The above are the three methods of how to get the total number of array elements in PHP. In actual programming, we can choose the appropriate method to obtain the total number of array elements according to the specific situation. Whether it is count, sizeof or the number of variables, they are all very simple and feasible methods, and the calculation speed is also very fast.
The above is the detailed content of How to know the total number of array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
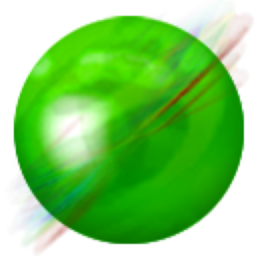
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
