For more and more apps nowadays, long pressing an element to pop up the operation has become a very common operation method. Today, we will talk about how to implement long-press pop-up deletion in development using uniapp.
- Defining elements in HTML
First, we need to define the elements that need to be operated on in HTML. In this example, we can use v-for
to generate a list, and then each list item should contain the action we need, such as a delete button. The HTML code is as follows:
<template> <div> <div> <div>{{ item.name }}</div> <button>删除</button> </div> </div> </template>
where list
is an array, representing the data we need to display, item
is a reference to each item in the array, index
is the index of the current item in the array. Each list item needs to contain a div
element that displays the name, and a button to delete the item.
- Bind events to elements
Next, we need to bind events to the elements we just defined. We need to bind a @longpress
event, which will be triggered when the user long presses the element. At the same time, we also need to record the index of the item that the user long-pressed, so that we can use it in the pop-up delete operation. The HTML code is as follows:
<template> <div> <div> <div>{{ item.name }}</div> <button>删除</button> </div> </div> </template>
<script> export default { data() { return { list: [{ name: "item 1" }, { name: "item 2" }, { name: "item 3" }], // 列表数据 longPressIndex: null // 长按的项的下标 }; }, methods: { showMenu(index) { this.longPressIndex = index; // TODO: 显示删除操作的菜单 }, deleteItem(index) { // TODO: 删除列表项 } } }; </script>
As shown above, we record the index of the currently long-pressed item in the showMenu
method, and then we can use it in the pop-up delete operation.
- Pop up the delete operation menu
The next thing we need to achieve is the highlight: pop up the delete operation menu. We can use the uni.showActionSheet
API provided by uniapp to achieve this. We can call it in the showMenu
method to pop up the menu. The code is as follows:
<script> export default { data() { return { list: [{ name: "item 1" }, { name: "item 2" }, { name: "item 3" }], // 列表数据 longPressIndex: null // 长按的项的下标 }; }, methods: { showMenu(index) { this.longPressIndex = index; uni.showActionSheet({ itemList: ["删除"], success: res => { if (res.tapIndex === 0) { this.deleteItem(this.longPressIndex); } } }); }, deleteItem(index) { this.list.splice(index, 1); } } }; </script>
Now, we have successfully implemented the function of long-press pop-up deletion operation. When the user long presses a list item, a menu will pop up. After the user selects delete, the item is deleted from the list.
Summary
Through the above method, we can easily implement the long press pop-up deletion operation in uniapp. However, it should be noted that the long press operation may be different on different platforms, so it needs to be handled for different platforms. Especially in mini programs, when implementing a long press operation, you need to call the mini program API instead of the API provided by uniapp. At the same time, it is also necessary to note that the API used by different versions of uniapp may also be different.
The above is the detailed content of How to use uniapp to implement long press pop-up deletion operation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
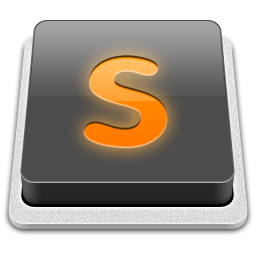
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
