When writing a JavaScript clock, we often need to display time information such as hours, minutes, seconds, etc. However, since the values of hours, minutes, and seconds are usually less than two digits, a padding operation is required so that the clock can be displayed normally. This article will introduce methods and techniques on how to use JavaScript to perform clock filling operations.
JavaScript clock basics
Before we start discussing the clock filling operation, we need to first understand how to use JavaScript to create a basic clock. The following is a simple JavaScript clock sample program:
function updateClock() { var now = new Date(); var hour = now.getHours(); var minute = now.getMinutes(); var second = now.getSeconds(); document.getElementById("clock").innerHTML = hour + ":" + minute + ":" + second; } setInterval(updateClock, 1000);
In the above code, we first created an updateClock
function, which passes the new Date()
function Get the current time and extract the hours, minutes and seconds respectively. Then, we use the document.getElementById()
function to get the clock element in the HTML page and set its content to the current time. Finally, we use the setInterval()
function to call the updateClock
function every 1 second to achieve real-time updates of the clock.
Clock filling methods and techniques
In the above code, we can see that if the hour, minute or second value of the current time is less than two digits, there will be an unsightly appearance. display effect. For example, when the minute number is 9, the clock displays "10:9:35" instead of "10:09:35". In order to fix this problem, we need to perform a clock padding operation. The following are two common filling methods and techniques:
- Using string filling
function zeroPadding(num, length) { return ('0' + num).slice(-length); }
In the above code, we define a file called The function of zeroPadding
, which accepts two parameters: num
represents the value to be filled, and length
represents the number of digits after filling. Inside the function, we first convert the value to a string, then add a 0 in front and use the slice()
function to get the string with the specified number of digits. For example, when we need to pad the minutes to two digits, calling zeroPadding(9, 2)
will return a string "09"; when we need to pad the seconds to three digits , calling zeroPadding(35, 3)
will return a string "035".
- Use number formatting
function formatNumber(num, length) { return num.toLocaleString(undefined, {minimumIntegerDigits: length}); }
In the above code, we define a function named formatNumber
, which accepts two parameters : num
represents the value to be filled, length
represents the number of digits after filling. Inside the function, we use the toLocaleString()
function new in ECMAScript 6 to format the value into a string with the specified number of digits. minimumIntegerDigits
option specifies the minimum number of integer digits.
The following is a sample code that uses the above padding methods and techniques to improve the above JavaScript clock program:
function updateClock() { var now = new Date(); var hour = zeroPadding(now.getHours(), 2); var minute = zeroPadding(now.getMinutes(), 2); var second = zeroPadding(now.getSeconds(), 2); var timeStr = hour + ":" + minute + ":" + second; document.getElementById("clock").innerHTML = timeStr; } setInterval(updateClock, 1000);
It should be noted that in the above code we used zeroPadding
Function performs filling operation. If you prefer to use the formatNumber
function, simply replace the line containing zeroPadding
in the above code with the following:
var hour = formatNumber(now.getHours(), 2); var minute = formatNumber(now.getMinutes(), 2); var second = formatNumber(now.getSeconds(), 2);
Summary
When writing JavaScript clocks, clock filling operation is a basic skill. This article introduces you to two common padding methods and techniques: string padding and number formatting. No matter which method you use, the clock filling operation can be easily implemented, making your JavaScript clock program more beautiful and practical.
The above is the detailed content of How to use JavaScript to perform clock padding operations. For more information, please follow other related articles on the PHP Chinese website!
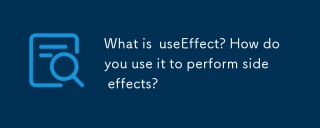
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
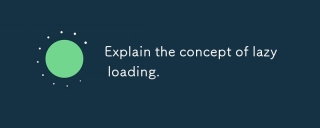
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
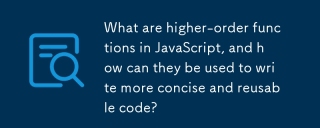
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
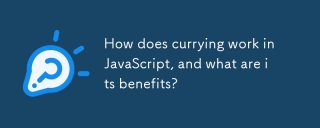
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
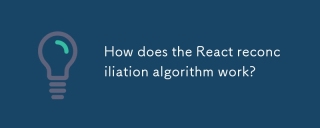
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
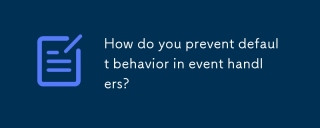
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
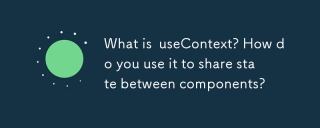
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
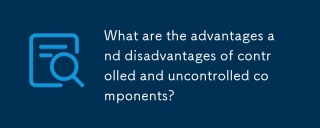
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
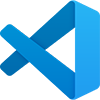
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
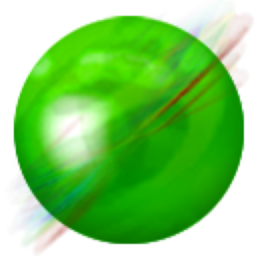
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
