


In PHP programming, using associative arrays is a common operation method. Associative arrays allow the use of strings as keys and can correspond to any type of value. However, sometimes we encounter a problem where we cannot use variables as keys for associative arrays. This problem seems simple, but in fact it can bring some strange results to the program. This article will explain in detail the cause of this problem and propose some solutions.
1. The phenomenon of the problem
In PHP, we can create an associative array in the following way:
$items = array( "apple" => 2.5, "orange" => 1.5, "banana" => 3.0 );
Suppose we need to obtain the relevant array based on the variables entered by the user value, then we will try to obtain this value through the following methods:
$item_name = "apple"; echo "The price of $item_name is ".$items[$item_name];
However, when we execute the above code, we will find that the program does not output the expected results, but displays the following error message:
Notice: Undefined index: apple in /path/to/script.php on line X
This error message tells us that there is no element with the key name "apple" in the $items array. But in fact we have defined this element in the array, why does this problem occur?
2. Cause of the problem
The reason for this problem is that when we use a variable as the key name of the array, PHP will use the value of this variable as the key name. That is, if the value of $item_name is "apple", then PHP will actually look for $items["apple"] as an array element. But if this element does not exist in the $items array, PHP will report a Notice level error.
We can further determine the cause of the problem by printing out the $items array. The following is the code to print out the $items array:
foreach ($items as $key => $value) { echo "$key: $value\n"; }
After executing the above code, we will see results similar to the following:
apple: 2.5 orange: 1.5 banana: 3.0
As you can see, the key names in the $items array are all It is of string type. But when we use a variable as the key name of the array, PHP will convert the value of the variable to a string type. In this way, if the value of the variable is not a valid string, the search will fail.
As a simple example, suppose we execute the following code:
$items[1] = 2.5; $item_name = 1; echo "The price of $item_name is ".$items[$item_name];
This code will also report a Notice-level error because the key name "1" does not exist in the $items array. "Elements. This is because PHP converts the value "1" of $item_name into the integer type 1, causing the search to fail.
3. Solution
In order to solve this problem, we need to ensure that when using a variable as the key name of an associative array, the value of the variable must be a valid string. Here are some solutions:
1. Use cast
We can use cast to convert the variable to string type. Specifically, you can use the following method:
$item_name = (string) $item_name;
This code will force the value of $item_name to a string type. This way, no matter what type the value of $item_name is, we can ensure that it is correctly converted to string type.
2. Use sprintf function
We can also use sprintf function to format strings. Specifically, you can use the following method:
$item_name = sprintf("%s", $item_name);
This code will force $item_name to a string. The first parameter of the sprintf function is a format string, where %s means forcing a variable to a string type.
3. Create a mapping array
We can create a mapping array to convert variables into valid associative array key names. For example:
$map = array( "apple" => "apple", "orange" => "orange", "banana" => "banana" ); $item_name = "apple"; $items[$map[$item_name]];
In this code, the $map array maps "apple" to "apple", "orange" to "orange", and "banana" to "banana". We use the $map array to convert the variable $item_name into a valid string. The expression $items[$map[$item_name]] will continue to work and will output the price as expected.
4. Summary
In PHP, associative arrays are a very powerful function. But when we use variables as keys of associative arrays, we need to pay attention to the type of the variables. If the variable type is incorrect, the associative array lookup will fail. We can solve this problem by using cast, sprintf function, or creating a mapping array. But no matter which method is used, the type of the variable should be ensured to ensure the normal operation of the program.
The above is the detailed content of What should I do if variables cannot be used in php associative arrays?. For more information, please follow other related articles on the PHP Chinese website!
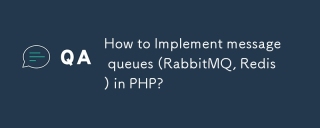
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
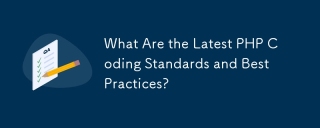
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
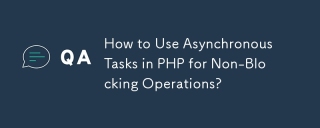
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
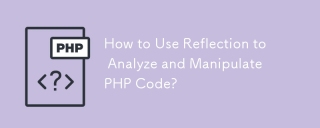
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
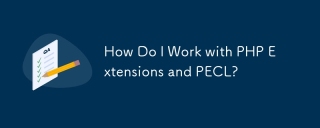
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
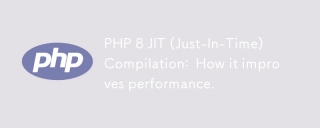
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
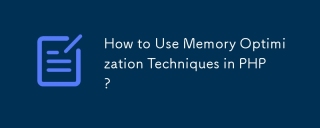
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
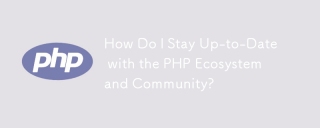
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
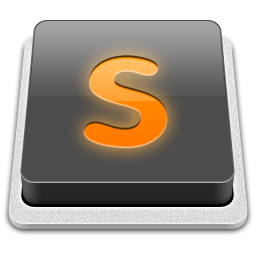
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment