In PHP programming, we often encounter situations where we need to delete empty two-dimensional arrays. In order to help everyone better understand this problem and how to solve it, this article will discuss in depth the methods and techniques of deleting empty two-dimensional arrays in PHP.
- Understanding two-dimensional arrays
Before we start discussing how to delete empty two-dimensional arrays, we must first understand what a two-dimensional array is. Simply put, a two-dimensional array is an array composed of multiple one-dimensional arrays. Each one-dimensional array represents a specific collection of data, and a two-dimensional array combines these one-dimensional arrays.
For example, the following is a two-dimensional array $employee, which contains three one-dimensional arrays. Each one-dimensional array stores information about an employee:
$employee = array( array('name' => 'Tom', 'age' => 25, 'salary' => 6000), array('name' => 'Jack', 'age' => 28, 'salary' => 8000), array('name' => 'Lucy', 'age' => 24, 'salary' => 5000) );
In this two-dimensional array , each one-dimensional array is an array containing three elements, where 'name', 'age' and 'salary' are key names, and 25, 28 and 24, 6000, 8000 and 5000 are key values. If we need to traverse this two-dimensional array and perform some operations, such as outputting the salary of each employee, it can be done in the following way:
foreach($employee as $emp) { echo "The salary of ".$emp['name']." is ".$emp['salary']."<br>"; }
The foreach statement here will traverse the entire $employee array and add each Assign a one-dimensional array to the variable $emp, and then output the salary of each employee in the loop body.
- Delete empty two-dimensional arrays
When dealing with complex two-dimensional arrays, we may encounter the situation of empty arrays, that is, one-dimensional arrays corresponding to certain elements. There are no key-value pairs in the array. This situation is not uncommon in data processing, but when we try to perform other operations on this two-dimensional array, the empty array may affect our calculation results and program efficiency. Therefore, the empty two-dimensional array needs to be deleted. The following introduces two methods of deleting empty two-dimensional arrays:
Method 1: Using the array_filter function
The array_filter function is one of the commonly used array functions in PHP. Its function is to filter the elements in the array. Elements, only retain elements that meet the specified rules. We can delete empty arrays in a two-dimensional array through the array_filter function. Specifically, an anonymous function can be used as the first parameter of array_filter. This function will be applied to each element in the array to determine whether to retain the element.
For example, the following is an example of using the array_filter function to delete an empty two-dimensional array:
$employee = array( array('name' => 'Tom', 'age' => 25, 'salary' => 6000), array('name' => '', 'age' => '', 'salary' => ''), array('name' => 'Lucy', 'age' => 24, 'salary' => 5000) ); $result = array_filter($employee, function($emp) { return count(array_filter($emp)) > 0; }); print_r($result);
In this example, we define a two-dimensional array named $employee, which contains three One-dimensional array. The second 1D array is empty and we need to delete it. When using the array_filter function, we pass an anonymous function as the first parameter. In this anonymous function, we execute the array_filter function on each one-dimensional array to remove empty elements. Then, we compare whether there are still elements in the one-dimensional array. If there are elements, return true, otherwise return false. Finally, the array_filter function will filter out the empty array based on the return value of this anonymous function and return a non-empty array.
Method 2: Use foreach loop
In addition to using the array_filter function, we can also delete empty arrays in the two-dimensional array through the foreach loop. Specifically, we can traverse the entire two-dimensional array and perform judgment on each one-dimensional array. If the number of elements in this one-dimensional array is 0, delete it.
For example, the following is an example of using a foreach loop to delete an empty two-dimensional array:
$employee = array( array('name' => 'Tom', 'age' => 25, 'salary' => 6000), array('name' => '', 'age' => '', 'salary' => ''), array('name' => 'Lucy', 'age' => 24, 'salary' => 5000) ); foreach($employee as $key => $emp) { if(count(array_filter($emp)) == 0) { unset($employee[$key]); } } print_r($employee);
In this example, we also define a two-dimensional array $employee, which contains three one-dimensional arrays. dimensional array. Use a foreach loop to traverse this two-dimensional array and perform judgment operations on each one-dimensional array. We use the array_filter function to filter out empty elements and obtain the number of filtered elements. If the number of elements is 0, it means that the one-dimensional array is empty and needs to be deleted. We use the unset function to delete it from the original array. Finally, we output the results.
- Summary
Using two-dimensional arrays in PHP is a very common operation. When dealing with these complex arrays, we sometimes need to delete empty arrays to avoid empty arrays. Negative impact on our calculations. In this article, we introduce two methods of deleting empty two-dimensional arrays in PHP, including using the array_filter function and using a foreach loop. Both methods have their own advantages and disadvantages. We can choose the method that suits us according to actual needs. Hope this article is helpful to everyone!
The above is the detailed content of How to delete empty two-dimensional array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
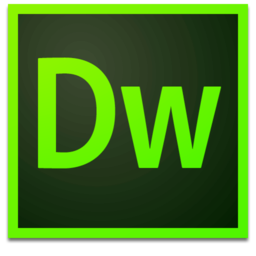
Dreamweaver Mac version
Visual web development tools
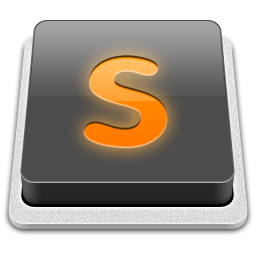
SublimeText3 Mac version
God-level code editing software (SublimeText3)
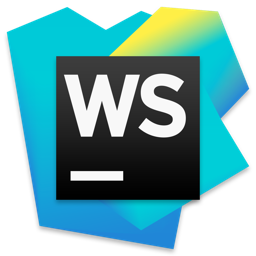
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
