PHP is a general-purpose, open source, server-side scripting language, suitable for Web development, especially suitable for Web development, and can be embedded in HTML. In PHP, array is a very commonly used data type. It can store multiple data items. These data items can be of any data type, including numbers, strings, and even objects.
In PHP, sometimes we need to perform some operations or obtain some information based on array elements. This article will explore how to perform various operations based on array elements.
1. Get the value of the array element
The method of getting the array element is very simple, just use the array subscript, as shown below:
$array = ['apple', 'banana', 'orange']; echo $array[1]; // 输出:banana
In the above code , we defined an array containing 3 elements, and then obtained the second element banana through subscript 1.
For associative arrays, you can also use subscripts to access:
$user = ['name' => '张三', 'age' => 18, 'gender' => '男']; echo $user['name']; // 输出:张三
In the above code, $user is an associative array, and we use the key name to get the value in the array.
2. Determine whether an array element exists
In PHP, we can use the in_array() function to determine whether an element exists in the array. The usage method is as follows:
$array = ['apple', 'banana', 'orange']; if (in_array('banana', $array)) { echo '存在'; } else { echo '不存在'; }
In the above code, we use the in_array() function to determine whether the banana element exists in the array. If it exists, the output exists, otherwise the output does not exist.
For associative arrays, we can use the array_key_exists() function to determine whether the specified key exists in the array, as follows:
$user = ['name' => '张三', 'age' => 18, 'gender' => '男']; if (array_key_exists('name', $user)) { echo '存在'; } else { echo '不存在'; }
In the above code, we use the array_key_exists() function to determine whether the specified key exists in the array. The key name exists, if it exists then the output exists, otherwise the output does not exist.
3. Replace the value of an array element
In PHP, we can use subscripts to replace the value of an element in the array. As shown below:
$array = ['apple', 'banana', 'orange']; $array[1] = 'pear'; var_dump($array); // 输出:array(3) { [0]=>string(5) "apple" [1]=>string(4) "pear" [2]=>string(6) "orange" }
In the above code, we replace the element banana with subscript 1 in the array with pear. This operation will change the value of the corresponding subscript in the array.
For associative arrays, you can also modify the value corresponding to the specified key through assignment, as follows:
$user = ['name' => '张三', 'age' => 18, 'gender' => '男']; $user['age'] = 19; var_dump($user); // 输出:array(3) { ["name"]=>string(6) "张三" ["age"]=>int(19) ["gender"]=>string(3) "男" }
In the above code, we modify the value of the key age in the array from 18 to 19.
4. Delete array elements
In PHP, we can use the unset() function to delete an element in the array. As shown below:
$array = ['apple', 'banana', 'orange']; unset($array[1]); var_dump($array); // 输出:array(2) { [0]=>string(5) "apple" [2]=>string(6) "orange" }
In the above code, we use the unset() function to delete the element banana with subscript 1 in the array.
For associative arrays, you can also use the unset() function to delete the value corresponding to the specified key, as follows:
$user = ['name' => '张三', 'age' => 18, 'gender' => '男']; unset($user['age']); var_dump($user); // 输出:array(2) { ["name"]=>string(6) "张三" ["gender"]=>string(3) "男" }
In the above code, we use the unset() function to delete the key in the array is the value of age.
Summary
Array is a very commonly used data type in PHP. Mastering how to perform various operations based on array elements is one of the basic skills of PHP developers. This article introduces how to access array elements based on subscripts, determine whether an array element exists, replace the value of an array element, and delete an array element. I hope readers can benefit from it.
The above is the detailed content of How to perform various operations based on array elements in php. For more information, please follow other related articles on the PHP Chinese website!
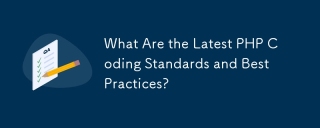
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
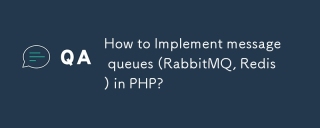
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
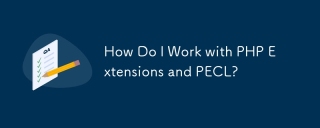
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
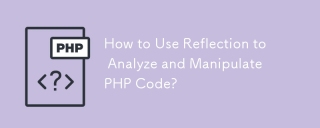
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
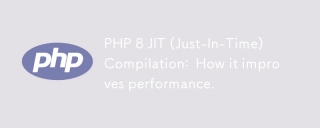
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
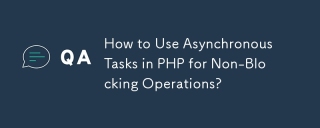
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
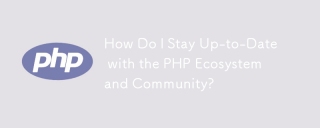
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
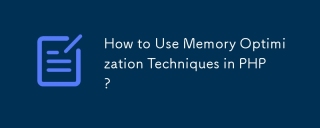
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
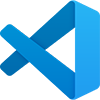
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
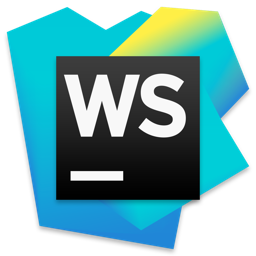
WebStorm Mac version
Useful JavaScript development tools
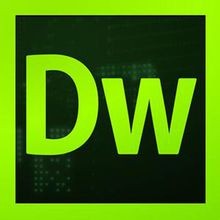
Dreamweaver CS6
Visual web development tools
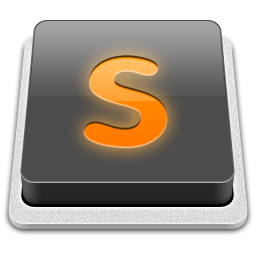
SublimeText3 Mac version
God-level code editing software (SublimeText3)
