


During the development process, we may encounter situations where JSON data is stored in the database. This usually does not present a problem when dealing with English strings, but when we involve Chinese characters, we may encounter garbled characters. This article will introduce how to solve the JSON garbled problem that occurs when storing Chinese characters into a MySQL database.
- Database character set
First, we need to confirm whether the database character set is correct. The default character set of MySQL is Latin1, which does not support Chinese character sets. If your encoding is not UTF-8, you will need to change MySQL's character set. In the MySQL command line, enter the following command to change the character set:
SET NAMES 'utf8';
Then execute the following command:
ALTER DATABASE `your_database` CHARACTER SET utf8 COLLATE utf8_general_ci;
- Encoding issues
Another FAQ It's an encoding issue. Your text editor may use a different encoding than the server, or may use a different encoding than the MySQL database. To avoid garbled characters, make sure all files use the same encoding. For php files, we recommend using UTF-8 encoding.
- Storing JSON data into the database
During the development process, the best way to store JSON data is to convert the data into a JSON string through PHP's json_encode function , and then insert it into the database table. For Chinese characters, UTF-8 encoding format is required.
The following is a sample code to store JSON data into a MySQL database:
//连接数据库 $conn = mysqli_connect($servername, $username, $password, $dbname); //设置字符集 mysqli_set_charset($conn, "utf8"); //将 JSON 数据转换为字符串 $json_data = json_encode($data, JSON_UNESCAPED_UNICODE); //插入数据到数据库表中 $sql = "INSERT INTO `table` (`id`, `data`) VALUES (NULL, '$json_data')"; $result = mysqli_query($conn, $sql); //关闭数据库连接 mysqli_close($conn);
- Reading JSON data
When you want to read the data stored in the database When working with JSON data in a JSON string, you can use PHP's json_decode function to convert the JSON string into a PHP array or object. Note that when using json_decode, the second parameter needs to be set to true to ensure that the data is returned as an array rather than an object.
The following is a sample code for reading JSON data from a MySQL database:
//连接数据库 $conn = mysqli_connect($servername, $username, $password, $dbname); //设置字符集 mysqli_set_charset($conn, "utf8"); //查询数据库 $sql = "SELECT `data` FROM `table` WHERE id = '1'"; $result = mysqli_query($conn, $sql); $row = mysqli_fetch_assoc($result); //将 JSON 字符串转换为 PHP 数组 $json_data = json_decode($row['data'], true); //关闭数据库连接 mysqli_close($conn);
Summary:
When storing JSON data, ensure that the database character set is UTF-8. The text editor uses the correct encoding format and uses PHP's json_encode function to convert the data to a JSON string. When reading JSON data, use PHP's json_decode function to convert the JSON string into a PHP array.
The above are some tips to solve the JSON garbled problem that occurs when storing Chinese characters into the MySQL database. We hope these tips will be helpful to you.
The above is the detailed content of What to do if php json saves Chinese garbled characters in the database. For more information, please follow other related articles on the PHP Chinese website!
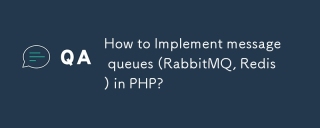
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
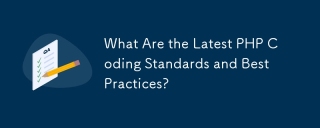
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
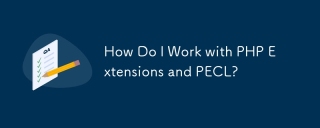
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
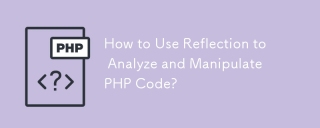
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
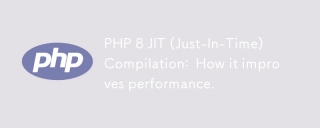
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
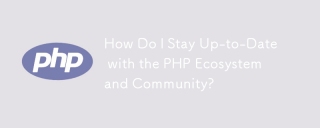
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
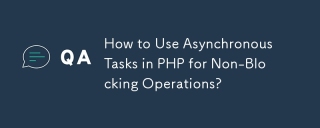
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
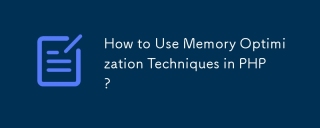
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
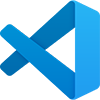
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
