During the PHP development process, it is often necessary to splice arrays. This can be achieved using the array_merge() function or the " " operator. The use of these two methods will be introduced in detail below.
1. Use the array_merge() function
array_merge() function is used to merge two or more arrays into one array and return it. It will append the subsequent array contents to the front in sequence. array and use the new array as the return value. The syntax of this function is as follows:
array array_merge ( array $array1 [, array $... ] )
Among them, the $array1 parameter is a required parameter and represents the first array. The $... parameter represents an optional parameter, which is the name of other arrays to be merged.
The following is an example of using the array_merge() function to splice arrays:
// 定义两个数组 $arr1 = array('a', 'b', 'c'); $arr2 = array(1, 2, 3); // 使用 array_merge() 函数将两个数组合并成一个新的数组 $result = array_merge($arr1, $arr2); print_r($result);
The output result of the above code is:
Array
(
[0] => a [1] => b [2] => c [3] => 1 [4] => 2 [5] => 3
)
As you can see, two arrays can be completely merged together using the array_merge() function.
2. Use the " " operator
The " " operator can also be used for array splicing. Its function is to merge two arrays into a new array and retain the contents of the original array. The key name and the corresponding value. If there are the same key names, the value of the subsequent array will overwrite the value of the previous array.
The following is an example of using the " " operator to splice arrays:
// 定义两个数组 $arr1 = array('a' => 1, 'b' => 2, 'c' => 3); $arr2 = array('d' => 4, 'e' => 5, 'a' => 6); // 使用“+”操作符将两个数组合并成一个新的数组 $result = $arr1 + $arr2; print_r($result);
The output result of the above code is:
Array
(
[a] => 1 [b] => 2 [c] => 3 [d] => 4 [e] => 5
)
You can see that using the " " operator will merge the arrays together and retain the key names and corresponding values in the original array. If there are the same key names, the values of the subsequent arrays will be overwritten. The value of the previous array.
3. Use the array_merge() function in combination with the " " operator
If you want to retain the key names and corresponding values in the original array at the same time, and merge the arrays together completely, you can Use the array_merge() function with the " " operator.
The following is an example of using the array_merge() function and the " " operator to splice arrays:
// 定义两个数组 $arr1 = array('a' => 1, 'b' => 2, 'c' => 3); $arr2 = array('d' => 4, 'e' => 5, 'a' => 6); // 结合使用 array_merge() 函数和“+”操作符将两个数组合并成一个新的数组 $result = $arr2 + $arr1; print_r($result);
The output result of the above code is:
Array
(
[d] => 4 [e] => 5 [a] => 1 [b] => 2 [c] => 3
)
As you can see, using the array_merge() function in combination with the " " operator can completely merge the arrays together and retain the key names and corresponding values in the original array. .
To sum up, you can use the array_merge() function or the " " operator to splice arrays in PHP, and use different methods according to different needs.
The above is the detailed content of How to splice arrays in php (two methods). For more information, please follow other related articles on the PHP Chinese website!
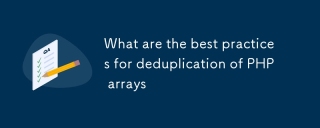
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
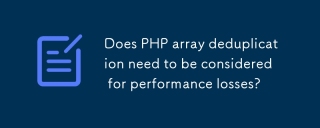
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
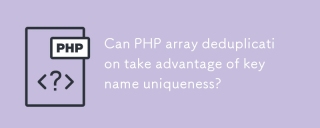
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
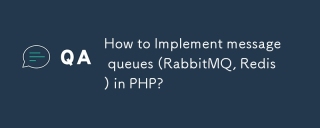
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
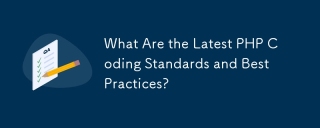
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
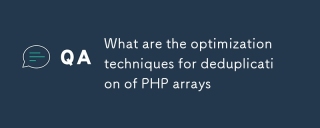
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
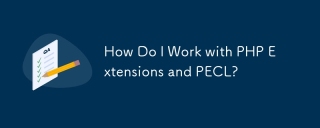
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
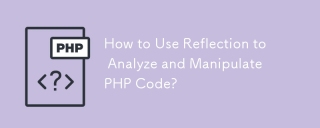
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
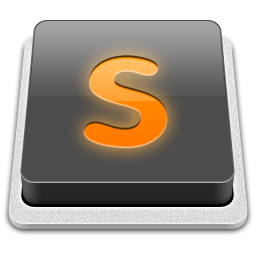
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment
