Sometimes we need to determine whether a string exists in an array. This situation is very common in PHP. In this article, we'll demonstrate a few different ways of querying whether a character is present in an array.
Method 1: Use the in_array() function
In PHP, we can use the in_array() function to check whether a value is in an array. The syntax of this function is as follows:
bool in_array(mixed $needle, array $haystack [, bool $strict = FALSE])
The parameter $needle is the value to be found, and the parameter $haystack is The array in which to find the value. Parameter $strict is optional and defaults to FALSE. If TRUE, use strict mode to compare values.
Sample code:
//Define array
$fruits = array('apple', 'banana', 'orange', 'grape');
// Find whether the value is in the array
if (in_array('apple', $fruits)) {
echo 'apple 在数组中';
} else {
echo 'apple 不在数组中';
}
Output result :
apple in the array
Method 2: Use array_search() function
Another way to find whether a value in an array exists is to use the array_search() function. This function returns the key name of the found value, or FALSE if not found.
Sample code:
//Define array
$fruits = array('apple', 'banana', 'orange', 'grape');
// Find value
$key = array_search('orange', $fruits);
if ($key !== false) {
echo 'orange 在数组中,键为:' . $key;
} else {
echo 'orange 不在数组中';
}
Output result:
orange In the array, the key is: 2
Method 3: Use in_array() and array_search() functions in combination
Sometimes we need to use both the in_array() and array_search() functions to query the values in the array, because they can handle different situations. If you want to check if a value is in an array, you can use the in_array() function, and if you need to get the key name, you can use the array_search() function.
Sample code:
//Define array
$fruits = array('apple', 'banana', 'orange', 'grape');
// Check if the value is in the array
if (in_array('banana', $fruits)) {
// 获取键名 $key = array_search('banana', $fruits); echo 'banana 在数组中,键为:' . $key;
} else {
echo 'banana 不在数组中';
}
Output result :
banana In the array, the key is: 1
Method 4: Use foreach to loop through the array
In PHP, we can also use foreach to loop through the array to determine Whether the value is in the array. This method is less concise than the in_array() and array_search() functions, but in some cases it can be easier to understand.
Sample code:
//Define array
$fruits = array('apple', 'banana', 'orange', 'grape');
// Traverse the array
foreach ($fruits as $key => $value) {
if ($value == 'orange') { echo 'orange 在数组中,键为:' . $key; break; }
}
Output result:
orange In the array, key For: 2
Conclusion
No matter which method you use, querying whether a character exists in an array is very easy. In any case, we can use in_array() or array_search() function to check if a value is in an array. If you need to get the key name, you should use the array_search() function. Using a foreach loop to iterate over an array is also a good option if you need a more flexible control flow.
The above is the detailed content of How to check if a character exists in an array in php. For more information, please follow other related articles on the PHP Chinese website!
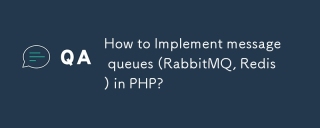
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
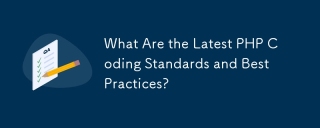
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
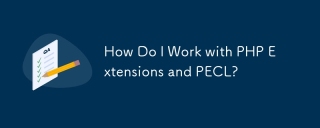
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
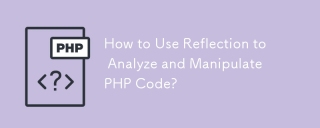
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
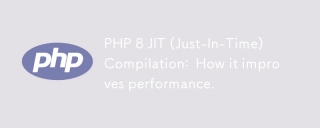
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
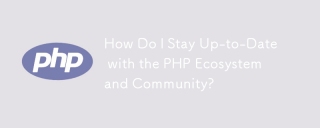
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
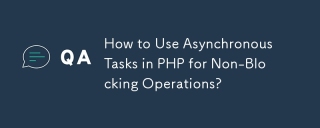
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
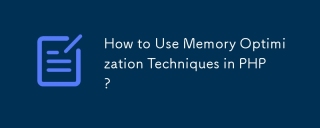
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
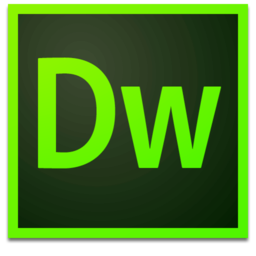
Dreamweaver Mac version
Visual web development tools
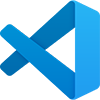
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
