The origin of double check lock
First let’s take a look at the non-thread-safe initialization singleton mode
public class UnsafeLazyInitialization { private static UnsafeLazyInitialization instance; public static UnsafeLazyInitialization getInstance(){ if(instance == null){ //1: 线程A执行 instance = new UnsafeLazyInitialization(); //2: 线程B执行 } return instance; } }
In the UnsafeLazyInitialization class, assume that when thread A executes code 1, thread B When code 2 is executed, thread A may see that the instance reference object has not yet been initialized.
For the UnsafeLazyInitialization class, we can synchronize the getInstance() method to achieve thread-safe delayed initialization. The sample code is as follows:
public static synchronized UnsafeLazyInitialization getInstance(){ if(instance == null){ //1: 线程A执行 instance = new UnsafeLazyInitialization(); //2: 线程B执行 } return instance; } }
Because the above code does the getInstance() method Without synchronization processing, this may lead to increased synchronization program overhead. If getInstance() is frequently called by multiple threads, the program execution performance will be reduced. On the contrary, if it is not called by multiple threads, the delayed initialization method of the getInstance() method will affect performance.
Before JVM 1.6, synchronized was a heavyweight lock, so it was very performance-consuming, so people thought of a double-check locking (Dobule-check Locking) solution to improve performance. The sample code is as follows:
public class DoubleCheckedLocking { //1、 private static Instance instance; //2、 public static Instance getInstance(){ //3、 if(instance == null){ //4、第一次检查 synchronized (DoubleCheckedLocking.class){ //5、枷锁 if(instance == null){ //6、第二次检查 instance = new Instance(); //7、问题的根源在这里 } //8、 } } return instance; } }
As shown in the above code: If the first check instance in step 4 is not null, there is no need to perform the following locking operation, which greatly reduces the performance problems caused by synchronized locks. There seems to be no problem with the above code. 1. When multiple thread views create new objects, the synchronized keyword can be used to ensure that only one thread successfully creates the object.
2. If the instance object has been created, obtain the object instance directly through the getInstatnce() method.
Double verification lock problem above
The above code looks perfect, but when step 4 is executed, instatnce! =null, the reference object of instatnce may not be initialized yet.
The root of the problem
When we execute the above code to step 7, instance = new Instance();, an object is created. The steps to create an object can be divided into three steps, as follows :
memory = allocate() //1.分配内存空间memory ctorInstance(memory) //2, 初始化对象在内存 分配内存空间memory上初始化 Singleton 对象 instance = memory //3、设置 instance 指向刚分配的内存地址memory
The above three lines of code 2 and 3 may be reordered. (On the JTI compiler, this reordering really happens) The execution sequence after the reordering of steps 2 and 3
memory = allocate() //1.分配内存空间memory instance = memory //3、设置 instance 指向刚分配的内存地址memory // 注意此时instance对象还没有被初始化,但是instance的引用已经不是null了。 ctorInstance(memory) //2, 初始化对象在内存 分配内存空间memory上初始化 Singleton 对象
Let’s take a look at the multi-thread execution sequence
public class DoubleCheckedLocking { //1、 private static volatile Instance instance; //2、 public static Instance getInstance(){ //3、 if(instance == null){ //4、第一次检查 synchronized (DoubleCheckedLocking.class){ //5、枷锁 if(instance == null){ //6、第二次检查 instance = new Instance(); //7、问题的根源在这里 } //8、 } } return instance; } }
The above is the detailed content of How to solve the double check lock problem in java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
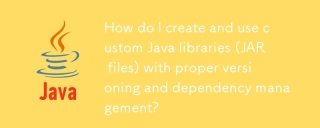
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
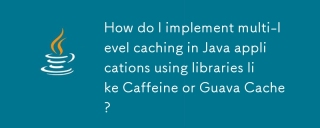
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
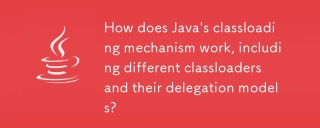
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
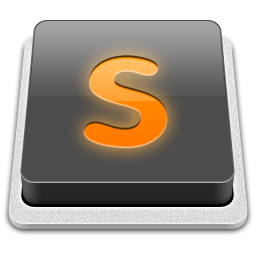
SublimeText3 Mac version
God-level code editing software (SublimeText3)