With the continuous development of JavaScript, Node.js plays an important role, allowing us to use JavaScript on the server side. Node.js provides powerful features and flexible solutions, making it the preferred platform for many developers to build web applications.
However, sometimes Node.js applications need to use some new features introduced in the ECMAScript 6 specification (i.e. ECMAScript 2015), such as Promise, arrow functions, let and const functions, etc. These new features can greatly simplify your code and make it more readable. In order to support these new features, the "Harmony" version of Node.js needs to be used.
Below we will detail how to enable these features by installing the "Harmony" version of Node.js.
What is the "Harmony" version of Node.js?
The "Harmony" version of Node.js is an implementation of the ECMAScript 6 specification. Node.js divides ES6 support into two parts: the first covers all completed specifications, and the second covers specifications that are not yet complete, and these specifications are called "Harmony" specifications.
In fact, "Harmony" is a name used by internal developers to refer to the ES6 specification. It provides some new features recently added to the ES6 specification, such as arrow functions, Promises, etc.
Enable the "Harmony" version
To enable the "Harmony" version, you need to start Node.js with a few different command line options. Specifically, the "--harmony" flag needs to be added to the command that launches the script. For example:
node --harmony index.js
You can add the following startup script configuration in the "package.json" file:
"scripts": { "start": "node --harmony index.js" }
Then you can use the following command to start the application:
npm start
Harmony Functional Examples
Next, we will introduce several functional examples using the "Harmony" version of Node.js.
1. Use the let keyword
The let keyword can replace var and is used to declare variables and set their initial values. It introduces block-level scoping, making variables clearer. Look at the following example:
for(let i=0; i<p>Since the scope of the let keyword is limited to the code block, when the control flow leaves the loop, the variable "i" will no longer be available. </p><p>2. Use arrow functions</p><p>Arrow functions are another important feature in ES6. They provide a more concise way to declare anonymous functions and bind this to the parent scope. For example: </p><pre class="brush:php;toolbar:false">const myFunction = arg => arg.toLowerCase(); console.log(myFunction("HELLO")); // 输出"hello"
In this example, "arg" is an argument, and the arrow function converts it to lowercase and returns the processed result. Since arrow functions do not have their own this value, they are often used in callback functions to avoid this binding errors.
3. Use Promise
Promise is a new method of handling asynchronous operations introduced in ES6. They provide a more elegant and powerful programming model that avoids "callback hell". For example:
const fetchData = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve("data"); }, 500); }); }; fetchData() .then(data => console.log(data)) .catch(err => console.log(err));
In this example, the Promise object represents an asynchronous operation and can resolve to a result when the operation completes. This approach is much simpler than traditional callback functions because it can chain multiple asynchronous operations and keep the flow clear in the code.
Summary
Using the "Harmony" version is an important way to enable ES6 specifications in Node.js. It allows developers to use many new features and take advantage of its server-side JavaScript platform to build more elegant, efficient and reliable web applications. Therefore, in order to fully master Node.js, we must learn to use the ECMAScript 6 specification and make good use of the new features it provides.
The above is the detailed content of How to add harmony to nodejs. For more information, please follow other related articles on the PHP Chinese website!

No,youshouldn'tusemultipleIDsinthesameDOM.1)IDsmustbeuniqueperHTMLspecification,andusingduplicatescancauseinconsistentbrowserbehavior.2)Useclassesforstylingmultipleelements,attributeselectorsfortargetingbyattributes,anddescendantselectorsforstructure

HTML5aimstoenhancewebcapabilities,makingitmoredynamic,interactive,andaccessible.1)Itsupportsmultimediaelementslikeand,eliminatingtheneedforplugins.2)Semanticelementsimproveaccessibilityandcodereadability.3)Featureslikeenablepowerful,responsivewebappl

HTML5aimstoenhancewebdevelopmentanduserexperiencethroughsemanticstructure,multimediaintegration,andperformanceimprovements.1)Semanticelementslike,,,andimprovereadabilityandaccessibility.2)andtagsallowseamlessmultimediaembeddingwithoutplugins.3)Featur

HTML5isnotinherentlyinsecure,butitsfeaturescanleadtosecurityrisksifmisusedorimproperlyimplemented.1)Usethesandboxattributeiniframestocontrolembeddedcontentandpreventvulnerabilitieslikeclickjacking.2)AvoidstoringsensitivedatainWebStorageduetoitsaccess

HTML5aimedtoenhancewebdevelopmentbyintroducingsemanticelements,nativemultimediasupport,improvedformelements,andofflinecapabilities,contrastingwiththelimitationsofHTML4andXHTML.1)Itintroducedsemantictagslike,,,improvingstructureandSEO.2)Nativeaudioand

Using ID selectors is not inherently bad in CSS, but should be used with caution. 1) ID selector is suitable for unique elements or JavaScript hooks. 2) For general styles, class selectors should be used as they are more flexible and maintainable. By balancing the use of ID and class, a more robust and efficient CSS architecture can be implemented.

HTML5'sgoalsin2024focusonrefinementandoptimization,notnewfeatures.1)Enhanceperformanceandefficiencythroughoptimizedrendering.2)Improveaccessibilitywithrefinedattributesandelements.3)Addresssecurityconcerns,particularlyXSS,withwiderCSPadoption.4)Ensur

HTML5aimedtoimprovewebdevelopmentinfourkeyareas:1)Multimediasupport,2)Semanticstructure,3)Formcapabilities,and4)Offlineandstorageoptions.1)HTML5introducedandelements,simplifyingmediaembeddingandenhancinguserexperience.2)Newsemanticelementslikeandimpr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment
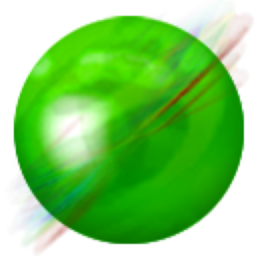
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
