


With the rapid development of front-end technology, web development has also become complex and changeable. Especially when we need to request data from different domain names, we will encounter cross-domain problems. This article will introduce how to use Node.js to receive cross-domain requests sent through the POST method.
First of all, cross-domain problems are caused by the browser’s same-origin policy. The same-origin policy means that scripts with different domain names, different protocols, and different ports cannot obtain data from each other. This means that if our page needs to obtain data from other domain names, an error will be reported. In order to solve this problem, we need to use some means to bypass the same origin policy.
One way to solve the cross-domain problem is to use CORS (Cross-Origin Resource Sharing) technology. CORS allows us to explicitly specify in the response which domain names can access our resources through Ajax. However, if our API server does not implement CORS, or we cannot modify the configuration on the server, we need to try other methods to solve the problem.
A common method is to use JSONP technology. JSONP dynamically creates a script tag in the page, and then requests cross-domain data through the tag. The src attribute of this tag points to a JavaScript file on the API server that returns JSON data. JSONP solves the cross-domain problem, but it can only send GET requests, not POST requests.
Therefore, we need another way to implement cross-domain POST requests. The following is an example of using Node.js to implement a cross-domain POST request:
First, we need to use the http module of Node.js to create a web server and listen for POST requests from the client:
const http = require('http'); const server = http.createServer((req, res) => { if (req.method === 'POST') { let body = ''; req.on('data', data => { body += data; }); req.on('end', () => { console.log(body); res.end(); }); } }); server.listen(8080);
This simple web server will listen to POST requests from the client on port 8080, and output the request body to the console.
Next, we need to use the XMLHttpRequest object on the client to send the POST request. However, due to cross-domain issues, we cannot directly send the request to the API server. Therefore, we first need to create a proxy server on the client side, and then let the proxy server forward the request.
The code of the proxy server is as follows:
const http = require('http'); const clientReq = http.request({ method: 'POST', hostname: 'yourapi.com', path: '/path/to/api', headers: { 'Content-Type': 'application/json' } }, (res) => { res.on('data', (data) => { /* do something */ }); }); clientReq.on('error', (error) => { /* handle error */ }); process.stdin.on('data', (chunk) => { clientReq.write(chunk); }); process.stdin.on('end', () => { clientReq.end(); });
This proxy server will forward the request read from the standard input to the API server.
Finally, we need to implement cross-domain POST requests by starting the proxy server on the client and then sending the POST request to the proxy server. The sample code is as follows:
const http = require('http'); const querystring = require('querystring'); const postData = querystring.stringify({ 'msg': 'Hello World!' }); const options = { hostname: 'localhost', port: 8080, path: '/proxy', method: 'POST', headers: { 'Content-Type': 'application/x-www-form-urlencoded', 'Content-Length': Buffer.byteLength(postData) } }; const req = http.request(options, (res) => { res.setEncoding('utf8'); res.on('data', (chunk) => { console.log(`BODY: ${chunk}`); }); res.on('end', () => { console.log('No more data in response.') }) }); req.on('error', (e) => { console.error(`problem with request: ${e.message}`); }); // 请求的数据 req.write(postData); req.end();
This code snippet will send a POST request to the proxy server, and the proxy server will forward the request to the API server. The response returned by the API server will be forwarded back to the client by the proxy server.
Summary: Cross-domain issues are an important issue in web development and require us to take some technical means to solve them. In this article we introduce how to use Node.js to receive cross-domain POST requests and use a proxy server to bypass the same-origin policy. Hope this article is helpful to you.
The above is the detailed content of How to receive cross-origin requests sent via POST method with Node.js. For more information, please follow other related articles on the PHP Chinese website!

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
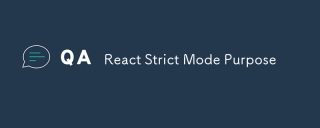
React Strict Mode is a development tool that highlights potential issues in React applications by activating additional checks and warnings. It helps identify legacy code, unsafe lifecycles, and side effects, encouraging modern React practices.
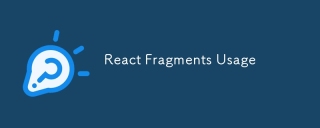
React Fragments allow grouping children without extra DOM nodes, enhancing structure, performance, and accessibility. They support keys for efficient list rendering.
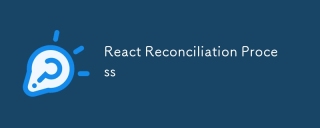
The article discusses React's reconciliation process, detailing how it efficiently updates the DOM. Key steps include triggering reconciliation, creating a Virtual DOM, using a diffing algorithm, and applying minimal DOM updates. It also covers perfo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
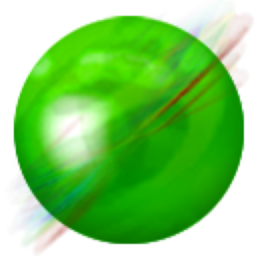
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
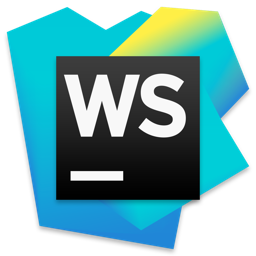
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.