How to use Java exception handling UncaughtExceptionHandler
Exception handling
Thread uncaught exception UncaughtException requires UncaughtZExceptionHandler for processing
So why do you have to use UncaughtZExceptionHandler?
The main thread can easily capture the thread, but the sub-thread cannot
As can be seen from the following code, even if the sub-thread throws an exception, the main thread will not Unaffected
public class ChildException implements Runnable{ public static void main(String[] args) { new Thread(new ChildException()).start(); for (int i = 0; i < 10; i++) { System.out.println(i); } } @Override public void run() { throw new RuntimeException(); } } /* * Exception in thread "Thread-0" java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.ChildException.run(ChildException.java:14) at java.lang.Thread.run(Thread.java:748) 0 1 2 3 4 5 6 7 8 9 * */
As can be seen from the code below, even if you want to use catch to capture child thread exceptions, it is useless
try catch is for the main thread, there is no way to catch the exception of the sub-thread
public class CantCatch implements Runnable { public static void main(String[] args) throws InterruptedException { try { new Thread(new CantCatch(), "thread0").start(); Thread.sleep(300); new Thread(new CantCatch(), "thread1").start(); Thread.sleep(300); new Thread(new CantCatch(), "thread2").start(); Thread.sleep(300); new Thread(new CantCatch(), "thread3").start(); Thread.sleep(300); } catch (RuntimeException e) { System.out.println("catch"); } } @Override public void run() { throw new RuntimeException(); } } /* * Exception in thread "thread0" java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.CantCatch.run(CantCatch.java:22) at java.lang.Thread.run(Thread.java:748) Exception in thread "thread1" java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.CantCatch.run(CantCatch.java:22) at java.lang.Thread.run(Thread.java:748) Exception in thread "thread2" java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.CantCatch.run(CantCatch.java:22) at java.lang.Thread.run(Thread.java:748) Exception in thread "thread3" java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.CantCatch.run(CantCatch.java:22) at java.lang.Thread.run(Thread.java:748) Process finished with exit code 0 * */
Exceptions can be caught by try catch in the run method, but it is particularly troublesome because it needs to be done manually Try catch in each run method
UncaughtExceptionHandler
Custom UncaughtExceptionHandler
public class MyUncaughtHandler implements Thread.UncaughtExceptionHandler{ private String name; public MyUncaughtHandler(String name) { this.name = name; } @Override public void uncaughtException(Thread t, Throwable e) { Logger logger = Logger.getAnonymousLogger(); logger.log(Level.WARNING, "线程异常" + t.getName(), e); System.out.println(name + "捕获" + t.getName()+ e); } }
Use a custom class to catch exceptions
public class UseOwnExceptionHandler implements Runnable { public static void main(String[] args) throws InterruptedException { Thread.setDefaultUncaughtExceptionHandler(new MyUncaughtHandler("MyHandler")); // try { new Thread(new UseOwnExceptionHandler(), "thread0").start(); Thread.sleep(300); new Thread(new UseOwnExceptionHandler(), "thread1").start(); Thread.sleep(300); new Thread(new UseOwnExceptionHandler(), "thread2").start(); Thread.sleep(300); new Thread(new UseOwnExceptionHandler(), "thread3").start(); Thread.sleep(300); // } catch (RuntimeException e) { // System.out.println("catch"); // } } @Override public void run() { // try { throw new RuntimeException(); // } catch (RuntimeException e) { // System.out.println("e"); // } } } /* * 一月 29, 2023 11:22:01 上午 com.jx.JavaTest.Thread.Exception.MyUncaughtHandler uncaughtException 警告: 线程异常thread0 java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.UseOwnExceptionHandler.run(UseOwnExceptionHandler.java:24) at java.lang.Thread.run(Thread.java:748) MyHandler捕获thread0java.lang.RuntimeException 一月 29, 2023 11:22:01 上午 com.jx.JavaTest.Thread.Exception.MyUncaughtHandler uncaughtException 警告: 线程异常thread1 java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.UseOwnExceptionHandler.run(UseOwnExceptionHandler.java:24) at java.lang.Thread.run(Thread.java:748) MyHandler捕获thread1java.lang.RuntimeException 一月 29, 2023 11:22:02 上午 com.jx.JavaTest.Thread.Exception.MyUncaughtHandler uncaughtException 警告: 线程异常thread2 java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.UseOwnExceptionHandler.run(UseOwnExceptionHandler.java:24) at java.lang.Thread.run(Thread.java:748) MyHandler捕获thread2java.lang.RuntimeException 一月 29, 2023 11:22:02 上午 com.jx.JavaTest.Thread.Exception.MyUncaughtHandler uncaughtException 警告: 线程异常thread3 java.lang.RuntimeException at com.jx.JavaTest.Thread.Exception.UseOwnExceptionHandler.run(UseOwnExceptionHandler.java:24) at java.lang.Thread.run(Thread.java:748) MyHandler捕获thread3java.lang.RuntimeException Process finished with exit code 0 * */
The above is the detailed content of How to use Java exception handling UncaughtExceptionHandler. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
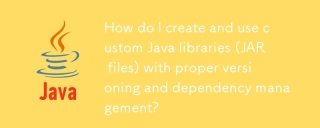
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
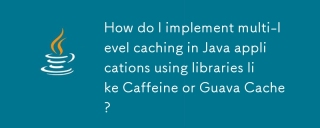
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
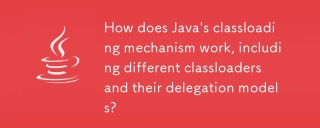
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
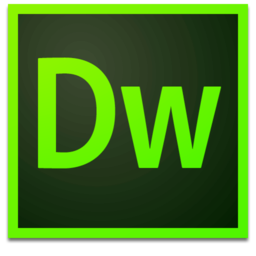
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.