Golang is a very popular programming language. Its powerful features include automatic garbage collection, concurrency, etc., making it a popular choice. During the development process, error handling is indispensable. This article will detail how to catch errors in Golang.
1. Brief description of errors
The error type is used in Golang to represent the return value of a function to indicate the error situation during the function call. If an error occurs during function execution, a non-nil error value is returned. If the function executes successfully, a nil value is returned.
A basic example:
func Divide(a int, b int) (int, error) { if b == 0 { return 0, errors.New("b cannot be zero") } return a / b, nil } func main() { result, err := Divide(6, 3) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Result:", result) } }
In the above example, the return value of the Divide function is two parameters-an integer and a value of the wrong type. If b is 0, the Divide function returns 0 and an error message "b cannot be zero". In the main function, multiple assignment is used to accept the return value of the Divide function. If err is not equal to nil, it means that an error occurred in the execution of the Divide function. Otherwise, the Divide function is executed successfully.
2. Error handling
In the process of Golang development, error handling is an essential part. Errors may come from files, networks, operating systems, hardware, etc. If these errors cannot be handled properly, they may affect the stability and correctness of the program.
- Error type
In Golang, the error type is an interface type and has only one method, Error() string, for returning error information. Normally, we use the errors package to create an error object:
import "errors" func SomeFunc() error { return errors.New("Some error occurred!") }
When an exception occurs in the program, an error type value is usually returned. Developers should use the information in the error object to check problems and handle errors.
- Error handling
In Golang, error handling can be implemented using if statements, as shown below:
result, err := SomeFunc() if err != nil { // 处理err } else { // 处理result }
During the checking process, we You should first determine whether err is nil. If err is not nil, it means there is an error in the current function, and we need to handle the error.
During the processing process, some logs can be output, or returned directly to the caller. If there is a problem with the program, the problem should be reported directly to the user.
- Error message
Use Golang’s errors package to create a customized error message:
import "fmt" type MyError struct { Line int Message string } func (e *MyError) Error() string { return fmt.Sprintf("%d:%s", e.Line, e.Message) } func SomeFunc() error { return &MyError{Line: 42, Message: "Something went wrong!"} }
In the above code, a MyError is customized Type, this type has two attributes - Line (indicating the number of lines where the error occurred) and Message (specific error message). This type also implements the Error() method for returning error information. In SomeFunc, a value of type MyError is returned.
In the process of writing code, we should try to avoid simply returning error information, but provide some useful diagnostic information to help developers quickly locate problems.
3. Error Capture
In Golang, there are many ways to capture errors:
- panic and recovery mechanisms
In Golang, the panic function is used to cause runtime errors, such as array subscript out of bounds, division by 0, etc. Once the program executes the panic function, the program will stop execution and start backtracing the caller until the top-level function. This function can use the recover function to capture the panic during the backtracing process, and then handle it accordingly.
Simple example:
func main() { defer func() { if err := recover(); err != nil { fmt.Println(err) } }() panic("An error occurred!") }
In the above example, use the defer keyword to handle panic. After a panic occurs, execute the defer statement. If the recover function is called in the defer statement, the recover function will return the parameters passed in by the panic function and restore the execution flow of the program to normal execution, otherwise the program will exit.
When using panic and recover, you need to follow some guidelines:
- recover function can only be used in defer statement;
- recover function can only be used in defer statement It is only valid when called within the function;
- After a panic occurs, during the call stack backtrace, if no recover instance is found that can handle the panic, the program will exit.
- defer mechanism
#The defer mechanism can be used to clean up functions, such as closing files, releasing memory, unlocking resources, etc. No matter where the function ends (either normally or terminated by panic after causing an error), the defer statement will be executed, so the use of the defer mechanism can ensure that the function cleanup is executed correctly.
Simple example:
func SomeFunc() error { f, err := os.OpenFile("test.txt", os.O_RDONLY, 0644) if err != nil { return err } defer f.Close() // 相关代码... }
In the above example, the defer keyword is used to postpone the Close operation of the file handle until the end of the function for processing.
4. Summary
Error handling is an integral part of the Golang programming process. When writing code, you should pay attention to using the error type to return error information, provide useful diagnostic information, use if statements for error handling, etc. At the same time, you can use the panic and recover mechanisms to capture errors and the defer mechanism for cleanup, thereby improving the stability and correctness of the program and providing users with a better experience.
The above is the detailed content of Details on how to catch errors in Golang. For more information, please follow other related articles on the PHP Chinese website!
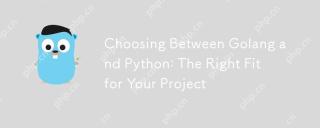
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
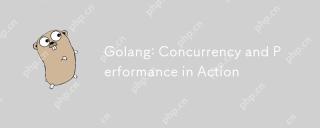
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
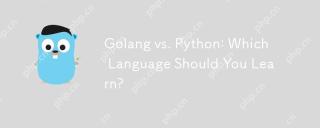
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
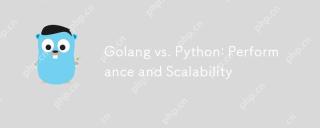
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
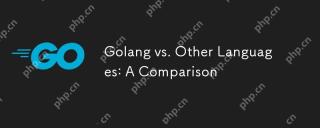
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
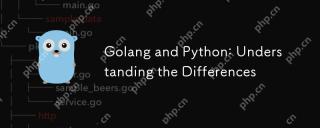
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
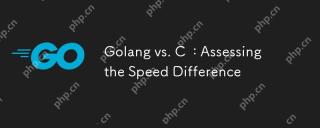
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
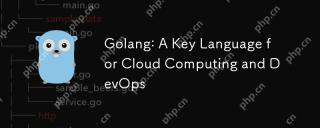
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
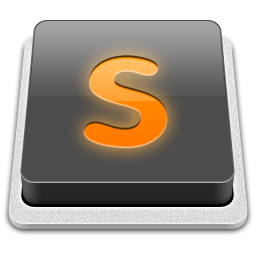
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.