In Vue, it is a common requirement to check whether the DOM has a certain class name. Two methods are introduced below to implement this function.
Method 1: Using $refs
In Vue, you can use the $refs
attribute to get a reference to an element in the component. You can then use the native JavaScript method classList.includes()
to determine whether the DOM element has a certain class name. Here is a sample code:
<template> <div></div> </template> <script> export default { mounted() { const myDiv = this.$refs.myDiv; //获取myDiv元素的引用 if (myDiv.classList.includes('my-class')) { //判断是否有my-class类名 console.log('myDiv has the class "my-class"'); } else { console.log('myDiv does not have the class "my-class"'); } } }; </script>
Method 2: Using $el
Another method is to use the $el
property of the component to get the DOM element of the component. The following is a sample code:
<template> <div></div> </template> <script> export default { mounted() { const myDiv = this.$el; //获取组件的DOM元素 if (myDiv.classList.includes('my-class')) { //判断是否有my-class类名 console.log('the component has the class "my-class"'); } else { console.log('the component does not have the class "my-class"'); } } }; </script>
Summary
You can easily check whether the DOM has a certain value in Vue through $refs
and $el
Class name. Both of the above methods can achieve this function, and the specific method chosen can be determined according to actual needs.
The above is the detailed content of How to check if dom has a class name in vue (two methods). For more information, please follow other related articles on the PHP Chinese website!
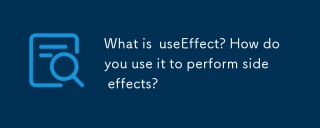
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
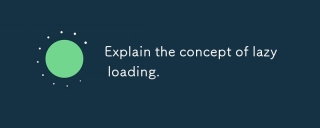
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
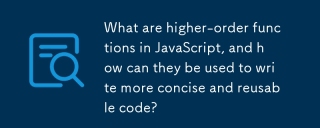
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
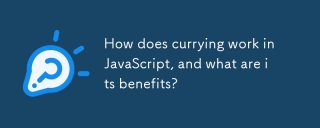
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
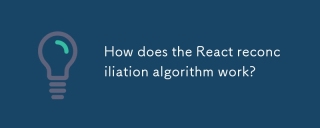
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
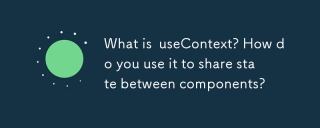
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
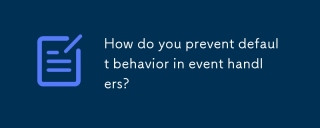
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
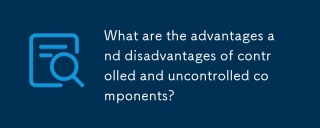
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
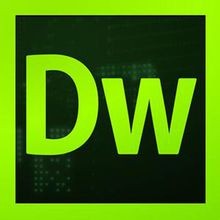
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
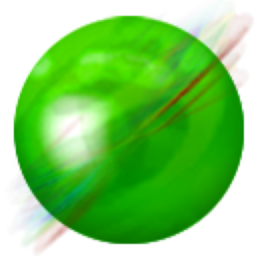
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
