Vue is a popular JavaScript framework that provides developers with a modern way to build web applications. Vuex is a state management model of Vue that allows you to easily manage, share and synchronize state in your application. In this article, we will focus on how to save data using Vuex in a Vue application.
- What is Vuex?
Vuex is an open source state management library that can be used with Vue.js. It allows you to share state among different components of your application and keep it synchronized throughout your application. The core idea of Vuex is the "single state tree", which saves all the state of the application (such as data in components) in a "store". This makes state management more predictable and easier to maintain.
- Installing and setting up Vuex in your Vue application
Before using Vuex, you need to install it in your Vue application. Vuex can be downloaded and installed through the NPM package manager.
In the project, you need to import Vuex before the Vue instance:
import Vue from 'vue'; import Vuex from 'vuex'; Vue.use(Vuex);
Next, you need to add a new Vuex store instance. A Vuex store instance is an object that contains all the state and logic in your application.
const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment (state) { state.count++ } } })
In this example, the store instance will have a state property named "count" and a change method named "increment". This change method will increase the value of count. We can use it in components by:
this.$store.commit('increment')
- How to store data to Vuex
Storing data to Vuex in a Vue application is not complicated. To store data, you need to define a mutations method on the store instance. This method can be called from any component to change state.
Let’s take a look at an example. Let's say we have an array called "todos" in our application and we want to store it in a state property called "todos".
const store = new Vuex.Store({ state: { todos: [] }, mutations: { addTodo (state, todo) { state.todos.push(todo) } } })
In this example, we define a mutations method named addTodo. This method will be called in the component to add a new todo to the state.todos array. Components can call it in the following ways:
this.$store.commit('addTodo', todo)
Note: The "addTodo" parameter is a todo object.
- Summary
As mentioned above, Vuex is a powerful state management library for Vue.js. By using Vuex in your application, you can better manage state and logic. To store data in Vuex, you need to define the mutations method in the store instance. In a component you can use them by calling this.$store.commit. Hope this article is helpful to you.
The above is the detailed content of How to save data using Vuex in Vue application. For more information, please follow other related articles on the PHP Chinese website!
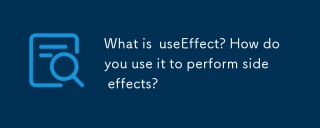
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
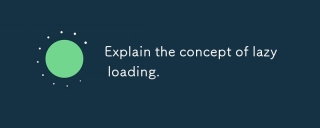
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
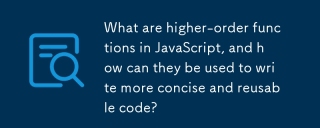
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
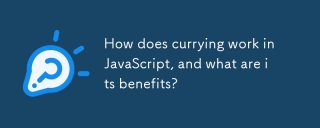
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
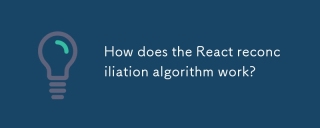
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
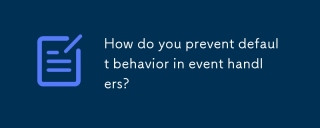
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
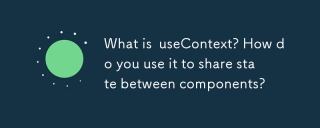
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
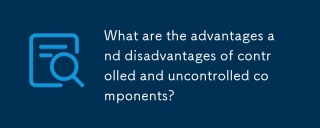
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
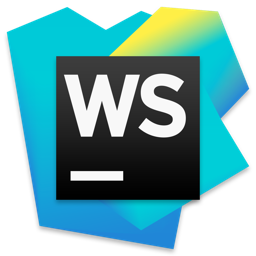
WebStorm Mac version
Useful JavaScript development tools
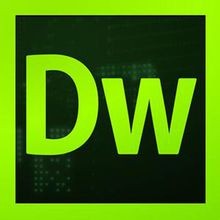
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
