Go language (also known as Golang) is a statically strongly typed, compiled language developed by Google. It is simple and efficient and is widely used in network servers, cloud computing, big data and other fields. In this article, we will introduce how to use Go language to implement a shutdown function.
Implementing shutdown
Before describing how to use Go language to implement shutdown, we need to understand some basic knowledge, such as how to execute system commands. Go language provides the os package to implement the function of executing system commands. The following is a sample program:
package main import ( "os" "os/exec" "fmt" ) func main() { cmd := exec.Command("ls") cmd.Stdout = os.Stdout cmd.Run() }
In this sample program, we execute the ls command by creating an exec.Cmd object and redirect the standard output to os.Stdout. Finally, we call the Run method to execute the command.
Next, we can use this knowledge to implement the shutdown function. In Windows systems, we can use the "shutdown" command to shutdown. The following is the sample code:
package main import ( "os/exec" "fmt" ) func main() { cmd := exec.Command("shutdown", "-s", "-t", "0") err := cmd.Run() if err != nil { fmt.Println(err) } else { fmt.Println("Shutdown command executed successfully.") } }
In this sample program, we execute the Windows "shutdown" command by creating an exec.Cmd object and passing the "-s" and "-t 0" parameters. Among them, "-s" means shutdown, and "-t 0" means shutdown immediately. Finally, we call the Run method to execute the command. If an error occurs during execution, we will output an error message; otherwise, we will output a prompt message.
Note: In Linux systems, the shutdown command is not "shutdown", but "poweroff".
Summary
Through the introduction of this article, we have learned how to use Go language to implement the shutdown function. Using the exec.Cmd object in the os package, we can easily execute system commands. Of course, in practical applications, issues such as system permissions and user interaction also need to be considered. Hope this article is helpful to you.
The above is the detailed content of How to use Go language to implement a shutdown function. For more information, please follow other related articles on the PHP Chinese website!
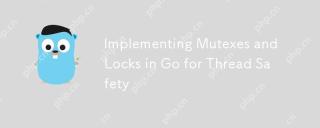
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
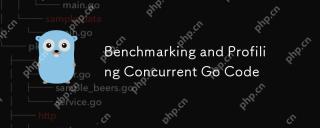
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
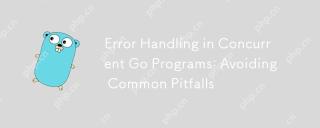
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
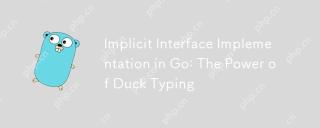
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
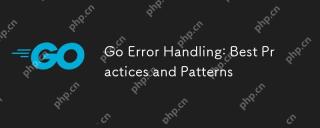
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
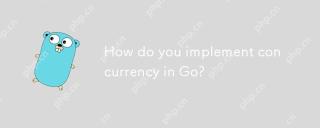
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
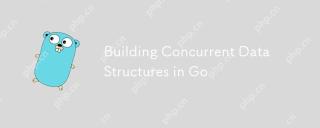
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
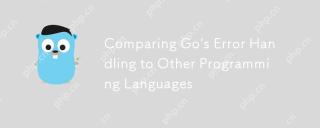
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
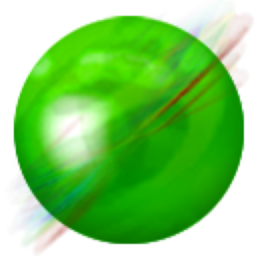
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
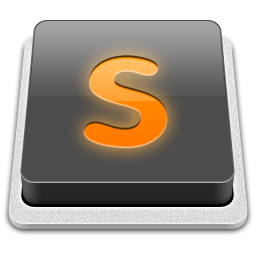
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
