In PHP, we often need to remove certain elements in an array to meet actual needs. So, how to remove elements from the array? This article will cover a few different methods.
1. Use the unset() function
The unset() function can destroy the specified variable and thereby remove elements from the array. For example, we have the following array:
$arr = array('a', 'b', 'c', 'd', 'e');
If we want to delete the third element in the array (that is, the element with index 2), we can use the unset() function:
unset($arr[2]);
In this way, Element 'c' in the array is deleted. You can also delete multiple elements, for example:
unset($arr[1], $arr[3]);
This will delete the second and fourth elements in the array (elements with index 1 and 3). It should be noted that using the unset() function only deletes the elements in the array, but does not rearrange the key values of the array. Therefore, holes will be left in the array. If you need to re-index the array, you can use the array_values() function, for example:
$arr = array_values($arr);
2. Use the array_splice() function
array_splice() function can delete elements in the array and replace them. The deleted element. The syntax of this function is as follows:
array_splice(array &$input, int $offset [, int $length = count($input)], mixed $replacement = [])
Where, $input is the array to be operated on; $offset is the index of the first element to be deleted (or replaced) (if it is a negative number, it is calculated from the end of the array ); $length is the number of elements to be removed (if not specified, all elements from $offset to the end of the array are removed); $replacement is the value used to replace the removed elements.
The sample code is as follows:
$arr = array('a', 'b', 'c', 'd', 'e'); array_splice($arr, 2, 1);
In this way, the element 'c' in the array is deleted. If you want to delete multiple elements, you can set $length to the number of elements to be deleted, for example:
array_splice($arr, 1, 2);
In this way, the second and third elements in the array are deleted. If you want to replace the deleted element, you can set the $replacement parameter to the value to be replaced, for example:
array_splice($arr, 1, 2, array('x', 'y', 'z'));
In this way, the second and third elements in the array are replaced with 'x', ' y' and 'z'.
3. Use the array_diff() function
array_diff() function can return the difference set of two or more arrays. We can put the elements we want to delete into a new array and then use the array_diff() function to remove the elements. The sample code is as follows:
$arr = array('a', 'b', 'c', 'd', 'e'); $remove = array('c', 'd'); $result = array_diff($arr, $remove);
In this way, only 'a', 'b' and 'e' are left in the $result array. It is important to note that the array_diff() function compares the values in the two arrays, not the keys, so if there are identical values in the arrays, only one value will be retained.
4. Use array_filter() function
array_filter() function can filter elements in the array according to specified conditions. We can use this function to remove certain elements from an array. The sample code is as follows:
$arr = array('a', 'b', 'c', 'd', 'e'); $new_arr = array_filter($arr, function($value) { return $value != 'c' && $value != 'd'; });
An anonymous function is used here as the callback function, which returns all elements that are not equal to 'c' and 'd'. This leaves only 'a', 'b' and 'e' in the $new_arr array.
5. Use the unset() function combined with a loop
The last method is to use the unset() function combined with a loop to remove elements from the array. The specific operation is: traverse the array, find the element to be deleted, and then use the unset() function to delete it. The sample code is as follows:
$arr = array('a', 'b', 'c', 'd', 'e'); foreach ($arr as $key => $value) { if ($value == 'c' || $value == 'd') { unset($arr[$key]); } }
In this way, the 'c' and 'd' elements in the array are deleted. It should be noted that when using the unset() function to delete an array element, the key value of the array will be changed. Therefore, you need to use reference passing when traversing the array. If you need to reindex an array, you can use the array_values() function.
Summary
This article introduced several different methods that can be used to remove elements from PHP arrays. Different methods are suitable for different scenarios. Choosing the appropriate method can realize the requirements more efficiently. If you have other good methods, please leave a message in the comment area to share!
The above is the detailed content of How to remove elements from an array in php (5 methods). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
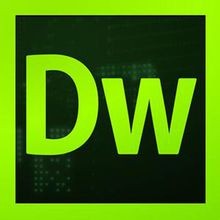
Dreamweaver CS6
Visual web development tools
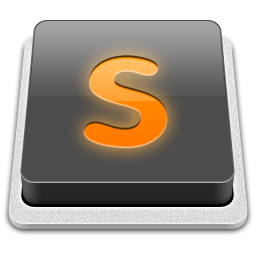
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
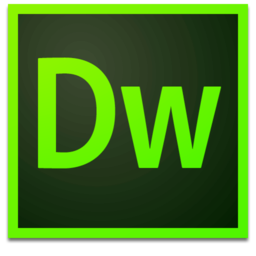
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
