With the rapid development of front-end technology, Vue.js has become a widely used Javascript framework in the industry. It can be used to build complex single-page web applications. This article will introduce how to use Vue to build a basic system.
1. Getting started
First, we need to install Vue locally. You can directly download the official Vue.js library, or use npm to install it as follows:
npm install vue
Introduce the Vue.js library into the HTML page:
<script></script>
You can use the Vue CLI (command line interface) Let’s quickly build a new project. Here we use Vue CLI 3 to create a new project.
npm install -g @vue/cli vue create my-project
There will be a series of configuration options that you need to fill in. After selecting, you can create a new Vue project.
2. Create components
Vue adopts the MVVM mode, that is, the view layer and data layer are separated. Through Vue.js, we can quickly create components, and a component is equivalent to a container of data.
Creating a component is very simple. You only need to register a component in the Vue instance and declare its required data and methods. The following is a simple example of creating a component:
<div> <my-component></my-component> </div>
Vue.component('my-component', { data: function () { return { message: 'Hello Vue!' } }, template: '<div>{{ message }}</div>' }) new Vue({ el: '#app' })
In the above code, we registered a component named "my-component" in the Vue instance through the Vue.component
method . Its data comes from the message
attribute in the data
method.
Finally, mount the my-component
component to the specified HTML element in the Vue instance.
3. Component communication
In Vue, communication between components is a relatively common requirement. The following explains the communication methods between components.
3.1 Props
Props are a way to pass data to child components. It allows parent components to pass data to child components through property binding.
In the parent component template:
<template> <child-component></child-component> </template> <script> export default { data() { return { messageFromParent: 'parent message' } } } </script>
In the child component template:
<template> <div>{{ message }}</div> </template> <script> export default { props: { message: String } } </script>
In the above code, we created the parent component in the template
tag A child component named "child-component" and passed a string named messageFromParent using the :message
attribute.
The props
of a subcomponent is an object used to define the properties and types that the current component can receive. In this example, the child component uses only one message
attribute, and its type is String
.
3.2 Event
Event is a way to allow child components to communicate with parent components. It allows child components to notify parent components that something has happened through events.
In the child component template:
<template> <div>click me</div> </template> <script> export default { methods: { onChildClick() { this.$emit('child-click') } } } </script>
In the parent component template:
<template> <child-component></child-component> </template> <script> export default { methods: { onChildClick() { console.log('child clicked') } } } </script>
In the above code, @click
in the child component is monitored A click event, and an event named "child-click" is emitted to the parent component through the $emit
method.
<child-component></child-component>
in the parent component uses @child-click
to listen to the event, and when the "onChildClick" method is triggered, control The station will output "child clicked".
4. Routing
In Vue, routing is an important concept. It allows us to jump to different pages based on different URLs. The Vue framework uses Vue Router to implement routing functions.
First you need to install Vue Router in the project:
npm install vue-router --save
Create a routing component in the router.js file:
import Vue from 'vue' import Router from 'vue-router' import Home from './views/Home.vue' import About from './views/About.vue' Vue.use(Router) export default new Router({ mode: 'history', routes: [ { path: '/', name: 'home', component: Home }, { path: '/about', name: 'about', component: About } ] })
Use Vue.use
To install Vue Router, and then define two routes, namely the homepage and about page, and specify the component corresponding to each route through the component
attribute.
Finally, you need to introduce the routing component into the Vue instance:
import Vue from 'vue' import router from './router' import App from './App.vue' new Vue({ el: '#app', router, render: h => h(App) })
5. Summary
In this article, we briefly introduced how to use Vue to build a basic system. From getting started to component communication and routing, we have only touched on a small part of Vue. Vue has many powerful features and capabilities. I hope this article can be of some help to beginners.
The above is the detailed content of How to use Vue to build a basic system. For more information, please follow other related articles on the PHP Chinese website!

React’s popularity includes its performance optimization, component reuse and a rich ecosystem. 1. Performance optimization achieves efficient updates through virtual DOM and diffing mechanisms. 2. Component Reuse Reduces duplicate code by reusable components. 3. Rich ecosystem and one-way data flow enhance the development experience.

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
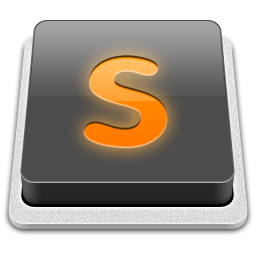
SublimeText3 Mac version
God-level code editing software (SublimeText3)