Golang (also known as Go) is a modern, fast and concurrent programming language. Go provides many built-in types and data structures, the most commonly used of which is map. Map is one of the most commonly used data structures in Go programming. It provides a simple key-value storage solution and supports fast query and modification operations, so it is essential in Go programming.
When using map, we usually need to perform query operations to obtain the value corresponding to a specific key value. So, how to perform map query operations? This article will introduce the method of Golang map query.
Basic usage of map query
map is a reference type. You can create an empty map using the make function. The syntax of the make function is as follows:
make(map[KeyType]ValueType, [cap])
where KeyType is the key Type, ValueType is the type of value, cap is the optional map capacity, indicating the initial capacity of the map.
The following is a sample program that creates a map, adds elements and queries:
package main import "fmt" func main() { // 创建一个空的 map scores := make(map[string]int) // 添加键值对 scores["Alice"] = 90 scores["Bob"] = 85 scores["Charlie"] = 80 // 查询键值对应的值 fmt.Println(scores["Bob"]) // 输出 85 }
In the above sample program, we first use the make function to create an empty map, and then pass the scores[key ] = value adds three sets of key-value pairs. Finally, we use scores["Bob"] to query Bob's scores and output the results.
It should be noted that when using map query, if the specified key does not exist, a zero value of the corresponding value type, such as nil, will be returned. In the above example, if we query for a key that does not exist, such as scores["David"], a zero value of type int 0 will be returned.
Determine whether the specified key exists in the map
In actual programming, we often need to determine whether the specified key exists in the map. The method to determine whether the specified key exists in the map is very simple. Just use the comma operator directly:
value, ok := scores["Bob"] if ok == true { fmt.Println("Bob's score is", value) } else { fmt.Println("Bob's score does not exist") }
In the above code, we use the comma operator to receive the return value of scores["Bob"] and ok at the same time. Boolean value. If ok is true, it means that the key Bob exists in scores and its value can be obtained; otherwise, it means that this key does not exist in scores.
Traverse map
When using map, we often need to traverse all key-value pairs in the map. Go provides the range keyword for iterating over the elements in a map.
The following is a sample program for traversing the map:
package main import "fmt" func main() { // 创建一个 map scores := map[string]int{ "Alice": 90, "Bob": 85, "Charlie": 80, } // 遍历 map for key, value := range scores { fmt.Println(key, value) } }
In the above code, we use the range keyword to iterate through all key-value pairs in the scores map and output their key sums value.
Summary
In Golang, map is a very powerful data structure, which provides a simple key-value storage solution and supports fast query and modification operations. This article introduces the basic usage of map queries, methods of determining whether a specified key exists in the map, and traversing the map. Mastering this knowledge allows programmers to use the Golang programming language more efficiently.
The above is the detailed content of How to operate map elements in golang. For more information, please follow other related articles on the PHP Chinese website!
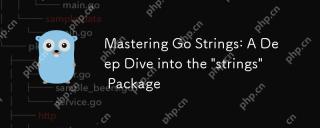
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
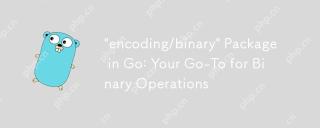
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
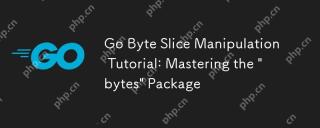
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
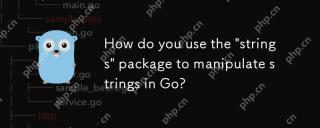
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
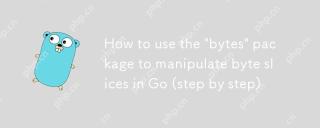
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
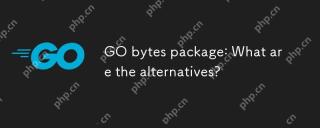
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
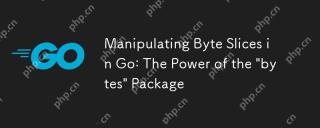
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
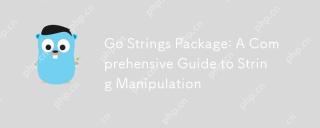
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
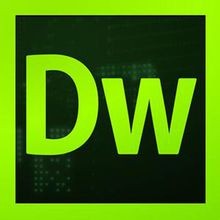
Dreamweaver CS6
Visual web development tools
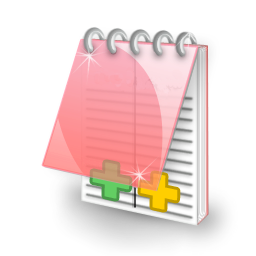
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
