In the Go language, there is no concept of class, so there is no inheritance in traditional object-oriented languages. However, in the Go language, an inheritance-like effect can be achieved through the combination of structures and anonymous fields.
First, let us look at the usage of combination in go language. Composition refers to embedding one structure within another structure to achieve code reuse and modularization. For example, we can define a Person structure:
type Person struct { Name string Age int }
Then, we can embed the Person structure in the Student structure:
type Student struct { Person School string }
In this way, the Student structure not only has its own Properties, you can also access properties in the parent class through the Person property. For example:
s := Student{ Person: Person{Name: "Tom", Age: 18}, School: "CMS", } fmt.Println(s.Name) // Tom fmt.Println(s.Age) // 18
You can also access methods in the parent class through the embedded Person attribute:
func (p *Person) SayHello() { fmt.Printf("Hello, my name is %s.\n", p.Name) } type Student struct { Person School string } func main() { s := Student{ Person: Person{Name: "Tom", Age: 18}, School: "CMS", } s.SayHello() // Hello, my name is Tom. }
However, if we want to define a method in the Student structure with the same name as the Person structure ,what can we do about it? At this time, you need to use the characteristics of anonymous fields.
Anonymous field is a special field type that does not specify a name, only a type. Through anonymous fields, variables and methods with the same name in the parent class can be referenced in the subclass. We can use the following method to define a method with the same name as the Person structure in the Student structure:
type Person struct { Name string Age int } func (p *Person) SayHello() { fmt.Printf("Hello, my name is %s.\n", p.Name) } type Student struct { Person School string } func (s *Student) SayHello() { fmt.Printf("Hello, my name is %s and I am a student of %s.\n", s.Name, s.School) } func main() { s := Student{ Person: Person{Name: "Tom", Age: 18}, School: "CMS", } s.SayHello() // Hello, my name is Tom and I am a student of CMS. }
In the above code, we define the SayHello method with the same name as the Person structure in the Student structure, and The properties in the parent class are accessed through s.Name and s.School.
In summary, the Go language does not have the concept of inheritance, but through the combination of structures and anonymous fields, effects similar to inheritance can be achieved.
The above is the detailed content of Detailed explanation of how golang achieves inheritance effect. For more information, please follow other related articles on the PHP Chinese website!
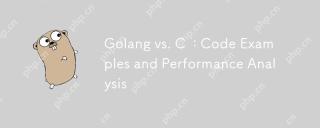
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
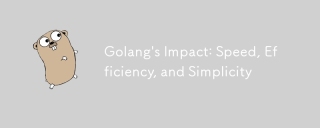
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
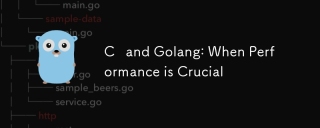
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
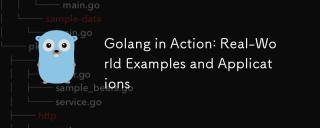
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
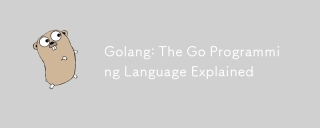
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
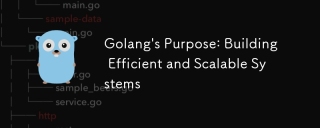
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
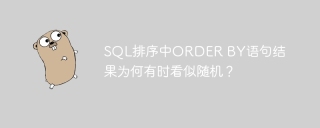
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
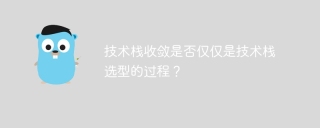
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
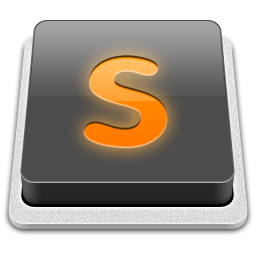
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
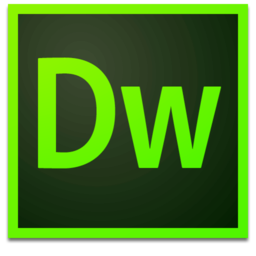
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool