In PHP, it is very common to use AES CBC mode for encryption and decryption. However, when using AES CBC mode to decrypt, you may encounter garbled characters. This problem is very common, but easy to solve. In this article, I will introduce how to normally decrypt AES CBC encrypted data through PHP and avoid garbled characters.
1. Problem description
In PHP, we can use openssl_encrypt and openssl_decrypt functions to perform encryption and decryption operations respectively. For example, the following code uses AES CBC mode to encrypt data:
$key = '1234567890123456'; $data = 'hello world'; $iv = '1234567890123456'; $encrypted = openssl_encrypt($data, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, $iv);
where $key is the key, $data is the data to be encrypted, and $iv is the initial vector. After encryption, the $encrypted variable will get the encrypted data. Now, we need to decrypt it:
$key = '1234567890123456'; $encrypted = 'soLPpFUpwJdVEaYpuu6zRg=='; $iv = '1234567890123456'; $decrypted = openssl_decrypt($encrypted, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, $iv); echo $decrypted;
However, after running the above code, you may get the following output:
纭洏鍥?J 鍗虫槑涓?
This is a piece of gibberish, not the original data "hello world ". This is because the decryption function has strict limits on the length of the input parameters, so if the length of the data to be decrypted is incorrect, garbled characters will occur.
2. Solution
In order to solve this problem, we need to understand some basic knowledge of AES CBC mode.
In AES CBC mode, both encryption and decryption require an initialization vector (Initialization Vector, IV). This initial vector needs to use the same value during encryption and decryption, otherwise it will cause data errors during decryption.
The length of the initialization vector must be equal to the block size required by the encryption algorithm. For example, when using 128-bit AES CBC mode, the initialization vector must be 16 bytes long (128 bits / 8 bits). If this requirement is not met, garbled characters or other problems will occur during decryption.
Therefore, we need to check whether the length of the input parameter meets the requirements. When the length is illegal, padding operation is required. Padding can be done before decryption to ensure that the length of the input data is the same as that used when encrypting.
In view of the above problems and solutions, we can modify the original code to adapt to the requirements of AES CBC mode, as shown below:
$key = '1234567890123456'; $encrypted = 'soLPpFUpwJdVEaYpuu6zRg=='; $iv = '1234567890123456'; // 检查初始向量长度是否正确 if (strlen($iv) != 16) { echo 'Error: IV length is not valid!'; exit; } // 检查输入参数长度是否正确 $decrypted = openssl_decrypt($encrypted, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, $iv); if ($decrypted === false) { // 进行填充操作 $padded_data = $encrypted . str_repeat(chr(16), 16 - (strlen($encrypted) % 16)); $decrypted = openssl_decrypt($padded_data, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, $iv); } echo $decrypted;
In the above code, first check whether the initial vector length is correct. If it is incorrect, an error is output and the program exits. Next, the input data length before decryption is checked. If the length is incorrect, padding is performed to ensure that the data length meets the requirements.
In this way, garbled characters can be avoided during decryption.
3. Summary
When using AES CBC mode for encryption and decryption, if the length of the input parameter is incorrect, decryption may fail and garbled characters may appear. To avoid this problem, we need to check the length of the input parameters and perform necessary padding.
The above is my solution to the problem of decrypting garbled characters in PHP AES CBC mode. Hope it helps.
The above is the detailed content of How to solve php aes cbc decryption garbled code. For more information, please follow other related articles on the PHP Chinese website!
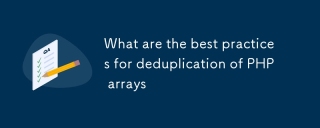
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
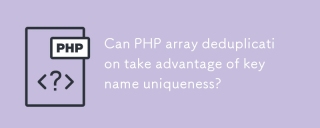
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
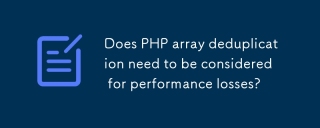
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
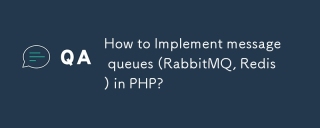
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
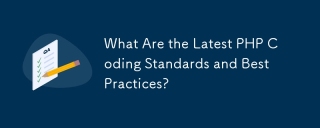
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
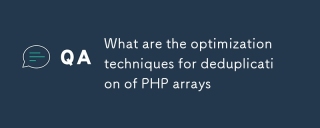
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
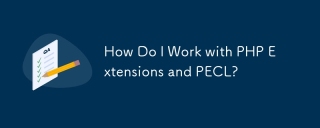
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
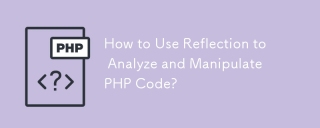
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
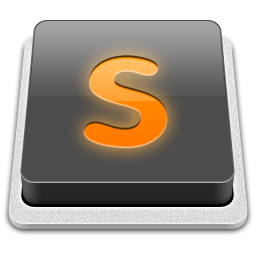
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
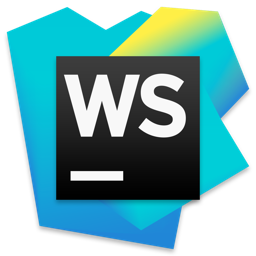
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
