Golang is a fast and convenient programming language that has received more and more widespread attention and use in recent years. In basic Golang programming, it is often necessary to change the font of text. This article will introduce how to use Golang to change the font.
The basic steps to change the font are:
- Download the font
- Install the font to the computer
- Use Golang to print and use the font
Step one: Download fonts
There are many ways to download fonts, but the easiest way is to download them online and choose the fonts that meet your needs. After downloading the fonts, we need to move them to the folder of our Golang project.
Step 2: Install fonts to the computer
Font files usually have a .ttf or .otf suffix. Under Windows systems, we can copy these font files to the Fonts of the operating system. folder. Under Mac system, we need to copy the font files to the /Library/Fonts or ~/Library/Fonts folder.
Step 3: Use Golang to print and use fonts
Golang provides a method to output fonts in the console. We can use the Println function in the built-in fmt package in Golang to print the string with changed font. However, it is important to note that we need to use the fonts installed in the second step to print the output.
The sample code is as follows:
package main import ( "fmt" "golang.org/x/image/font" "golang.org/x/image/font/basicfont" "golang.org/x/image/font/inconsolata" "golang.org/x/image/font/opentype" "image" "image/color" "image/draw" "image/png" "io/ioutil" "os" ) func main() { tempFile, err := downloadFontTemporarily() if err != nil { fmt.Printf("Failed to download the font: %v", err) return } // Cleanup the temp file. defer os.Remove(tempFile.Name()) fontBytes, err := ioutil.ReadFile(tempFile.Name()) if err != nil { fmt.Printf("Failed to read the font: %v", err) return } // Parse the font, allowing for a variety of font types. f, err := opentype.Parse(fontBytes) if err != nil { fmt.Printf("Failed to parse the font: %v", err) return } const size = 72 d := &font.Drawer{ Dst: draw.NewRGBA(image.Rect(0, 0, 1024, 1024)), Src: image.NewUniform(color.White), Face: truetype.NewFace(f, &truetype.Options{Size: size}), } d.Dot = fixed.Point26_6{ X: (fixed.I(10)), Y: (fixed.I(50)), } d.DrawString("Hello World!") img := d.Dst.(*draw.RGBA) png.Encode(os.Stdout, img) } func downloadFontTemporarily() (*os.File, error) { // Download a font so we can draw it. resp, err := http.Get("https://storage.googleapis.com/golang/go1.9beta1.linux-amd64.tar.gz") if err != nil { return nil, fmt.Errorf("Failed to open source image: %v", err) } // Write the file to a temporary directory so `font.OpenType` can use it. tempFile, err := ioutil.TempFile("", "font.ttf") if err != nil { return nil, fmt.Errorf("Failed to create temp file: %v", err) } // Cleanup the temporary file, defer os.Remove(tempFile.Name()) if _, err = io.Copy(tempFile, resp.Body); err != nil { return nil, fmt.Errorf("Failed to write font to temp file: %v", err) } // Seek back to the start of the file so it can be read again later. if _, err = tempFile.Seek(0, io.SeekStart); err != nil { return nil, fmt.Errorf("Failed to seek to start of temporary file: %v", err) } return tempFile, nil }
This is a sample program that prints the "Hello World!" string. It uses the specified font and uses the DrawString function to draw the characters on the picture. , and finally convert the image to PNG format and output it to the standard output.
For different fonts, we can also use the built-in font package or basicfont package in Golang or the golang.org/x/image/font/inconsolata package to draw fonts.
Summary
Through the introduction of this article, we can see that the method of changing fonts in Golang is very simple. Just download the font, install the font, and then use the Println function in Golang to output the specified font. At the same time, by using the built-in font package or basicfont package or golang.org/x/image/font/inconsolata package to draw fonts, we can greatly increase the flexibility and scalability of Golang applications.
The above is the detailed content of How to change font with Golang. For more information, please follow other related articles on the PHP Chinese website!
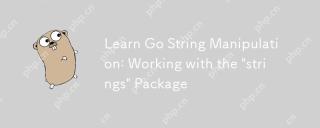
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
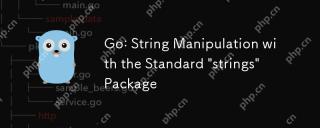
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
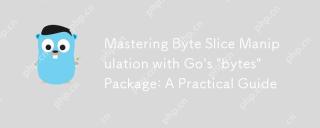
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
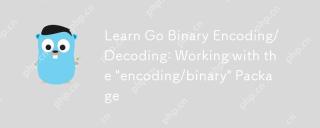
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
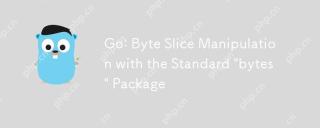
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
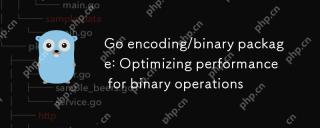
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
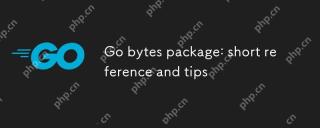
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
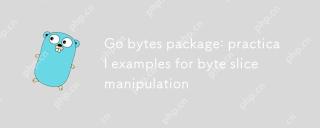
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
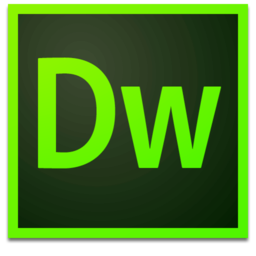
Dreamweaver Mac version
Visual web development tools
