PHP is a widely used programming language and it is widely used for web development. In PHP, date is a common data type because many web applications need to involve dates and times. PHP provides built-in date and time functions that can convert a date represented by a string into a date object. In this article, we will learn how to convert PHP string to date.
Convert a string to a date using PHP built-in functions
PHP provides built-in date and time functions, some of which can convert a date represented by a string into a date object. Here are some examples of these functions:
strtotime()
The strtotime() function accepts a string representing a date and time and converts it to Unix timestamp format. Its basic syntax is as follows:
strtotime(string $time, [int $now])
$time
The parameter is a string representing the date and time, which can be in multiple formats, such as: YYYY-MM-DD HH:MM:SS or MM/DD/YYYY etc. $now
The optional parameter represents the Unix timestamp of the current time.
The following is a sample code that uses strtotime() to convert from a string to a date:
$dateString = '2020-05-31 15:45:00'; $date = strtotime($dateString); echo date('Y-m-d H:i:s', $date); // 输出:2020-05-31 15:45:00
In the above code, we first define a $dateString
string, which Represents 2020-05-31 15:45:00
, and then passes it as a parameter to the strtotime() function. This function returns a Unix timestamp, so we can use the date() function to format it into a date and time string.
DateTime::createFromFormat()
The DateTime::createFromFormat() method creates a new DateTime object from a formatted string. Its basic syntax is as follows:
DateTime::createFromFormat(string $format, string $time, [DateTimeZone $timezone])
$format
The parameter is a format string describing the format of the $time
parameter. $time
The parameters are strings representing date and time. $timezone
The parameter is the time zone, optional. (Defaults to the server's time zone).
The following is a sample code using DateTime::createFromFormat() to convert from a string to a date:
$dateString = '2020-05-31 15:45:00'; $date = DateTime::createFromFormat('Y-m-d H:i:s', $dateString); echo $date->format('Y-m-d H:i:s'); // 输出:2020-05-31 15:45:00
In the above code, we first define a $dateString
A string that represents 2020-05-31 15:45:00
, which is then passed as a parameter to the DateTime::createFromFormat() method. This method returns a DateTime object and we use the format() method to format the date and time string.
Use third-party libraries to convert strings to dates
In addition to using PHP built-in functions, there are many third-party libraries that can help us convert strings to dates. Here are some popular PHP date parsing libraries:
- Carbon
- Noodlehaus\Time
- JDateTime
Here we will Introduces how to use the Carbon library to convert strings to dates. Carbon is a popular date processing library that provides many easy-to-use methods. The following is a sample code to convert from a string to a date using the Carbon library:
use Carbon\Carbon; $dateString = '2020-05-31 15:45:00'; $date = Carbon::parse($dateString); echo $date->format('Y-m-d H:i:s'); // 输出:2020-05-31 15:45:00
In the above code, we first imported the Carbon library and defined a $dateString
string , and then use the Carbon::parse() method to convert it to a Carbon object. Finally, we use the format() method to format the date and time into a string.
Conclusion
Converting a string to a date is a common task in PHP, especially in web applications. This article explains how to convert a string into a date object using PHP's built-in functions and third-party libraries. No matter which method you choose, these functions and libraries can help you convert strings to dates and provide easy-to-use and flexible ways to format date objects.
The above is the detailed content of How to convert php string to date. For more information, please follow other related articles on the PHP Chinese website!
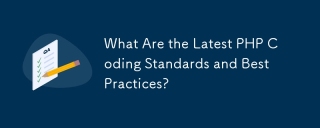
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
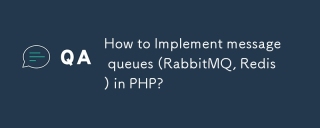
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
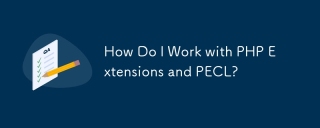
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
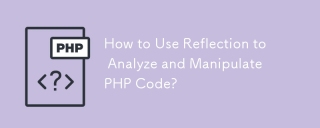
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
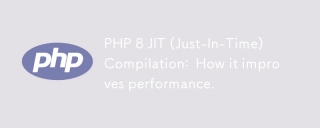
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
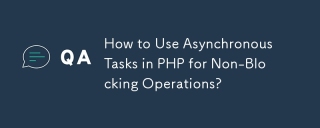
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
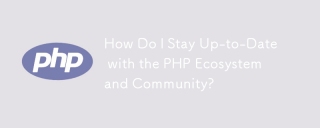
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
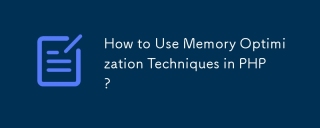
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
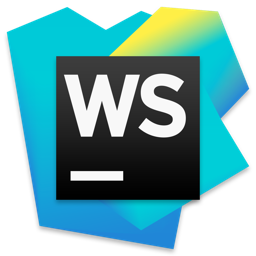
WebStorm Mac version
Useful JavaScript development tools
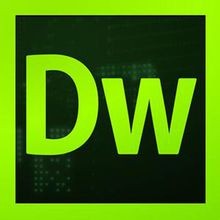
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
