Golang is a rapidly growing programming language. Its simplicity, ease of use and efficient running speed have attracted more and more developers to use it. In Golang, time-related operations are essential, and this article will focus on how to perform time increase operations in Golang.
1. Overview of time
In Golang's time package, time is expressed using the time.Time structure, which includes year, month, day, hour, minute, second and Time elements such as nanoseconds. At the same time, there are many functions that can manipulate time in this package, so that we can increase time.
2. Time increase method
In Golang, the time is increased in different units such as year, month, day, hour, minute, second and so on. Different time increasing methods will be introduced below.
- Add year
To add a year, you can use the AddDate method. The prototype of this method is as follows:
func (t Time) AddDate(years int, months int, days int) Time
Among them, years represents the number of years to add, months Indicates the number of months added, and days indicates the number of days added. The following is a specific example:
package main import ( "fmt" "time" ) func main() { t := time.Now() fmt.Println("添加1年后的时间:", t.AddDate(1, 0, 0)) fmt.Println("添加1年1个月后的时间:", t.AddDate(1, 1, 0)) fmt.Println("添加2年2个月3天后的时间:", t.AddDate(2, 2, 3)) }
The output result is as follows:
添加1年后的时间: 2021-06-05 19:41:52.4936932 +0800 CST m=+315.743666501 添加1年1个月后的时间: 2021-07-05 19:41:52.4936932 +0800 CST m=+345.743666501 添加2年2个月3天后的时间: 2023-08-08 19:41:52.4936932 +0800 CST m=+805.743666501
- Add the month
To add the month, you can use the AddDate method, the definition of this method As mentioned above. The following is a specific example:
package main import ( "fmt" "time" ) func main() { t := time.Now() fmt.Println("添加2个月后的时间:", t.AddDate(0, 2, 0)) fmt.Println("添加4个月5天后的时间:", t.AddDate(0, 4, 5)) }
The output result is as follows:
添加2个月后的时间: 2021-08-05 19:41:52.4936932 +0800 CST m=+798.743666501 添加4个月5天后的时间: 2021-10-10 19:41:52.4936932 +0800 CST m=+849.743666501
- Increase the number of days
To increase the number of days, you can use the Add method, the prototype of this method As follows:
func (t Time) Add(d Duration) Time
Among them, d represents the increased time period. The following is a specific example:
package main import ( "fmt" "time" ) func main() { t := time.Now() fmt.Println("添加2天后的时间:", t.Add(48*time.Hour)) fmt.Println("添加3小时后的时间:", t.Add(3*time.Hour)) }
The output results are as follows:
添加2天后的时间: 2021-06-07 19:41:52.4936932 +0800 CST m=+558.743666501 添加3小时后的时间: 2021-06-06 22:41:52.4936932 +0800 CST
- Increase the number of hours and minutes
Same as increasing the number of hours and minutes You can use the Add method, as shown below:
package main import ( "fmt" "time" ) func main() { t := time.Now() fmt.Println("添加2小时后的时间:", t.Add(2*time.Hour)) fmt.Println("添加30分钟后的时间:", t.Add(30*time.Minute)) }
The output result is as follows:
添加2小时后的时间: 2021-06-05 21:41:52.4936932 +0800 CST 添加30分钟后的时间: 2021-06-05 20:11:52.4936932 +0800 CST
- Increase the number of seconds
To increase the number of seconds, you can use the Add method, As shown below:
package main import ( "fmt" "time" ) func main() { t := time.Now() fmt.Println("添加20秒后的时间:", t.Add(20*time.Second)) fmt.Println("添加120秒后的时间:", t.Add(120*time.Second)) }
The output result is as follows:
添加20秒后的时间: 2021-06-05 19:42:12.4936932 +0800 CST 添加120秒后的时间: 2021-06-05 19:43:52.4936932 +0800 CST
3. Summary
The time increase operation is one of the methods often used in development. Through the introduction of this article , we can see that in Golang, the time increases from different units such as years, months, days, hours, minutes, seconds, etc. At the same time, you can use the AddDate and Add methods to complete method operations on time. I hope this article can be helpful to everyone.
The above is the detailed content of How to do time increment operation in Golang. For more information, please follow other related articles on the PHP Chinese website!
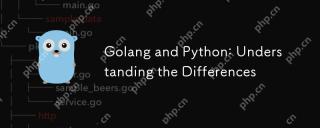
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
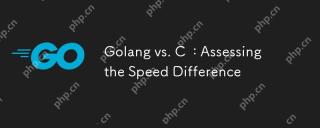
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
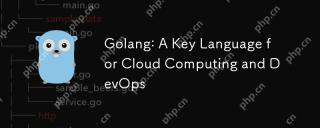
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
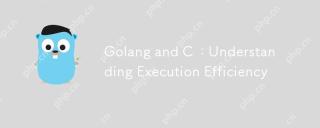
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
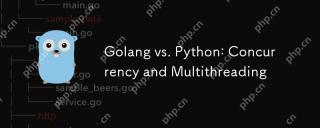
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
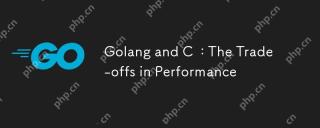
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
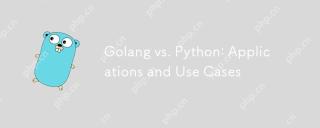
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
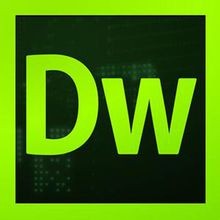
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor

Zend Studio 13.0.1
Powerful PHP integrated development environment
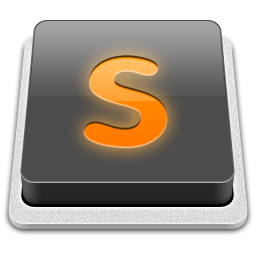
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software