jQuery is a popular JavaScript library designed to simplify operations such as HTML document traversal, event handling, animation, and AJAX operations. When using jQuery, you can also use JavaScript to enhance its functionality. This article will introduce how to use JavaScript in jQuery.
- Using JavaScript syntax in jQuery
jQuery itself is based on the JavaScript language, and jQuery code can also be used in conjunction with native JavaScript code. For example, you can program using JavaScript concepts such as control flow statements, variables, and functions.
Compared with native JavaScript, jQuery's syntax is more concise and easier to read. For example, you can use jQuery's selector to select elements through CSS selectors. The example is as follows:
// 使用原生JavaScript选择并操作元素 var element = document.querySelector('#myElement'); element.style.color = 'red'; // 使用jQuery选择并操作元素 $('#myElement').css('color', 'red');
- Using JavaScript API in jQuery
jQuery can also use JavaScript. API to enhance its functionality. For example, you can use the event handling methods provided by JavaScript to bind events and submit AJAX requests.
// 使用原生JavaScript实现AJAX请求 var xhr = new XMLHttpRequest(); xhr.open('GET', '/api/data', true); xhr.onload = function() { console.log(xhr.responseText); }; xhr.send(); // 使用jQuery实现AJAX请求 $.ajax({ url: '/api/data', type: 'GET', success: function(response) { console.log(response); } });
In addition, jQuery can also use the libraries provided by JavaScript to handle dates, forms, mathematical operations, etc. For example, you can use moment.js to handle date formatting, use the Math library to perform mathematical operations, and so on.
// 使用原生JavaScript格式化日期 var date = new Date(); var formattedDate; formattedDate = date.getFullYear() + '-' + (date.getMonth() + 1) + '-' + date.getDate() + ' ' + date.getHours() + ':' + date.getMinutes() + ':' + date.getSeconds(); console.log(formattedDate); // 使用moment.js格式化日期 console.log(moment().format('YYYY-MM-DD HH:mm:ss')); // 使用原生JavaScript实现数学运算 var num1 = 10; var num2 = 5; console.log(num1 + num2); // 使用Math库实现数学运算 console.log(Math.floor(Math.random() * 10));
- Using JavaScript plugins with jQuery
There are a large number of plugins in the jQuery ecosystem that provide high-quality functionality extensions. These plug-ins are also written based on JavaScript language and can provide more powerful and customizable functions.
For example, you can use the jQuery UI plug-in to achieve interactive effects such as pop-up windows, drag-and-drop, sorting, and date selection. jQuery UI provides a large number of preset effects and is highly scalable and can be customized according to your own needs.
nbsp;html> <meta> <title>jQuery UI demo</title> <link> <script></script> <script></script> <script> $(function() { // 拖拽效果 $('#draggable').draggable(); // 日期选择器 $('#datepicker').datepicker(); // 弹窗效果 $('#dialog').dialog({ autoOpen: false, show: { effect: "blind", duration: 1000 }, hide: { effect: "explode", duration: 1000 } }); $('#opener').click(function() { $('#dialog').dialog('open'); }); }); </script> <!-- 拖拽效果 --> <div> <p>Drag me around</p> </div> <!-- 日期选择器 --> <label>Select a date:</label> <input> <!-- 弹窗效果 --> <button>Open Dialog</button> <div> <p>This is the default dialog which is useful for displaying information. The dialog window can be moved, resized and closed with the 'x' icon.</p> </div>
Summary:
Using JavaScript in jQuery can achieve more flexible, powerful, and customizable effects. You can use JavaScript's native syntax, API, third-party libraries, plug-ins and other methods to enhance the functions of jQuery. For better code readability and maintenance, it is recommended to use jQuery's syntax and methods as uniformly as possible.
The above is the detailed content of Let's talk about how to use JavaScript in jQuery. For more information, please follow other related articles on the PHP Chinese website!

No,youshouldn'tusemultipleIDsinthesameDOM.1)IDsmustbeuniqueperHTMLspecification,andusingduplicatescancauseinconsistentbrowserbehavior.2)Useclassesforstylingmultipleelements,attributeselectorsfortargetingbyattributes,anddescendantselectorsforstructure

HTML5aimstoenhancewebcapabilities,makingitmoredynamic,interactive,andaccessible.1)Itsupportsmultimediaelementslikeand,eliminatingtheneedforplugins.2)Semanticelementsimproveaccessibilityandcodereadability.3)Featureslikeenablepowerful,responsivewebappl

HTML5aimstoenhancewebdevelopmentanduserexperiencethroughsemanticstructure,multimediaintegration,andperformanceimprovements.1)Semanticelementslike,,,andimprovereadabilityandaccessibility.2)andtagsallowseamlessmultimediaembeddingwithoutplugins.3)Featur

HTML5isnotinherentlyinsecure,butitsfeaturescanleadtosecurityrisksifmisusedorimproperlyimplemented.1)Usethesandboxattributeiniframestocontrolembeddedcontentandpreventvulnerabilitieslikeclickjacking.2)AvoidstoringsensitivedatainWebStorageduetoitsaccess

HTML5aimedtoenhancewebdevelopmentbyintroducingsemanticelements,nativemultimediasupport,improvedformelements,andofflinecapabilities,contrastingwiththelimitationsofHTML4andXHTML.1)Itintroducedsemantictagslike,,,improvingstructureandSEO.2)Nativeaudioand

Using ID selectors is not inherently bad in CSS, but should be used with caution. 1) ID selector is suitable for unique elements or JavaScript hooks. 2) For general styles, class selectors should be used as they are more flexible and maintainable. By balancing the use of ID and class, a more robust and efficient CSS architecture can be implemented.

HTML5'sgoalsin2024focusonrefinementandoptimization,notnewfeatures.1)Enhanceperformanceandefficiencythroughoptimizedrendering.2)Improveaccessibilitywithrefinedattributesandelements.3)Addresssecurityconcerns,particularlyXSS,withwiderCSPadoption.4)Ensur

HTML5aimedtoimprovewebdevelopmentinfourkeyareas:1)Multimediasupport,2)Semanticstructure,3)Formcapabilities,and4)Offlineandstorageoptions.1)HTML5introducedandelements,simplifyingmediaembeddingandenhancinguserexperience.2)Newsemanticelementslikeandimpr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
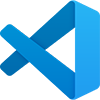
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
