ThinkPHP is a very powerful PHP framework that provides a wealth of functions and tools to help PHP developers quickly build efficient web applications. In ThinkPHP, querying data that meets specific conditions is a very common operation. One common operation is to query the value of a specified field. In this article, we will introduce how to query the value of a specified field using ThinkPHP.
Step 1: Connect to the database
Before using ThinkPHP to query the database, you first need to set the database connection information in the configuration file. Open the database.php configuration file in the conf directory and set the database connection information:
return [ // 数据库类型 'type' => 'mysql', // 服务器地址 'hostname' => 'localhost', // 数据库名 'database' => 'test', // 用户名 'username' => 'root', // 密码 'password' => '', // 端口 'hostport' => '3306', // 数据库编码默认采用utf8 'charset' => 'utf8', ];
Step 2: Query the value of the specified field
It is very simple to use ThinkPHP to query the value of the specified field. We can use the select method to query the value of a specified field. For example, we have a user table which contains id, username and password fields. To query the usernames of all users, you can use the following code:
// 创建一个User模型实例 $user = new \app\model\User(); // 查询所有用户的用户名 $usernames = $user->field('username')->select(); // 打印用户名 foreach ($usernames as $username) { echo $username['username'] . "<br>"; }
In the above code, we first create a User model instance. Then, we use the field method to specify the field name we want to query. Finally, we use the select method to query the value of the specified field. In the foreach loop, we printed each username.
Step 3: Query the value of a specified field in a single record
Sometimes, we only need to query the value of a specified field in a single record. We can use the find method to achieve this operation. For example, if we want to query the username of the user with id 1, we can use the following code:
// 创建一个User模型实例 $user = new \app\model\User(); // 查询id为1的用户的用户名 $username = $user->where('id', 1)->value('username'); // 打印用户名 echo $username;
In the above code, we first create a User model instance. Then, we use the where method to specify the record with id equal to 1. Finally, we use the value method to get the value of the username field.
Step 4: Limit the number of query results
Normally, we do not need to query the specified fields in all records. We can use the limit method to limit the number of query results. For example, if we only want to query the usernames of the first 10 users, we can use the following code:
// 创建一个User模型实例 $user = new \app\model\User(); // 查询前10个用户的用户名 $usernames = $user->field('username')->limit(10)->select(); // 打印用户名 foreach ($usernames as $username) { echo $username['username'] . "<br>"; }
In the above code, we use the limit method to specify the number of query results to be 10.
Step 5: Summary
In this article, we introduced how to use ThinkPHP to query the value of a specified field. We use the select and find methods to achieve this operation. We also introduced how to use the limit method to limit the number of query results. Through these methods, we can easily query specified fields in the records that meet specific conditions in the database. I hope this article can help you better use the ThinkPHP framework.
The above is the detailed content of Let's talk about how thinkphp queries the value of a specified field. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
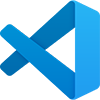
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
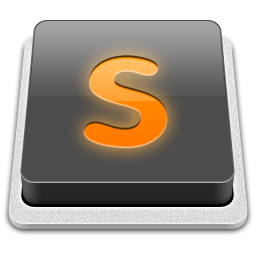
SublimeText3 Mac version
God-level code editing software (SublimeText3)
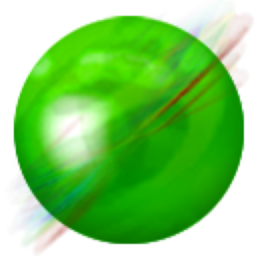
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
