In Golang programming, error handling is a very important part. It not only helps us better understand runtime errors, but also helps us optimize code and improve program robustness. However, in practical applications, we will inevitably encounter some errors, but we do not want the program to terminate due to these errors. Therefore, we need to learn how to handle and ignore these errors correctly.
1. The Importance of Error Handling
In Golang, error handling is very important. When errors occur while the program is running, if we do not handle these errors, the program will terminate abnormally, which is very unfriendly to users. More importantly, program termination will result in the loss of runtime state, which can seriously affect the correctness and robustness of the program. Therefore, handling errors correctly is the key to ensuring program correctness and stability.
In Golang, errors are usually passed through return values. Functions can return an error object to indicate whether the function executed successfully. This approach allows developers to have more detailed control over errors, thereby ensuring the safety and robustness of the program.
2. Error handling methods
There are usually two error handling methods in Golang: one is the defer recover method, and the other is the if err != nil method. Below we will introduce the use of these two methods respectively.
1. Defer recover mode
The defer recover mode can restore the running status of the program when an error occurs in the program and output the corresponding error message. This approach allows us to handle errors more flexibly without affecting the normal operation of the program.
For example, we can use defer and recover to handle file operation errors:
func readFromFile(filename string) { f, err := os.OpenFile(filename, os.O_RDONLY, 0644) if err != nil { defer func() { if x := recover(); x != nil { fmt.Printf("runtime error: %v\n", x) } }() panic(fmt.Sprintf("open file error: %v", err)) } defer f.Close() // read file content }
In this example, we use defer to execute recover after the function ends to restore the running status of the program, and at the same time use Panic throws a file open error. If the file fails to open, the program will terminate abnormally after processing. However, since we have added recovery, the program will first restore the state and then output an error message, and then continue to execute subsequent code.
2.if err != nil method
if err != nil method is a common error handling method in Golang. Typically, when performing an operation, we check the operation return value and handle errors.
For example, when printing the file content, we need to check whether the file is opened successfully:
func printFileContent(filename string) { f, err := os.OpenFile(filename, os.O_RDONLY, 0644) if err != nil { fmt.Printf("open file error: %v", err) return } defer f.Close() // read file content }
In this example, we handle the failure to open the file by checking the file opening error. If opening the file is successful, we close the file before the function ends. This approach allows us to handle errors more flexibly and ensure program stability.
3. Error ignoring situation
Of course, sometimes we also need to ignore certain errors. However, when ignoring errors, we need to be very careful and perform necessary checks and processing. Let's take a look at the situation of error ignoring in Golang:
1. End of file
When we read the file, an io.EOF error will occur when we reach the end of the file. In some cases, this error may be perfectly normal, so we can ignore this error when reading the file:
func readFromFile(filename string) { f, err := os.OpenFile(filename, os.O_RDONLY, 0644) if err != nil { fmt.Printf("open file error: %v", err) return } defer f.Close() var buf []byte for { n, err := f.Read(buf) if err == nil { fmt.Println(string(buf[:n])) continue } if err == io.EOF { break } fmt.Printf("read file error: %v", err) return } }
In this example, we ignore the io.EOF error when reaching the end of the file , and terminate the program with other errors. Doing so can increase the robustness of the program.
2. Type conversion error
During type conversion, type incompatibility may occur, such as when converting a string to a number. If this error occurs, we can choose to ignore the error and use the default value.
For example, when converting a string to an integer, we can choose to use the default value 0:
func strToInt(str string) int { num, err := strconv.Atoi(str) if err != nil { return 0 } return num }
In this example, we handle the error by judging whether err is empty, and if not The converted integer is returned on error, otherwise the default value 0 is returned. This approach allows us to handle errors very flexibly and improve the stability and robustness of the program.
3. System call errors
In Golang, some system calls may return system-level errors, such as the file cannot be opened or the file system cannot be accessed. These errors usually cannot be ignored as they may mean major problems on the system.
For example, when accessing a local disk, we need to check whether the calling operation is successful:
func checkDisk() { _, err := os.Stat("/") if err != nil { fmt.Printf("check disk error: %v", err) return } fmt.Println("disk check ok") }
In this example, we check whether the file system is available by calling the os.Stat method. If an error occurs, we will output the error message and terminate the program. Doing this can avoid program problems due to hardware failure.
4. Summary
Error handling is a very important part of Golang programming. By handling errors correctly, we can ensure the stability and robustness of the program and improve the reliability and ease of use of the program. When ignoring errors, we need to perform necessary checks and processing to avoid the impact of errors on the program. In Golang, we usually use defer recover and if err != nil to handle errors. It should be noted that error handling is very situation-dependent and requires careful analysis and evaluation when handling errors.
The above is the detailed content of How golang handles and ignores these errors correctly. For more information, please follow other related articles on the PHP Chinese website!
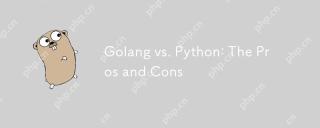
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
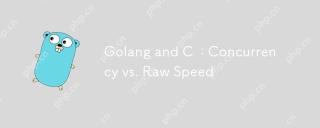
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
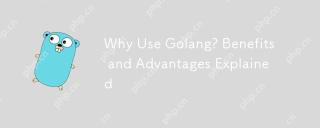
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
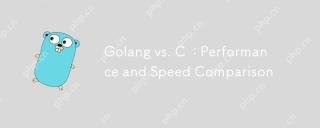
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
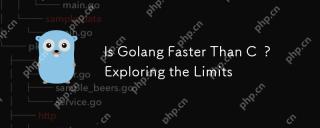
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
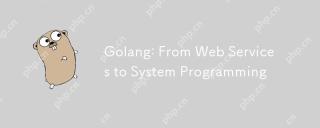
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
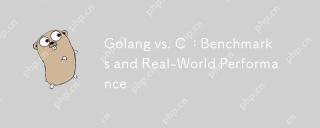
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
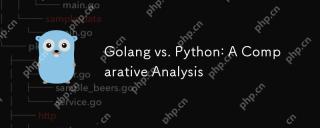
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
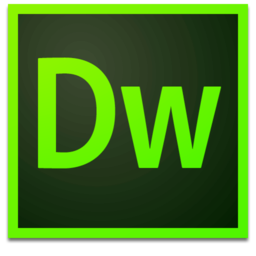
Dreamweaver Mac version
Visual web development tools
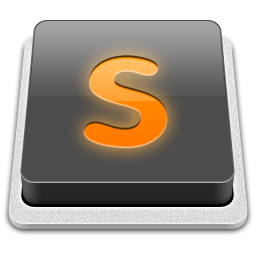
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
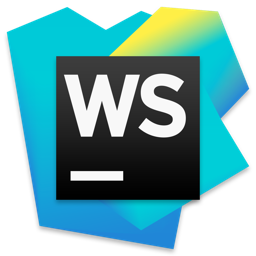
WebStorm Mac version
Useful JavaScript development tools