Golang is an efficient, simple, and reliable programming language that can handle various types of data. In web development, Golang's url escaping is a very important feature, which allows URLs to safely transmit special characters. In this article, we will discuss how to use URL escaping in Golang.
Golang's URL escaping requires the use of the built-in net/url package. There are two very important functions in this package, which are Escape and Unescape. The Escape function is used to escape non-letters and numbers in the URL, and the Unescape function is used to decode the escaped URL and restore it to the original URL.
In actual applications, when we need to send parameters to the server, we need to escape the parameters. Otherwise, the server may not be able to parse the parameters correctly. For example, there is a URL that needs to transmit the name "Bob&John?". This name contains special characters. If it is transmitted directly, the server cannot parse it correctly, so the parameter needs to be escaped. In Golang, we can use the Escape function to complete this process. The sample code is as follows:
package main import ( "fmt" "net/url" ) func main() { name := "+Bob&John?" fmt.Println(url.QueryEscape(name)) }
When we run this program, the output result is "+Bob&John?". This string is the escaped parameter we need to transmit.
When the server receives this parameter, it needs to use the Unescape function to decode it and restore it to the original parameter. The following sample code demonstrates how to decode escaped parameters:
package main import ( "fmt" "net/url" ) func main() { name := "%2BBob%26John%3F" decoded, err := url.QueryUnescape(name) if err != nil { fmt.Println(err) return } fmt.Println(decoded) }
When we run the program, the output is "Bob&John?", and this string is our original parameter.
In actual applications, we usually need to transmit multiple parameters to the server. Therefore, we need to splice these parameters according to certain rules and then escape them. In Golang, we can use url.Values to complete this process. The following sample code demonstrates how to concatenate multiple parameters and then escape them:
package main import ( "fmt" "net/url" ) func main() { values := url.Values{} values.Set("name", "+Bob&John?") values.Set("age", "20") values.Set("gender", "male") encoded := values.Encode() fmt.Println(encoded) }
When we run the program, the output result is "age=20&gender=male&name=+Bob&John?", this character The string is the escaped parameter we need to transmit.
Summary: URL escaping in Golang is a very important function that can help us transmit URL parameters safely. In Golang, we can use the Escape and Unescape functions provided in the built-in net/url package to complete URL escaping and decoding operations. We can also use url.Values to concatenate multiple parameters and then escape them.
The above is the detailed content of Discuss the use of URL escaping in Golang. For more information, please follow other related articles on the PHP Chinese website!
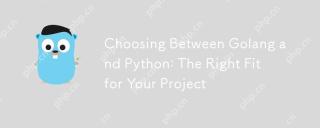
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
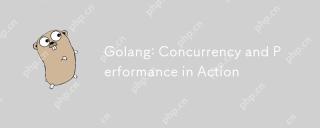
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
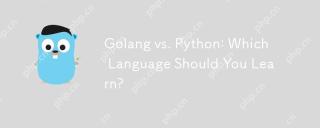
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
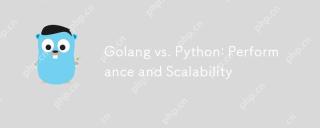
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
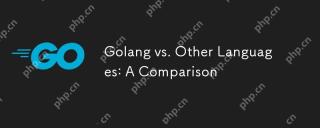
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
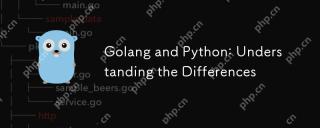
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
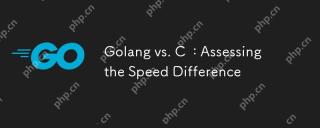
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
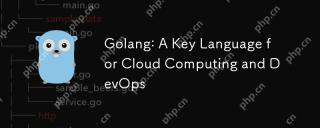
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
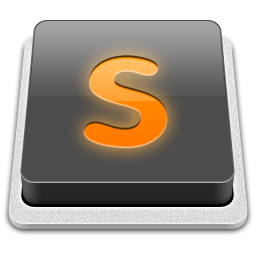
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.