Node.js is a fast, extensible JavaScript runtime whose functionality can be extended through the use of various modules. One of them is JSON (JavaScript Object Notation), a lightweight format for storing and exchanging data. In this article, I will show you how to use Node.js to add, delete, modify and query JSON data.
- Add JSON data
Node.js provides the fs module for reading and writing files. Saving JSON data to a file can be easily done using the fs module.
First, we need to create a JSON object and save it to a file. In the following code snippet, we create a students.json file and write a JSON object to the file:
const fs = require('fs'); const students = { "Tom": { "age": 18, "gender": "male" }, "Lily": { "age": 19, "gender": "female" } }; fs.writeFile('students.json', JSON.stringify(students), (err) => { if (err) throw err; console.log('The JSON data has been saved!'); });
In the above code, the method to write the file is fs.writeFile(), It requires three parameters: file name, data and callback function. The callback function accepts an error object as a parameter and returns null if the write is successful.
- Reading JSON data
Reading JSON data is also very simple. We just need to read the data from the file using the fs.readFile() method and convert it into a JSON object using the JSON.parse() method.
const fs = require('fs'); fs.readFile('students.json', (err, data) => { if (err) throw err; const students = JSON.parse(data); console.log(students); });
In the above code, we read the students.json file and convert it into a JSON object using the fs.readFile() method. The callback function accepts an error object and data as parameters. If there is an error, the err parameter will contain the error information, otherwise it will contain the contents of the file.
- Modify JSON data
To modify JSON data, we only need to use JavaScript’s object attribute access symbol (.) or square bracket access symbol ([]) to change Properties in the object.
const fs = require('fs'); fs.readFile('students.json', (err, data) => { if (err) throw err; const students = JSON.parse(data); // 修改Tom的年龄为20 students.Tom.age = 20; fs.writeFile('students.json', JSON.stringify(students), (err) => { if (err) throw err; console.log('The JSON data has been updated!'); }); });
In the above code, we read the students.json file and convert it into a JSON object. We then change Tom's age to 20 and write the changed data back to the file.
- Delete JSON data
To delete attributes from a JSON object, we can use the delete keyword.
const fs = require('fs'); fs.readFile('students.json', (err, data) => { if (err) throw err; const students = JSON.parse(data); // 删除Lily delete students.Lily; fs.writeFile('students.json', JSON.stringify(students), (err) => { if (err) throw err; console.log('The JSON data has been updated!'); }); });
In the above code, we delete the Lily property in the students object and write the changed data back to the file.
Summary
This is how to use Node.js to add, delete, modify and query JSON data. Use the fs module to read and write files, use the JSON.parse() method to convert JSON strings to JSON objects, and use the JSON.stringify() method to convert JSON objects to JSON strings. Using these methods, you can easily process JSON data so that it integrates seamlessly with your application.
The above is the detailed content of How to use Node.js to add, delete, modify and query JSON data. For more information, please follow other related articles on the PHP Chinese website!

The advantages of React are its flexibility and efficiency, which are reflected in: 1) Component-based design improves code reusability; 2) Virtual DOM technology optimizes performance, especially when handling large amounts of data updates; 3) The rich ecosystem provides a large number of third-party libraries and tools. By understanding how React works and uses examples, you can master its core concepts and best practices to build an efficient, maintainable user interface.

React is a JavaScript library for building user interfaces, suitable for large and complex applications. 1. The core of React is componentization and virtual DOM, which improves UI rendering performance. 2. Compared with Vue, React is more flexible but has a steep learning curve, which is suitable for large projects. 3. Compared with Angular, React is lighter, dependent on the community ecology, and suitable for projects that require flexibility.

React operates in HTML via virtual DOM. 1) React uses JSX syntax to write HTML-like structures. 2) Virtual DOM management UI update, efficient rendering through Diffing algorithm. 3) Use ReactDOM.render() to render the component to the real DOM. 4) Optimization and best practices include using React.memo and component splitting to improve performance and maintainability.

React is widely used in e-commerce, social media and data visualization. 1) E-commerce platforms use React to build shopping cart components, use useState to manage state, onClick to process events, and map function to render lists. 2) Social media applications interact with the API through useEffect to display dynamic content. 3) Data visualization uses react-chartjs-2 library to render charts, and component design is easy to embed applications.

Best practices for React front-end architecture include: 1. Component design and reuse: design a single responsibility, easy to understand and test components to achieve high reuse. 2. State management: Use useState, useReducer, ContextAPI or Redux/MobX to manage state to avoid excessive complexity. 3. Performance optimization: Optimize performance through React.memo, useCallback, useMemo and other methods to find the balance point. 4. Code organization and modularity: Organize code according to functional modules to improve manageability and maintainability. 5. Testing and Quality Assurance: Testing with Jest and ReactTestingLibrary to ensure the quality and reliability of the code

To integrate React into HTML, follow these steps: 1. Introduce React and ReactDOM in HTML files. 2. Define a React component. 3. Render the component into HTML elements using ReactDOM. Through these steps, static HTML pages can be transformed into dynamic, interactive experiences.

React’s popularity includes its performance optimization, component reuse and a rich ecosystem. 1. Performance optimization achieves efficient updates through virtual DOM and diffing mechanisms. 2. Component Reuse Reduces duplicate code by reusable components. 3. Rich ecosystem and one-way data flow enhance the development experience.

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
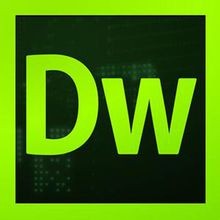
Dreamweaver CS6
Visual web development tools
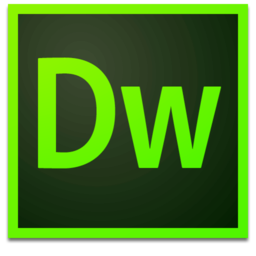
Dreamweaver Mac version
Visual web development tools