Golang is a popular programming language that is widely used in web applications, operating systems, distributed systems, cloud platforms and other fields. Among them, in string operations, we often encounter situations where multi-value replacement is required. The following will introduce how to implement multi-value replacement function in Golang.
1. Multi-value replacement of strings
In Golang, strings can be represented as Unicode code points (rune slices). Normally, we use the Replace function in the string package or the ReplaceAllString function in the Regexp package to perform single value replacement.
The usage method of Replace function is as follows:
func Replace(s, old, new string, n int) string
The usage method of ReplaceAllString function is as follows:
func ReplaceAllString(src, repl string) string
In the above function, s and src are both original strings, and old means needed The old string to be replaced, new or repl represents the new string after replacement, n represents the maximum number of replacements. However, if we want to replace multiple values at the same time, the above function cannot meet our needs. In this case, we can use the Map function in the strings package and define a mapping table in the Map function.
2. Use Map to implement multi-value replacement
The method of using the Map function is as follows:
func Map(mapping func(rune) rune, s string) string
In the above function, mapping is a function that converts each input Unicode Code points are mapped to output values, s is the string that needs to be mapped. We can set a mapping table in the mapping function to implement multi-value replacement.
The following is an example of implementing multi-value replacement:
package main import ( "fmt" "strings" ) func main() { oldStrs := []string{"world", "World"} newStrs := []string{"Go", "GoLang"} s := "Hello world! Hello World!" replacer := strings.NewReplacer(oldStrs...) fmt.Println(replacer.Replace(s)) mapping := func(r rune) rune { switch r { case 'w': return 'G' case 'o': return 'o' case 'r': return 'L' case 'l': return 'a' case 'd': return 'n' case 'W': return 'G' case '!' : return '!' default: return r } } fmt.Println(strings.Map(mapping, s)) }
In the above code, we store the string to be replaced and the replaced string in two slices oldStrs and newStrs respectively. , and passed to the NewReplacer function, through which the replacer object is created. Then, we define a mapping function in the Map function to map the old value in the original string to the new value, and use the Map function to perform an overall mapping operation on the original string to obtain the replaced string.
Run the above code, you can get the following results:
Hello Go! Hello GoLang! Hello Go! Hello GoLang!
3. Summary
In Golang, you can use the Map function in the strings package for multi-value replacement of strings. And define a mapping table in the Map function. This method can replace multiple values at the same time, making it more flexible and efficient. In actual development, it is necessary to pay attention to the order and number of different substitution values to avoid substitution errors and exceptions.
The above is the detailed content of How to implement multi-value replacement function in Golang. For more information, please follow other related articles on the PHP Chinese website!
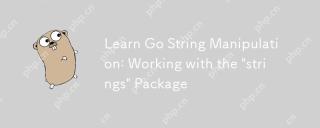
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
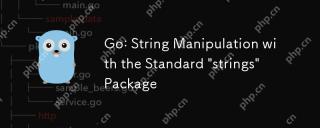
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
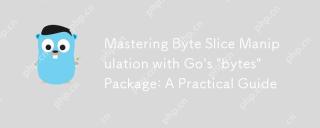
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
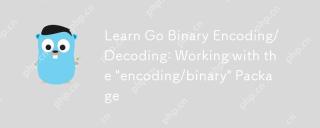
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
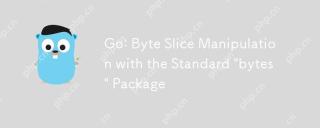
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
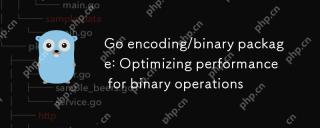
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
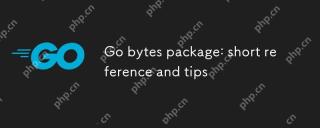
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
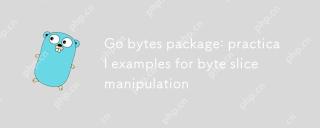
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
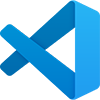
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
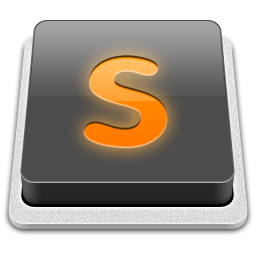
SublimeText3 Mac version
God-level code editing software (SublimeText3)
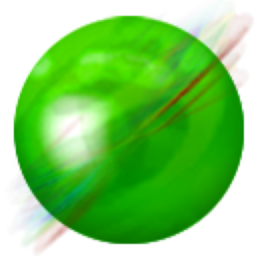
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
