In front-end development, using jQuery to dynamically add or delete page elements is a very common requirement. This article will introduce how to use jQuery to dynamically add or delete div content on the page.
1. Add div content
The most basic way to add div content is to use jQuery’s append method. This method adds a new element at the end of an existing element.
The sample code is as follows:
$(document).ready(function() { $("#addBtn").click(function() { $("#myDiv").append("<p>这是新的一行内容。</p>") }); });
In the above sample code, an event is triggered by clicking a button and a new row of p elements is added to the end of the element with the id "myDiv". This p element contains the content that needs to be added, which can be any html tag and text.
If you need to add multiple lines of content, you can change the content in the append method of the above example code to the HTML code that needs to be added. You can also use a loop to repeatedly add the same content, for example:
$(document).ready(function() { $("#addBtn").click(function() { for(var i = 0; i 这是新的一行内容。"); } }); });
In the above example code, clicking the button will add 5 new rows of p elements to the end of the element with the id "myDiv", each row containing the same html code.
2. Delete div content
The method of using jQuery to delete div content is also very simple. You can use the remove or empty method.
- remove method
The remove method can remove the selected element and all its child elements. The sample code is as follows:
$(document).ready(function() { $("#removeBtn").click(function() { $("#myDiv").remove(); }); });
In the above sample code, clicking the button will delete the element with the id "myDiv" and all its child elements.
- empty method
The empty method removes all child elements of the selected element, but retains the selected element itself. The sample code is as follows:
$(document).ready(function() { $("#emptyBtn").click(function() { $("#myDiv").empty(); }); });
In the above sample code, clicking the button will retain the element itself with the id "myDiv" but delete all the child elements of that element.
3. Summary
Through the above introduction, we can see that it is very simple to use jQuery to dynamically add or delete div content. Just use the append method to add content and the remove or empty method to delete content. In actual projects, this function is usually used to dynamically display data, add and delete form items, etc. Developers can use and adjust this feature more according to different needs.
The above is the detailed content of How to dynamically add and delete div content in jquery. For more information, please follow other related articles on the PHP Chinese website!
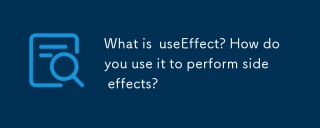
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
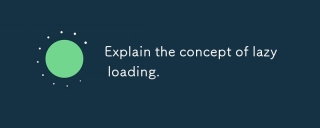
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
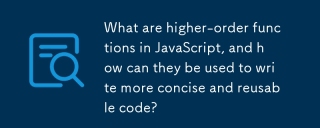
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
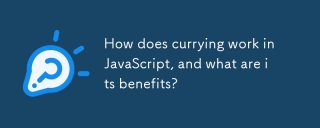
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
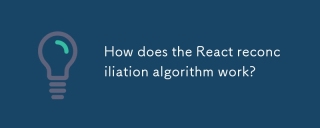
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
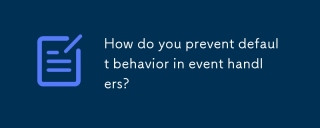
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
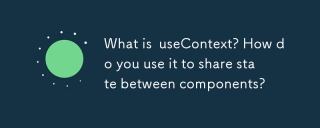
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
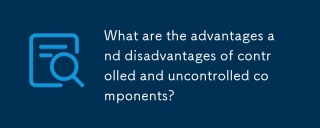
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
