In Golang, URL escaping is the process of converting a URL string into a URL-safe string. A URL string is a string that combines various characters, including letters, numbers, symbols, etc. However, HTTP URLs need to be converted into safe strings to ensure that no problems will occur during network transmission, which requires escaping the URL strings.
There are two ways to escape URL, one is to escape reserved characters, and the other is to escape non-ASCII characters. Both of these escaping methods are used to avoid errors caused by special characters in the URL string.
Escape reserved characters
Reserved characters refer to characters with special meanings in URL strings, such as spaces, vertical bars, question marks, percent signs, etc. These characters need to be escaped when used in URLs.
In Golang, URL escaping provides two methods, one is to use the Escape function in the net/url package, and the other is to use the EscapeString function. The Escape function is used to escape special characters in the URL string, and the EscapeString function is used to escape the entire URL string. The following is an example:
package main import ( "fmt" "net/url" ) func main() { urlString := "http://www.example.com/path with spaces" escapedString := url.QueryEscape(urlString) fmt.Println("Escaped string:", escapedString) }
The output result is:
Escaped string: http%3A%2F%2Fwww.example.com%2Fpath+with+spaces
As you can see, spaces are escaped to " ", and characters such as colons, slashes, and periods are escaped to other characters. The form of the ASCII code value.
Non-ASCII character escaping
In addition to reserved characters that need to be escaped, you also need to pay attention to the escaping of non-ASCII characters when using URLs. Non-ASCII characters refer to characters in the Unicode encoding table. These characters need to be escaped by converting them to the form %HH, where HH represents the hexadecimal value of the character in the Unicode encoding table.
In Golang, URL escaping non-ASCII characters requires the use of the PathEscape function in the net/url package. The sample code is as follows:
package main import ( "fmt" "net/url" ) func main() { urlString := "http://www.example.com/你好" escapedString := url.PathEscape(urlString) fmt.Println("Escaped string:", escapedString) }
The output result is:
Escaped string: http://www.example.com/%E4%BD%A0%E5%A5%BD
Yes As you can see, the non-ASCII character "hello" is escaped to the form hello, which is the hexadecimal value of this character in the Unicode encoding table.
Summary
In Golang, URL escaping is a very important skill. By escaping reserved characters and non-ASCII characters in the URL string, you can ensure that there will be no problems during network transmission. In actual development, it is necessary to have an in-depth understanding of URL escaping rules to reduce URL encoding errors.
The above is the detailed content of How to escape golang url. For more information, please follow other related articles on the PHP Chinese website!
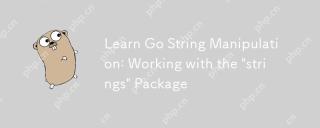
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
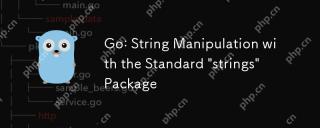
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
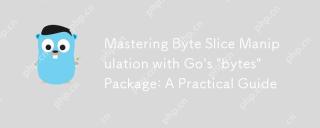
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
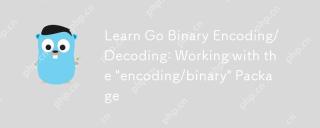
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
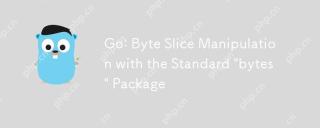
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
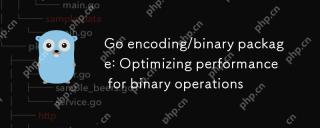
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
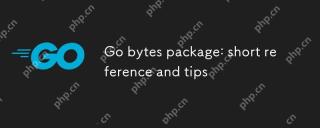
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
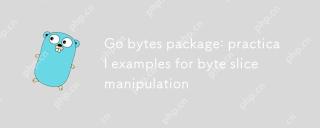
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
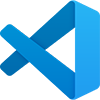
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
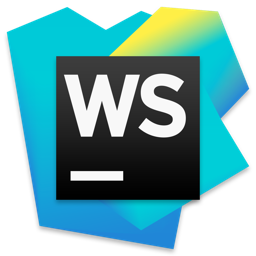
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
