


In PHP development, it is often necessary to find discontinuous numbers in a sequence of numbers. How to realize this requirement quickly and efficiently? This article will explain it to you in detail.
1. Problem background
Find discontinuous numbers in a sequence of numbers, that is, find the numbers that have a certain interval from the next number after a certain number. For example, given a sequence [1, 2, 6, 7, 9, 12, 15, 17] and asked to find discontinuous numbers, assuming the interval is 4, the return value is [2, 9, 17].
2. Problem Analysis
To realize this requirement, we need to traverse the entire number sequence and do the following processing for each number:
- Check the current number and the previous number Whether the difference of a number is equal to the specified interval. If equal, it indicates that the number is one of the discontinuous numbers; if not equal, the number is recorded as the current number.
- Add the recorded numbers to a result array, and finally return the result array.
In specific implementation, the following methods can be used:
- Define a $result array to store discontinuous numbers.
- Define a $previous variable to record the previous number.
-
Traverse the sequence of numbers and process each number.
- If the difference between the number and the previous number is equal to the specified interval, the number is added to the $result array;
- Otherwise, the number is recorded as $previous.
- Return $result array.
The specific implementation code is as follows:
function findDiscontinuousNumbers($nums, $interval) { $result = []; $previous = null; foreach ($nums as $num) { if (!is_null($previous) && $num - $previous == $interval) { $result[] = $num; } $previous = $num; } return $result; } $nums = [1, 2, 6, 7, 9, 12, 15, 17]; $interval = 4; $result = findDiscontinuousNumbers($nums, $interval); print_r($result);
3. Code optimization
The above implementation can already meet the requirements, but it may not be efficient in actual use. . Consider the following optimization:
- When a number has been recorded as a discontinuous number, the following numbers cannot be continuous with it, so $previous can be set to this discontinuous number before the next processing .
- For the search of digital sequences with large differences, during the traversal process, the position of the last discontinuous number can be recorded, and the next search can be processed directly from this position, which can reduce unnecessary traversals.
The optimized code is as follows:
function findDiscontinuousNumbers($nums, $interval) { $result = []; $previous = null; $last_discontinuous_index = null; // 上一次不连续数字的索引位置 for ($i = 0; $i <p> IV. Summary</p><p>This article briefly introduces the method of finding discontinuous numbers in PHP and gives Basic implementation. In actual use, appropriate implementation methods and optimization measures should be selected according to different needs to achieve better performance and effects. </p>
The above is the detailed content of How to find non-consecutive numbers in a sequence of numbers in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
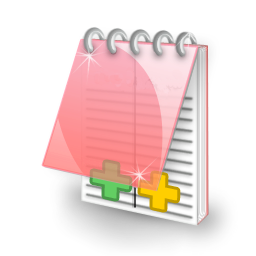
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use
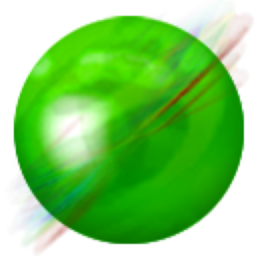
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
