Golang is a very popular programming language that focuses on simplifying the lives of developers. Manipulating JSON data is a common task in this language. JSON (JavaScript Object Notation) is a lightweight data exchange format used to transfer data between interfaces. How to transfer JSON data in Golang? Here are some tips.
The first method is to use Golang’s built-in package encoding/json. This package allows us to convert JSON to corresponding Go types, and Go types to JSON. For example, here is the sample code to convert JSON to struct:
type Person struct { Name string `json:"name"` Age int `json:"age"` Gender string `json:"gender"` } func main() { jsonString := `{"name":"Jack","age":30,"gender":"male"}` var p Person err := json.Unmarshal([]byte(jsonString), &p) if err != nil { fmt.Println("解析 JSON 出错:", err) return } fmt.Println(p) // 输出:{Jack 30 male} }
In the above code, we define a Person struct and use json.Unmarshal to parse the JSON string into the struct. It should be noted that each field in the structure needs to be annotated with the json:""
tag so that the encoder and decoder know the field name to be converted.
The second method is to use the third-party package jsoniter. This package is capable of processing JSON data faster than the standard json package. Here is a sample code for converting JSON data using jsoniter:
import "github.com/json-iterator/go" func main() { jsonString := `{"name":"Jack","age":30,"gender":"male"}` var p map[string]interface{} jsoniter.Unmarshal([]byte(jsonString), &p) fmt.Println(p) // 输出:map[age:30 gender:male name:Jack] }
In the above code, we use jsoniter.Unmarshal to parse the JSON string into a map[string]interface{} object and print the object. It should be noted that this parsing method will destroy the type information of the JSON data, so subsequent type conversion may be required.
The third method is to use the third-party package go-simplejson. This package makes it easy to manipulate JSON data and supports chaining syntax. Here is a sample code using go-simplejson:
import "github.com/bitly/go-simplejson" func main() { jsonString := `{"name":"Jack","age":30,"gender":"male"}` js, err := simplejson.NewJson([]byte(jsonString)) if err != nil { fmt.Println("解析 JSON 出错:", err) return } name := js.Get("name").MustString() age := js.Get("age").MustInt() gender := js.Get("gender").MustString() p := Person{name, age, gender} fmt.Println(p) // 输出:{Jack 30 male} }
In the above code, we use simplejson.NewJson to convert the JSON string into a simple JSON object. We can then use the Get method to get the value of the object, and the MustString and MustInt methods to get the corresponding string and integer values.
In summary, there are many methods to choose from for converting JSON data in Golang. The most commonly used one is the standard library encoding/json. If you need faster speed, you can use jsoniter. In addition, go-simplejson is also a very useful JSON library, which provides chain syntax and easy operation. Either way, you can easily manipulate JSON data.
The above is the detailed content of How to transfer JSON data in Golang. For more information, please follow other related articles on the PHP Chinese website!
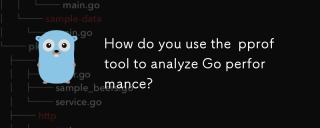
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
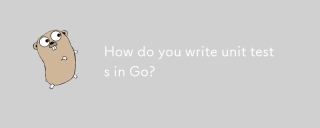
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
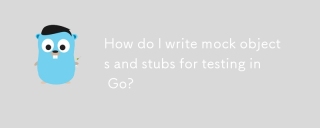
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
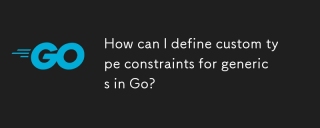
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
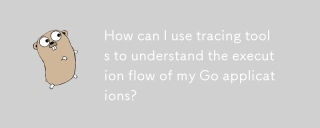
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
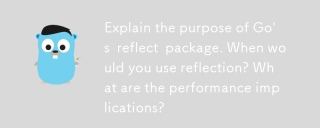
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
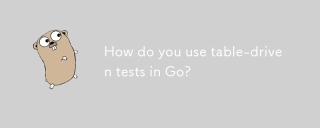
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
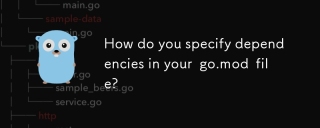
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
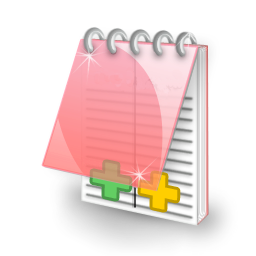
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
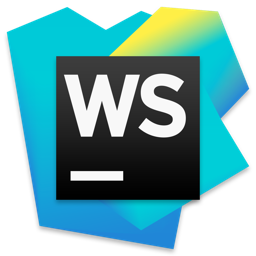
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor
