With the rapid development of Internet technology, modern applications are increasingly using JSON (JavaScript Object Notation) as the data transmission format. Golang, as an open source statically typed programming language, also provides developers with a powerful set of JSON processing tools. However, when using Golang to process JSON data, we sometimes encounter problems that require escaping.
This article will introduce you to the relevant knowledge of JSON escaping in Golang. We will explore what JSON escaping is, why JSON escaping is needed in Golang, and how to do JSON escaping in Golang.
1. What is JSON escaping?
JSON escaping refers to using the backslash character (\) in place of special characters in a JSON string. For example, special characters such as single quotes, double quotes, backspaces, newlines, etc. must be escaped using backslashes in JSON strings. This is to avoid these special characters from conflicting with the syntax of the JSON string.
2. Why is JSON escaping necessary in Golang?
When using JSON processing tools in Golang, we need to escape the JSON data. This is because Golang's JSON processing library does not escape Unicode characters by default, it only escapes reserved characters. This is done to improve efficiency and interoperability of data exchange, but it also brings some problems. For example, parsing errors are likely to occur when processing some JSON strings that require escaping Unicode characters.
3. How to escape JSON in Golang?
Golang provides some methods to handle JSON escaping. The following are several commonly used methods:
- Use Marshal method
When using JSON processing tools in Golang, you can use the Marshal method for JSON escaping. The Marshal method can serialize data into a JSON string and automatically escape special characters that need to be escaped.
The example is as follows:
type User struct { Name string `json:"name"` Age int `json:"age"` } user := User{Name: "John", Age: 18} jsonBytes, _ := json.Marshal(user) jsonStr := string(jsonBytes) fmt.Println(jsonStr)
Output result:
{"name":"John","age":18}
When using the Marshal method, the program will automatically escape the special characters in the string to make it conform to the JSON string grammar.
- Using RawMessage
The RawMessage type in Golang can serialize unknown JSON data into a string and preserve the original format. When using the RawMessage type, there is no need to manually escape the string because Golang will automatically escape all special characters that need to be escaped.
The example is as follows:
type User struct { Name string `json:"name"` Age int `json:"age"` Info json.RawMessage `json:"info"` } user := User{Name: "John", Age: 18, Info: json.RawMessage(`{"address": "Beijing", "phone": "123456789"}`)} jsonBytes, _ := json.Marshal(user) jsonStr := string(jsonBytes) fmt.Println(jsonStr)
Output result:
{"name":"John","age":18,"info":{"address": "Beijing", "phone": "123456789"}}
In this example, the RawMessage type is used to store user information, and the program automatically escapes JSON characters during serialization Special characters in the string.
4. Summary
With the popularity of JSON data, Golang provides a set of powerful JSON processing tools, which have been widely used in writing web applications. When processing JSON data, correct escaping is the prerequisite to ensure correct data transmission and accurate data parsing. JSON escaping in Golang can use the Marshal method or the RawMessage type to ensure that the data can be processed correctly during transmission and parsing.
The above is the detailed content of How to do JSON escaping in Golang. For more information, please follow other related articles on the PHP Chinese website!
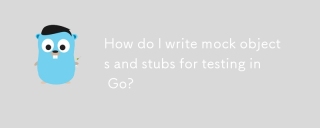
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
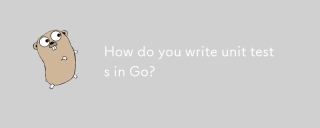
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
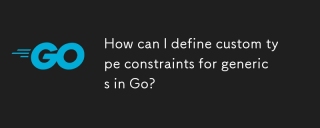
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
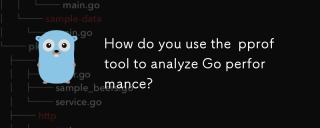
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
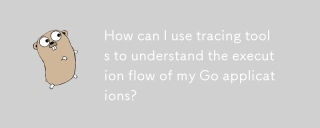
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
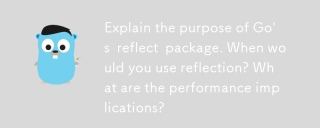
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
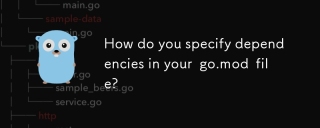
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
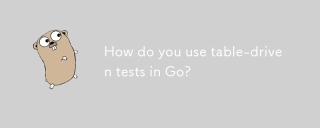
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
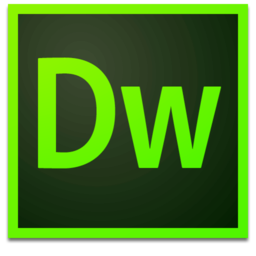
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
