Golang is a relatively new programming language that is becoming more and more popular as it continues to evolve. As Golang grows, people are developing more and more on it. In the development of Golang, query caching is a very important task. This article will discuss how to cache queries in Golang.
1. Why is query cache needed?
For a large application, data access is usually one of the most time-consuming operations. Therefore, in order to improve application performance, we need to optimize data access. A common optimization method is to use caching. By storing data in the cache, we can avoid frequently retrieving data from the database or other data sources, thereby significantly improving the response speed and performance of the application.
Caching plays a very important role in both web applications and mobile applications. Caching can not only reduce the pressure on the database, but also relieve the burden on network bandwidth and improve user experience. However, if the cache is misused or improperly used, some potential problems will occur, such as data inconsistency, cache invalidation, etc.
2. Cache query in Golang
In Golang, the query cache method is similar to other languages. Common methods include using in-memory caches, or using distributed caches such as Redis and Memcached, etc.
- Memory cache
Memory cache is a cache method based on memory implementation. In-memory cache queries are faster than other types of cache because memory access is much faster than hard disk access. In Golang, memory cache usually uses map type to store data. For example, the following code demonstrates how to use map to implement a simple in-memory cache:
package main import "fmt" func main() { cache := make(map[string]string) // 加入缓存 cache["foo"] = "bar" // 查询缓存 value, ok := cache["foo"] if ok { fmt.Println("缓存值:", value) } else { fmt.Println("缓存中不存在该值") } }
- Distributed Cache
Another common way is to use a distributed cache . Distributed cache is usually used to share data between multiple servers to avoid a single cache server becoming a bottleneck and improve cache availability. In Golang, we can use the open source cache servers Redis and Memcached to implement distributed caching.
The following code demonstrates how to use Redis in Golang to implement cached queries:
package main import ( "fmt" "github.com/go-redis/redis" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", DB: 0, }) // 设置值 err := client.Set("foo", "bar", 0).Err() if err != nil { panic(err) } // 查询缓存 value, err := client.Get("foo").Result() if err == redis.Nil { fmt.Println("缓存中不存在该值") } else if err != nil { panic(err) } else { fmt.Println("缓存值:", value) } }
3. Precautions for cached queries
When performing cached queries, there are some requirements Things to note:
- Validity period of cached data
The validity period of cached data refers to the time range within which the cached data is valid. When using cache, we need to always pay attention to this expiration date to avoid problems caused by cache invalidation. Generally speaking, the validity period of cached data should be consistent with business requirements.
- Cache hit rate
The hit rate refers to the probability of successfully obtaining data from the cache when querying the cache. The higher the hit rate, the better the cache effect. Therefore, it is very important to use the cache efficiently and improve the cache hit rate.
- Multi-level cache
In cache queries, multi-level cache is generally used to improve the hit rate. For example, we can use a combination of local cache and distributed cache, which can greatly improve the cache hit rate.
- Security of cached data
When using cache, we need to always pay attention to the security of cached data. Cache data needs to be protected from malicious tampering or theft, so we need to consider security issues such as cache encryption and identity authentication.
4. Summary
Caching queries is an important topic in Golang development. By leveraging caching, we are able to fetch data faster and benefit from offloading the database to improve application performance. When using cache, we need to pay attention to cache validity period, cache hit rate, multi-level cache, cache data security and other issues. Only when the cache is used correctly can we truly play its role, improve application performance and enhance user experience.
The above is the detailed content of Explore how to cache queries in Golang. For more information, please follow other related articles on the PHP Chinese website!
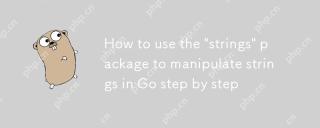
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
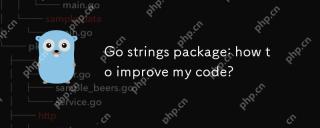
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
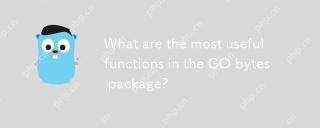
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
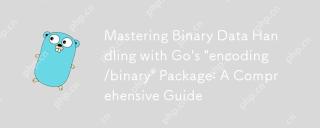
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
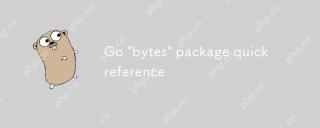
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
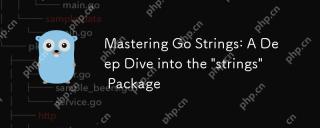
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
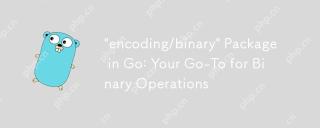
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
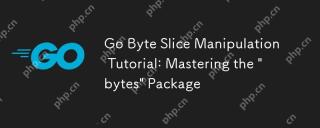
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
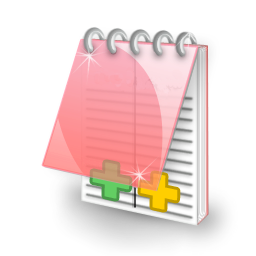
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
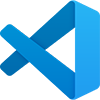
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
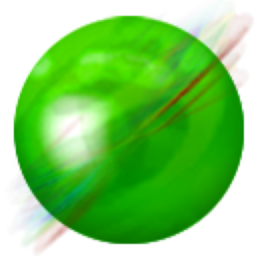
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
