Indefinite-length parameters in Golang mean that when a function is defined, the function is allowed to accept any number of parameters while ensuring that the function structure is concise and easy to read. This is a very flexible design that can be applied to a variety of situations and is very useful when writing large projects.
Generally speaking, we need to specify the parameter type and number when defining a function. For example, to define a function to calculate the sum of two integers, you can write it like this:
func add(a int, b int) int { return a + b }
This function definition is very simple and clear, and the parameter type and number are very clear. However, in some cases, we need to pass an indefinite number of parameters, such as calculating the sum of multiple integers. At this time, you can use variable length parameters.
In Golang, variable-length parameters are represented by ellipsis (...). For example, to define a function to calculate the sum of multiple integers, you can write it like this:
func add(nums ...int) int { sum := 0 for _, num := range nums { sum += num } return sum }
In this function definition, nums is a variable-length parameter, which means that any number of int type parameters can be passed. In the function body, a range loop is used to traverse the nums slice, add all parameters and return the sum.
Using variable-length parameters can greatly simplify function definition. Through variable-length parameters, the function can accept any number of parameters, and slices are used inside the function to process the passed parameters. This method is very efficient and makes the code more concise and readable. In practical applications, indefinite parameters are often used to deal with situations such as arrays and slices that require dynamic length.
In addition to using ellipses to indicate variable-length parameters, variable-length parameters can also be passed when calling functions. For example, when calling the add function, you can pass any number of int type parameters, regardless of the specific number:
sum := add(1, 2, 3, 4, 5)
This calling statement means that five int type parameters are passed to the add function, and then the add function will pass these parameters Add and return the sum. When calling a function, variable-length parameters can be used together with other parameters, which is very flexible.
The variable-length parameters in Golang are a very efficient and flexible design. With variable-length parameters, a function can accept any number of parameters without requiring the user to specify the parameter type and number in advance. This method can simplify the code and make the program more readable, understandable and efficient. In practical applications, variable-length parameters are often used to deal with dynamic length situations, which is very practical.
The above is the detailed content of In-depth analysis of variable (variable length) parameters in golang. For more information, please follow other related articles on the PHP Chinese website!
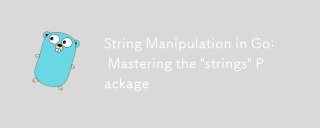
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
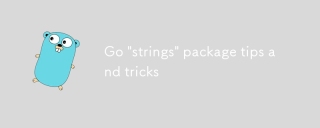
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
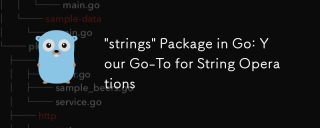
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
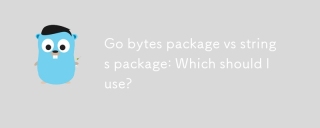
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
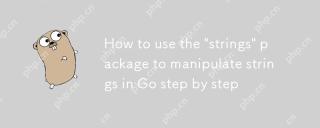
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
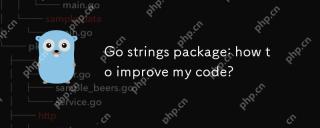
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
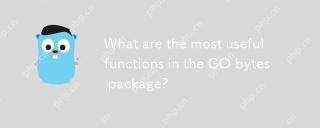
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
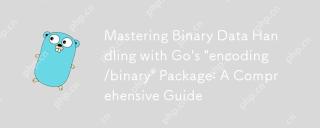
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
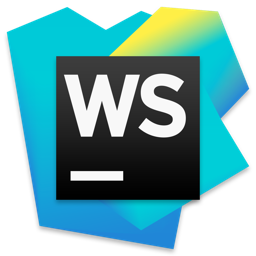
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
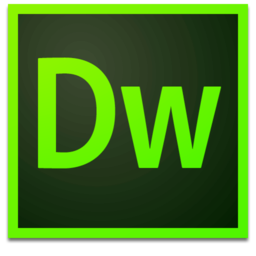
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
