


In web development, we often need to use PHP to process URLs. Sometimes, we need to remove the domain name in the URL and only keep the following path. This article will introduce how to use PHP to remove domain names from paths.
Method 1: Use parse_url function
PHP provides a very useful function parse_url to parse URLs. We can use this function to get various parts of the URL, including protocol, hostname, path, query string and fragments, etc.
We can first obtain each part of the URL through the parse_url function, and then only keep the path part. The code is as follows:
$url = 'http://www.example.com/path/to/file.html'; $path = parse_url($url, PHP_URL_PATH); echo $path; // 输出:/path/to/file.html
The above code first defines a URL string, then uses the parse_url function to obtain the path part, and finally outputs the path.
This method can effectively remove the domain name in the path, but it can only remove the host name, not the port number. If there is a port number in the URL, it will be preserved.
Method 2: Use str_replace function
In addition to using the parse_url function, we can also use PHP's built-in string replacement function str_replace. This function finds and replaces specified parts of a string.
We can define a URL string containing the host name, then use the str_replace function to replace the host name with an empty string, and finally keep only the path part. The code is as follows:
$url = 'http://www.example.com/path/to/file.html'; $path = str_replace('http://www.example.com', '', $url); echo $path; // 输出:/path/to/file.html
The above code first defines a URL string, and then uses the str_replace function to replace the host name with an empty string, thus removing the host name. Finally, we only need to output the path part.
This method can effectively remove the domain name and port number from the path, but it is only suitable for known host names. If we are processing some dynamically generated URLs, we may not be able to know the host. name.
Method 3: Use regular expressions
In addition to the above two methods, we can also use regular expressions to remove the domain name in the path. Regular expressions are a powerful text matching tool that can be used to find and replace certain parts of a string.
We can define a URL string containing the host name, and then use regular expressions to replace the host name with an empty string to remove the host name. The code is as follows:
$url = 'http://www.example.com/path/to/file.html'; $path = preg_replace('/^https?:\/\/[^\/]+/', '', $url); echo $path; // 输出:/path/to/file.html
The above code first defines a URL string, and then uses the preg_replace function to match and replace. The regular expression /^https?:\/\/[^\/] /
matches a string that begins with http:// or https:// and is followed by one or more characters that do not contain a slash. The purpose of this regular expression is to match the host name part. Use an empty string to replace the matched content, and finally get the path part minus the host name.
Note: Be very careful when using regular expressions, and be sure to ensure the accuracy of the regular expression, otherwise unpredictable errors may occur.
Summary
This article introduces three methods to remove domain names from paths in PHP: using the parse_url function, using the str_replace function and using regular expressions. Each method has its applicable scenarios and restrictions. Which method to use needs to be chosen flexibly based on the actual situation. Hope this article is helpful to readers.
The above is the detailed content of Detailed explanation of how to use PHP to remove domain names from paths. For more information, please follow other related articles on the PHP Chinese website!
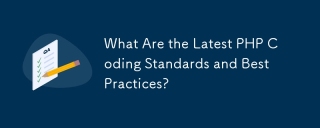
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
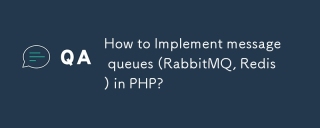
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
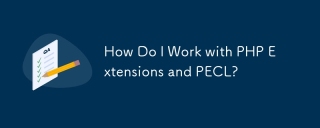
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
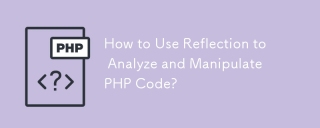
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
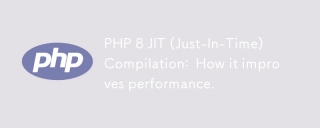
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
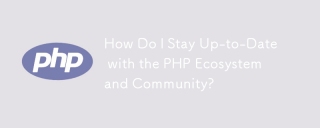
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
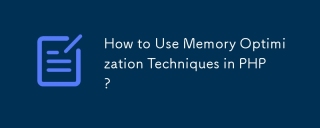
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
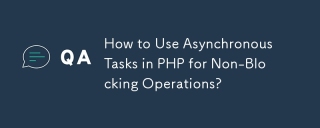
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
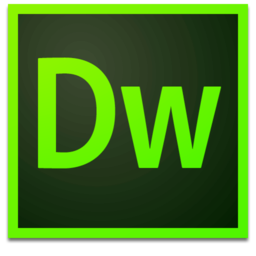
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
