PHP, as a scripting language, is very commonly used in web development, and modifying array values is also one of the common operations. This article will introduce how to modify array values through PHP.
1. Modify array elements by index
In PHP, the index of an array element can be a number or a string. We can modify the value of one of the elements through the index of the array.
Sample code:
$fruit = array('apple', 'banana', 'cherry'); //定义一个数组 $fruit[1] = 'orange'; //通过索引修改数组元素 print_r($fruit); //输出修改后的数组
Running result:
Array ( [0] => apple [1] => orange [2] => cherry )
In the above sample code, we modified it in the form of $fruit[1]
The element at index 1 in the array is changed from 'banana' to 'orange'.
2. Modify array elements through key names
In addition to modifying array elements through indexes, we can also modify the value of one of the elements through the key name of the array. This method will More convenient and in some cases more applicable.
Sample code:
$info = array('name' => 'Tom', 'age' => 20, 'gender' => 'male'); //定义一个关联数组 $info['age'] = 21; //通过键名修改数组元素 print_r($info); //输出修改后的数组
Running result:
Array ( [name] => Tom [age] => 21 [gender] => male )
In the above sample code, we use the form $info['age']
The element with the key name 'age' in the array was modified from the original 20 to 21.
3. Modify array elements through loops
In addition to the above two methods, we can also modify the element values in the array through loops. This method is suitable for situations where array elements need to be modified in batches.
Sample code:
$score = array(89, 92, 78, 85, 94); //定义一个数组 for($i=0; $i<count print_r><p>Running result: </p> <pre class="brush:php;toolbar:false">Array ( [0] => 94 [1] => 97 [2] => 83 [3] => 90 [4] => 99 )
In the above sample code, we traverse the array through a for loop and increase the value of each element by 5 .
Summary
Through the above three methods, we can modify the element values in the PHP array. Among them, the method of modifying array elements by key name is more flexible and can be changed at will according to your own needs. When modifying the element values in an array in batches through a loop, you need to pay attention to avoid the array going out of bounds. I hope this article can be helpful to PHP beginners.
The above is the detailed content of How to modify the value of an array in PHP (three methods). For more information, please follow other related articles on the PHP Chinese website!
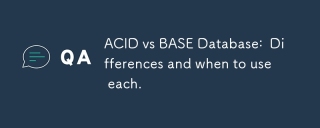
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
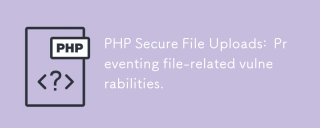
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
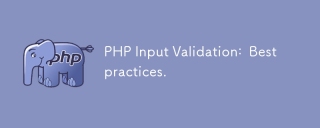
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
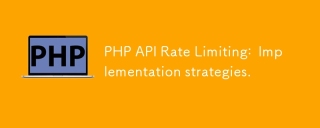
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
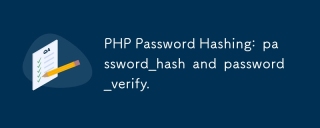
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
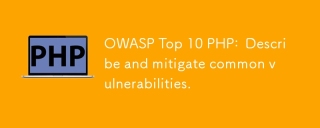
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
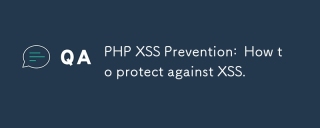
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
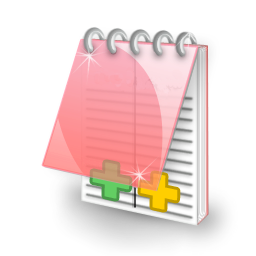
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version
