In Oracle database, a stored procedure is a reusable database object that can be called like a subroutine. Stored procedures are typically used to perform a series of database operations such as inserting, updating, deleting, and querying data. When developing Oracle database applications, stored procedures are a very important tool that can improve code reusability and performance.
In this article, we will explore how to create a simple stored procedure to create a table. The process of creating a table usually involves specifying information such as table name, column names, data types, and constraints. Using stored procedures can encapsulate this logic, making the code more modular and easier to maintain.
In Oracle database, creating stored procedures requires the use of PL/SQL language. PL/SQL is a structured programming language designed to increase the power and scalability of SQL. Through PL/SQL, we can define variables, control flow, handle exceptions, and call SQL statements.
The following is a simple stored procedure that creates a table with two columns.
CREATE OR REPLACE PROCEDURE create_table ( table_name IN VARCHAR2, column1_name IN VARCHAR2, column1_type IN VARCHAR2, column1_size IN NUMBER, column2_name IN VARCHAR2, column2_type IN VARCHAR2, column2_size IN NUMBER ) IS BEGIN EXECUTE IMMEDIATE 'CREATE TABLE ' || table_name || ' ( ' || column1_name || ' ' || column1_type || '(' || column1_size || '), ' || column2_name || ' ' || column2_type || '(' || column2_size || ') )'; END create_table;
In the above code, we use the CREATE OR REPLACE statement to create a stored procedure. CREATE OR REPLACE can be used to create new stored procedures or modify existing stored procedures. Next, we define a stored procedure called create_table that accepts seven input parameters. These parameters include the table name, the names, types and sizes of the two columns.
In the body of the stored procedure, we use the EXECUTE IMMEDIATE statement to execute dynamic SQL statements. Dynamic SQL statements are SQL statements generated when the program is running and can be used to implement functions such as dynamic tables, columns, and constraints. We use dynamic SQL statements to create tables and parameters to construct the SQL. Among them, || represents the string concatenation character, which is used to concatenate multiple strings into one string.
Next, let’s explain the meaning of each part in the stored procedure in detail.
- Stored procedure definition
CREATE OR REPLACE PROCEDURE create_table (
table_name IN VARCHAR2,
column1_name IN VARCHAR2,
column1_type IN VARCHAR2,
column1_size IN NUMBER,
column2_name IN VARCHAR2,
column2_type IN VARCHAR2,
column2_size IN NUMBER
) IS
In the stored procedure definition, we use the CREATE OR REPLACE PROCEDURE statement to create a stored procedure and specify the stored procedure name. OR REPLACE in CREATE OR REPLACE PROCEDURE means that if the stored procedure already exists, the original stored procedure will be overwritten.
In the stored procedure parameter list, we define seven parameters, among which table_name, column1_name, column1_type, column2_name and column2_type are input parameters of string type, and column1_size and column2_size are input parameters of numeric type.
- Stored procedure body
BEGIN
EXECUTE IMMEDIATE 'CREATE TABLE ' || table_name || ' (
' || column1_name || ' ' || column1_type || '(' || column1_size || '), ' || column2_name || ' ' || column2_type || '(' || column2_size || ')
)';
END create_table;
In the body of the stored procedure, we use the BEGIN and END keywords to limit the scope of the stored procedure code. Between BEGIN and END, we use the EXECUTE IMMEDIATE statement to execute dynamic SQL statements. The CREATE TABLE statement is used to create a new table, using parameters such as table name, column name, type, and size.
During the execution of the stored procedure, when the create_table stored procedure is called, seven parameters will be passed in. These parameters will be used to construct dynamic SQL statements and generate a new table. For example, if we call the create_table stored procedure and pass in the following parameters:
create_table('employees', 'id', 'NUMBER', 10, 'name', 'VARCHAR2', 50);
, a table named employees will be created, containing two columns: id and name, with data type and size of NUMBER(10 ) and VARCHAR2(50).
Summary
Stored procedure is a powerful database object that can help us implement reusable database logic and improve performance. In Oracle database, stored procedures are defined using PL/SQL language. Through stored procedures, we can encapsulate database operations such as creating tables in a process to facilitate calling and maintenance.
In this article, we introduced a simple stored procedure for creating a table with two columns. We used dynamic SQL statements and parameterized constructed SQL statements to make the stored procedures more flexible and configurable. After studying this article, I believe you have mastered the basic knowledge of Oracle stored procedures to create tables, and you can try to write more complex stored procedures to meet your business needs.
The above is the detailed content of How to create a simple stored procedure in oracle to create a table. For more information, please follow other related articles on the PHP Chinese website!

Key features of Oracle software include multi-tenant architecture, advanced analytics and data mining, real-time application clustering (RAC), and automated management and monitoring. 1) A multi-tenant architecture allows for the management of multiple independent databases in one database instance, simplifying management and reducing costs. 2) Advanced analytics and data mining tools such as Oracle Advanced Analytics and OracleDataMining help extract insights from data. 3) Real-time application cluster (RAC) provides high availability and scalability, improving system fault tolerance and performance. 4) Automated management and monitoring tools such as Oracle EnterpriseManager (OEM) to automate daily maintenance tasks and monitor numbers in real time

Oracle has a profound impact in the fields of data management and enterprise applications. Its database is known for its reliability, scalability and security, and is widely used in industries such as finance, medical care and government. Oracle's influence has also expanded to middleware and cloud computing fields such as WebLogicServer and OracleCloudInfrastructure (OCI), providing innovative solutions. Despite the competition in the open source database and cloud computing market, Oracle maintains its leading position through continuous innovation.

Oracle's mission is to "help people see the value of data", and its core values include: 1) Customer first, 2) Integrity, 3) Innovation, and 4) Teamwork. These values guide Oracle's strategic decision-making and business innovation in the market.

Oracle Database is a relational database management system that supports SQL and object relational models to provide data security and high availability. 1. The core functions of Oracle database include data storage, retrieval, security and backup and recovery. 2. Its working principle involves multi-layer storage structure, MVCC mechanism and optimizer. 3. Basic usages include creating tables, inserting and querying data; advanced usages involve stored procedures and triggers. 4. Performance optimization strategies include the use of indexes, optimized SQL statements and memory management.

In addition to database management, Oracle software is also used in JavaEE applications, data grids and high-performance computing. 1. OracleWebLogicServer is used to deploy and manage JavaEE applications. 2. OracleCoherence provides high-performance data storage and caching services. 3. OracleExadata is used for high performance computing. These tools allow Oracle to play a more diversified role in the enterprise IT architecture.

Oracle is not only a database company, but also a leader in cloud computing and ERP systems. 1. Oracle provides comprehensive solutions from database to cloud services and ERP systems. 2. OracleCloud challenges AWS and Azure, providing IaaS, PaaS and SaaS services. 3. Oracle's ERP systems such as E-BusinessSuite and FusionApplications help enterprises optimize operations.

Oracle software applications in the real world include e-commerce platforms and manufacturing. 1) On e-commerce platforms, OracleDatabase is used to store and query user information. 2) In manufacturing, OracleE-BusinessSuite is used to optimize inventory and production planning.

The reason why Oracle software shines in multiple fields is its powerful application and customized solutions. 1) Oracle provides comprehensive solutions from database management to ERP, CRM, SCM, 2) its solutions can be customized according to industry characteristics such as finance, medical care, manufacturing, etc. 3) Successful cases include Citibank, Mayo Clinic and Toyota, 4) The advantages lie in comprehensiveness, customization and scalability, but challenges include complexity, cost and integration issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
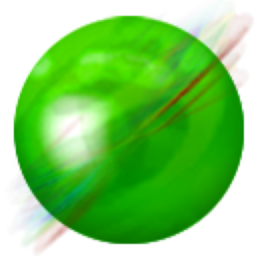
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Chinese version
Chinese version, very easy to use
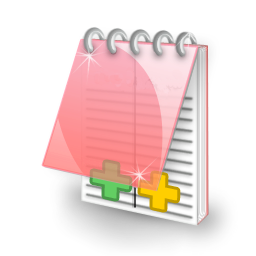
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
