PHP is a programming language widely used in Web development. Supporting Chinese character processing is one of its important features. In the process of processing Chinese characters, a common requirement is to convert Chinese characters into Pinyin and obtain the corresponding first letter of Pinyin. In this article, we will introduce how to use PHP to implement the function of converting Chinese to Pinyin, and build a simple and easy-to-use Chinese to Pinyin class.
1. Prerequisite knowledge
Before introducing the specific implementation of converting Chinese to Pinyin, we need to first understand some relevant prerequisite knowledge:
- The basics of Pinyin Concept
Pinyin is a spelling method based on the Latin alphabet that is used to express the syllables and tones of Chinese. In layman's terms, Pinyin is the "transliteration" of the Chinese language in the Latin alphabet. In mainland China, standard Mandarin uses Hanyu Pinyin.
- Methods of converting Chinese characters into Pinyin
Currently, the mainstream method of converting Chinese characters into Pinyin is to use phonetic sequence codes and letter spelling. Among them, the phonetic sequence code is a coding system formulated according to certain rules by analyzing the phonological structure of Chinese characters. Alphabet spelling is a method of spelling the pronunciation of Chinese characters and using Latin letters to represent pinyin.
2. Implementation of converting Chinese to Pinyin
After understanding the above prerequisite knowledge, we can start to introduce the specific method of using PHP to convert Chinese to Pinyin. Here, we will convert Chinese to Pinyin using alphabetical spelling, because this method is easier to understand and implement.
- Get Pinyin data
The first step is to obtain a data source that contains the mapping relationship between Chinese characters and Pinyin. Currently, there are many such data sources available online, such as Alibaba’s Pinyin data. Here, we will use another data source - Overtrue's Pinyin data.
After obtaining the data source, we need to parse it into a PHP data structure for subsequent processing. We can use the following code to convert the data into a PHP array:
$pinyin_data = file_get_contents('pinyin.json'); $pinyin_mapping = json_decode($pinyin_data, true);
Among them, pinyin.json
is the data source file we downloaded, and the json_decode
function can convert JSON Convert the formatted data into a PHP array.
- Chinese to Pinyin
After we have the Pinyin data, we can start to implement the core function of converting Chinese to Pinyin. Here we will implement a Pinyin
class, which contains two methods for converting Chinese characters into complete Pinyin and the first letter of Pinyin.
class Pinyin { private $pinyin_mapping; public function __construct($pinyin_data_file) { $pinyin_data = file_get_contents($pinyin_data_file); $this->pinyin_mapping = json_decode($pinyin_data, true); } public function convert($str, $delimiter = '', $remove_non_chinese = false) { $result = []; $regex = '/[\x{4e00}-\x{9fa5}]/u'; for ($i = 0; $i pinyin_mapping[$char][0]; $result[] = $pinyin; } else { if (!$remove_non_chinese) { $result[] = $char; } } } return implode($delimiter, $result); } public function convertInitials($str, $delimiter = '') { $result = []; $regex = '/[\x{4e00}-\x{9fa5}]/u'; for ($i = 0; $i pinyin_mapping[$char][1]; $result[] = $pinyin; } } return implode($delimiter, $result); } }
In the above code, the convert
method is used to convert Chinese characters into complete Pinyin, and the convertInitials
method is used to obtain the first letter of Pinyin. During the implementation process, we used the json_decode
function to parse the data source into a PHP array, and used the preg_match
function to determine whether the characters are Chinese characters.
When using this class, you can initialize it in the following way:
$pinyin = new Pinyin('pinyin.json');
After that, you can call the convert
and convertInitials
methods to execute Chinese is converted to Pinyin, for example:
echo $pinyin->convert('中文转拼音'); // zhōng wén zhuǎn pīn yīn echo $pinyin->convertInitials('中文转拼音'); // z w z p y
3. Summary
In this article, we introduce the specific method of using PHP to convert Chinese to Pinyin, and build a simple and easy-to-use Chinese to Pinyin. The processing of Chinese characters is an important issue in web development, and converting Chinese to Pinyin is one of the common requirements. Through the introduction of this article, I believe that readers have mastered the basic implementation methods of converting Chinese to Pinyin and can apply related technologies in actual development.
The above is the detailed content of How to use PHP to convert Chinese to Pinyin. For more information, please follow other related articles on the PHP Chinese website!
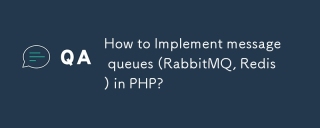
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
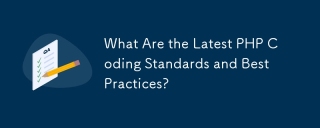
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
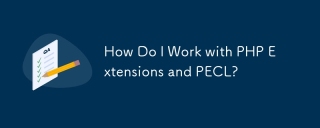
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
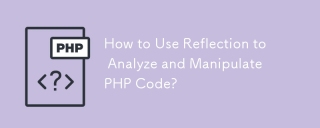
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
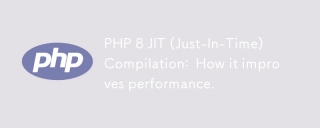
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
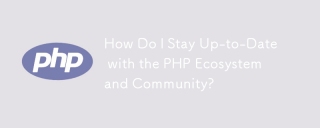
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
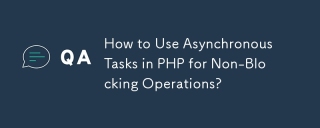
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
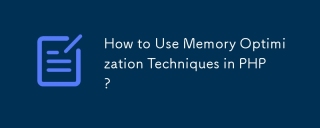
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
