In modern programming, many developers use XML (Extensible Markup Language) to store and process data. Simply put, XML is a markup language similar to HTML, which has good readability and parsability. The Go language (also known as Golang) is an increasingly popular programming language because of its efficient memory management and simple syntax.
In this article, we will discuss how to modify XML in Go language. Modifying XML is a simple but very important task, especially in large applications where updating and managing data becomes increasingly difficult. In the Go language, there are two ways to modify XML, one is to use the encoding/xml package in the standard library, and the other is to use the third-party library gokogiri.
Below, these two methods are introduced respectively.
Method 1: Use the encoding/xml package
The encoding/xml package provides a simple and efficient way to read and write XML files. It provides the xml.Unmarshal() function for parsing XML files and converting them into structured data. Once we parse the XML into structured data, we can update the XML by modifying it.
The following is a simple example that demonstrates how to use the encoding/xml package to modify an XML file:
package main import ( "encoding/xml" "fmt" "os" ) type Person struct { Name string `xml:"name"` Address string `xml:"address"` } func main() { file, err := os.Open("person.xml") if err != nil { fmt.Println("Error opening file:", err) return } defer file.Close() decoder := xml.NewDecoder(file) var p Person err = decoder.Decode(&p) if err != nil { fmt.Println("Error decoding XML:", err) return } p.Address = "New Address" file, err = os.Create("person.xml") if err != nil { fmt.Println("Error creating file:", err) return } defer file.Close() encoder := xml.NewEncoder(file) encoder.Indent("", " ") err = encoder.Encode(p) if err != nil { fmt.Println("Error encoding XML:", err) return } }
In the above example, we first open the XML file and then use xml.NewDecoder () function creates a new Decoder object, uses it to decode the XML file and convert it into a variable p of type Person. Next, we set p.Address to the new address and use the xml.NewEncoder() function to create a new Encoder object, use it to encode the variable p of type Person and write it back to the XML file.
Method 2: Using gokogiri
gokogiri is a Go language HTML/XML parser similar to the Ruby Nokogiri library. It provides a simple interface to access XML elements and attributes with good performance.
The following is a simple example that demonstrates how to use the gokogiri library to modify an XML file:
package main import ( "fmt" "github.com/moovweb/gokogiri" "github.com/moovweb/gokogiri/xml" "io/ioutil" ) func main() { content, err := ioutil.ReadFile("person.xml") if err != nil { fmt.Println("Error reading file:", err) return } doc, err := gokogiri.ParseXml(content) if err != nil { fmt.Println("Error parsing XML:", err) return } defer doc.Free() nameNode, err := doc.Search("//name") if err != nil { fmt.Println("Error searching for name node:", err) return } name := nameNode[0].FirstChild().Content() fmt.Println("Name:", name) addressNode, err := doc.Search("//address") if err != nil { fmt.Println("Error searching for address node:", err) return } addressNode[0].FirstChild().SetContent("New Address") err = ioutil.WriteFile("person.xml", []byte(doc.String()), 0644) if err != nil { fmt.Println("Error writing file:", err) return } }
In the above example, we first read the XML file and then use gokogiri.ParseXml( ) function parses it into a variable of type doc. Next, we search the XML file for name and address nodes using the doc.Search() function and access their first child node using the FirstChild() function. We can use the SetContent() function to set the content of the child node and update the address to "New Address".
Finally, we use the doc.String() function to convert the modified XML file into a string and use the ioutil.WriteFile() function to write it to the file system.
Conclusion
Go language provides two ways to modify XML files, one is through the encoding/xml package in the standard library, and the other is through the third-party library gokogiri. We can choose one of them to process XML files according to actual needs. Relatively speaking, the encoding/xml package is simpler. Since it is part of the standard library, we do not need to install any additional libraries. The gokogiri library provides more functions and can handle more complex XML files.
The above is the detailed content of How to modify xml in golang. For more information, please follow other related articles on the PHP Chinese website!
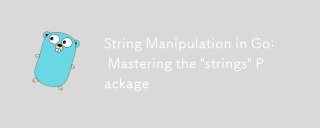
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
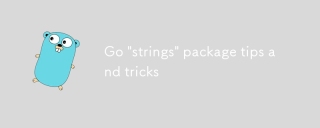
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
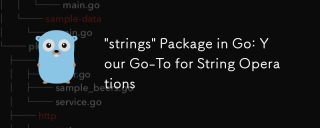
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
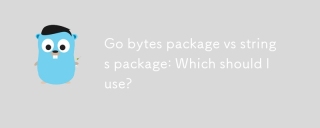
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
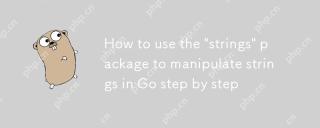
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
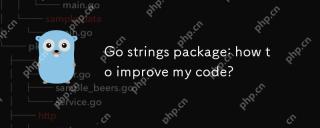
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
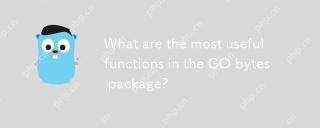
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
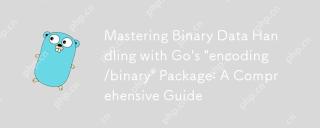
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
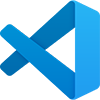
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
