Vue is one of the most popular JavaScript frameworks today and is often used to build single-page applications and websites. A common question is how to determine which container to jump to in Vue. This article will explore a few different ways to solve this problem.
- Using Vue Router
Vue Router is the official routing manager of Vue.js. It allows you to define routes for different URLs and provides a mechanism to update URLs while rendering the corresponding components into the correct container. To use Vue Router, you need to install it and add it to your Vue application.
First, install Vue Router:
npm install vue-router --save
Then, you need to add Vue Router as a plugin in your Vue application:
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter)
Next, define your routes. Let's say your Vue application has two components: Home and About. To define routes for these two components, you can write code like this:
const routes = [ { path: '/', component: Home }, { path: '/about', component: About } ]
In this example, the / path will render the Home component, and the /about path will render the About component.
Finally, create a VueRouter instance in your Vue application:
const router = new VueRouter({ routes })
Now you can use the router-link component in your Vue component to convert to the defined route:
<router-link>Home</router-link> <router-link>About</router-link>The
router-link component renders as a link that navigates the page to the specified route.
- Use Vue’s $refs attribute
Another way is to use Vue’s $refs attribute. This allows you to add references to Vue components and easily reference them when you need to access the components. To add a reference in a Vue component, use the ref attribute as shown below:
<template> <div> <div> <!-- 容器代码 --> </div> </div> </template>
In this example, we will add a reference named "container" to the div element. To access the reference, use this.$refs.container in the Vue component.
You can also use Vue's $route attribute to get the current route, and use Vue's $watch attribute to listen for route changes. This allows you to update the container when the route changes, like this:
<template> <div> <div> <!-- 容器代码 --> </div> </div> </template> <script> export default { watch: { '$route.path': function (newValue, oldValue) { this.updateContainer() } }, methods: { updateContainer: function () { // 获取当前路由 const path = this.$route.path // 获取容器元素 const container = this.$refs.container // 清空容器 container.innerHTML = '' // 根据当前路由更新容器 if (path === '/home') { // 在容器中添加Home组件 // ... } else if (path === '/about') { // 在容器中添加About组件 // ... } } } } </script>
In this example, we will use Vue's $watch property to monitor $route.path so that the updateContainer method is called when the route changes. This method gets the current route and container element and updates the container based on the current route.
- Using JavaScript's querySelector method
Finally, you can also use JavaScript's querySelector method to find the container in which to render the Vue component. querySelector allows you to select elements by ID, class, tag, etc. and then find them in the DOM.
The following is an example of using the querySelector method:
<template> <div> <div> <!-- 容器代码 --> </div> </div> </template> <script> export default { mounted: function () { // 查找容器元素 const container = document.querySelector('#container') // 根据路由更新容器 if (this.$route.path === '/home') { // 在容器中添加Home组件 // ... } else if (this.$route.path === '/about') { // 在容器中添加About组件 // ... } } } </script>
In this example, we use the querySelector method in the component's mounted lifecycle method to find the container element. We then update the container based on the current route.
Conclusion
There are many different ways to determine which container to jump to in Vue. Using Vue Router is a popular approach that allows you to define URL routes and allows you to render the correct Vue component when needed. An alternative is to use Vue's $refs property, which allows you to reference Vue components and access them when needed. Finally, you can also use JavaScript's querySelector method to find the container in which to render the Vue component. Whichever approach you choose, you should consider whether it matches the structure and needs of your Vue application.
The above is the detailed content of How does vue determine which container to jump to?. For more information, please follow other related articles on the PHP Chinese website!
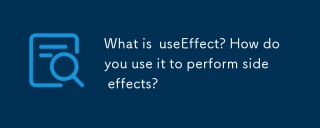
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
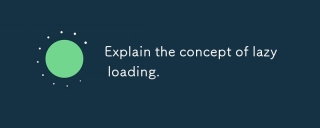
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
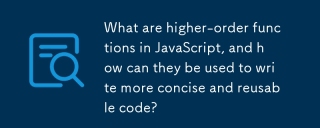
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
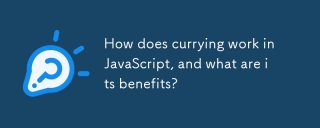
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
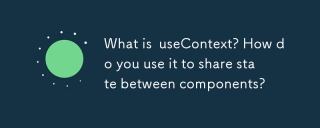
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
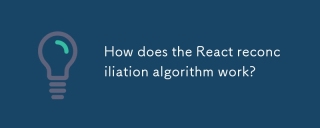
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
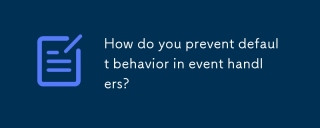
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
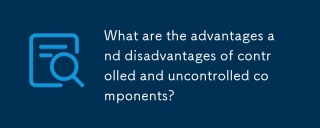
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
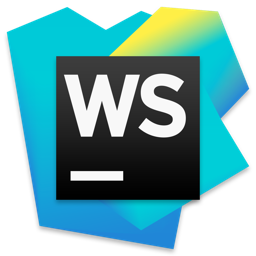
WebStorm Mac version
Useful JavaScript development tools
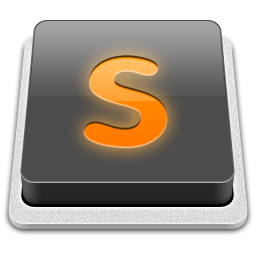
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
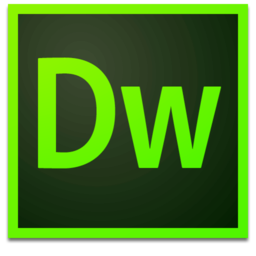
Dreamweaver Mac version
Visual web development tools
