Preface
Golang is a statically typed language, but it also provides a reflection mechanism so that the program can obtain the type information and structure information of the object at runtime and operate based on this information. In golang, reflection is used in many situations, such as dependency injection, json serialization, ORM, etc.
This article will introduce the basic concepts and common usage of golang reflection mechanism, and deepen understanding through some simple code examples. Hope readers can benefit from it.
Basic concepts of reflection mechanism
In golang, the core of the reflection mechanism is the reflect package. The reflect package provides multiple types and functions for reflecting the type information and structure information of objects at runtime. The following are some basic concepts:
- Type: Represents the type information of an object.
- Value: Represents the value information of an object.
- Kind: Represents the classification of an object type, such as int, string, map, etc.
Basic use of reflection mechanism
Get type information
In golang, use the reflect.TypeOf() function to obtain the type information of an object. The following is a simple example:
package main import ( "fmt" "reflect" ) func main() { var x float32 = 3.1415926 fmt.Println("type:", reflect.TypeOf(x)) }
In this example, we define a variable x of type float32, and use the reflect.TypeOf() function to obtain its type information. The main function will output: type: float32.
Get value information
Use the reflect.ValueOf() function to obtain the value information of an object, for example:
package main import ( "fmt" "reflect" ) func main() { var x float32 = 3.1415926 v := reflect.ValueOf(x) fmt.Println("value:", v) }
In this example, we also define a float32 Type variable x, and use the reflect.ValueOf() function to obtain its value information. The main function will output: value: 3.1415925.
Modify value information
We can modify the value information of an object through reflection. First, we need to use the reflect.ValueOf() function to obtain the value information of an object. Then, use the reflect.Value method to modify the object's value. For example:
package main import ( "fmt" "reflect" ) func main() { var x float32 = 3.1415926 v := reflect.ValueOf(&x) v.Elem().SetFloat(3.14) fmt.Println("x:", x) }
In this example, we also define a float32 type variable x and use the reflect.ValueOf() function to obtain its value information. The key is that we pass a pointer to the variable x. Then, call the Elem() method on the value information to get the value pointed to by this pointer. Finally, we call the SetFloat() method to change the value of x to 3.14 and output the new value.
Get the method of the object
We can use the reflect.TypeOf() and reflect.ValueOf() functions to obtain the method information of the object. For example:
package main import ( "fmt" "reflect" ) type User struct { Name string Age int } func (u User) Describe() { fmt.Printf("Name:%s Age:%d\n", u.Name, u.Age) } func main() { u := User{"Bob", 18} v := reflect.ValueOf(u) fmt.Println("methods:") for i := 0; i <p>In this example, we define a structure named User and create a method named Describe(). In the main function, we create a User object u and use the reflect.ValueOf() function to obtain its value information. Then we loop through all methods and print their type information. </p><p>Summary</p><p>In this article, we briefly introduce the basic concepts and common usage of golang reflection mechanism, and provide some simple code examples to deepen understanding. In fact, the reflection mechanism can do more things, such as dynamically creating objects, determining whether two objects are equal, dynamically calling methods, etc. If you want to learn more about the reflection mechanism, it is recommended to read the official documentation and more advanced cases. </p>
The above is the detailed content of Basic concepts and common usage of golang reflection mechanism. For more information, please follow other related articles on the PHP Chinese website!
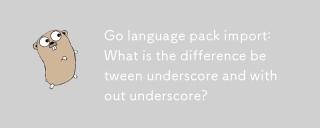
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
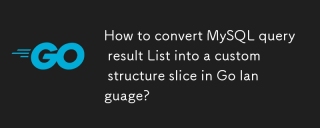
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
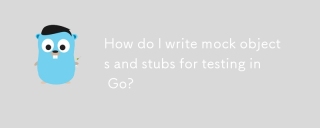
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
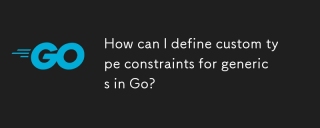
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
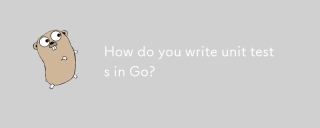
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
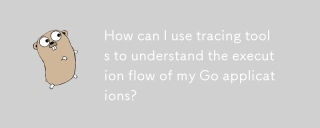
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
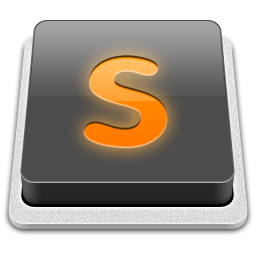
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
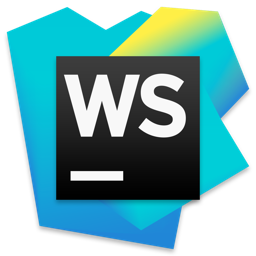
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
