Golang is an efficient programming language, which is characterized by strong readability, simple code writing, and extremely high execution speed. As a Golang developer, we often encounter situations where we need to operate on strings, and Golang provides many convenient functions and methods to meet this need. One of the common needs is to replace the content in a string. In this article, we will discuss string replacement operations in Golang.
First, let’s take a look at the most basic string replacement operation. The strings package in Golang provides the Replace() function to perform string replacement. The Replace() function accepts three parameters: the source string, the string to be replaced, and the replaced string. The following is a sample code implementation:
package main import ( "fmt" "strings" ) func main() { str := "Hello,World!" newStr := strings.Replace(str, "World", "Golang", -1) fmt.Println(newStr) }
In the above example, we use the strings.Replace() function to replace the string "World" with "Golang". The third parameter of the function -1 means to replace all matching strings. If we want to replace only the first matching string, we can set the third parameter to 1:
newStr := strings.Replace(str, "World", "Golang", 1)
Next let's see how to implement a more flexible and complex string replacement.
Regular expression replacement
Regular expression is a powerful string matching tool. In Golang, the regexp package provides support for regular expressions. We can use regular expressions to achieve more flexible and complex string replacement operations. The following is a sample code that implements replacing numbers with letters:
package main import ( "fmt" "regexp" ) func main() { str := "123abc" re := regexp.MustCompile(`\d+`) newStr := re.ReplaceAllStringFunc(str, func(s string) string { r := []rune(s) return string(r[0] + 49) }) fmt.Println(newStr) }
In the above example, we use the ReplaceAllStringFunc() function provided by the regexp package to replace strings. The ReplaceAllStringFunc() function accepts two parameters: the source string and the callback function. The callback function accepts a parameter string and returns the replaced string. In this example, we use the regular expression \d
to match numbers, and then replace each number with the letter after it in the callback function, such as 0 is replaced with a, 1 is replaced with b, to And so on.
Custom replacement function
In the above example, we used regular expressions to match strings. But sometimes we don't want to use regular expressions, but need to write a custom replacement function to complete the replacement operation. The following is a sample code that converts uppercase letters to lowercase letters:
package main import ( "fmt" "unicode" ) func main() { str := "Hello,World!" newStr := ReplaceFunc(str, func(r rune) rune { if unicode.IsUpper(r) { return unicode.ToLower(r) } return r }) fmt.Println(newStr) } func ReplaceFunc(str string, f func(rune) rune) string { runes := []rune(str) for i, c := range runes { runes[i] = f(c) } return string(runes) }
In the above example, we wrote a custom replacement function ReplaceFunc(). The ReplaceFunc() function accepts two parameters: a string and a callback function. The callback function accepts a rune type parameter and returns the replaced rune type, and then uses this callback function to replace each character in the string.
Summary
In Golang, we can use the strings package, regexp package and custom replacement functions to implement flexible and complex string replacement operations. These functions and methods provide many practical functions to efficiently complete string replacement operations. Hope this article can be helpful to you.
The above is the detailed content of Discuss string replacement operations in Golang. For more information, please follow other related articles on the PHP Chinese website!
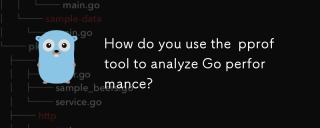
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
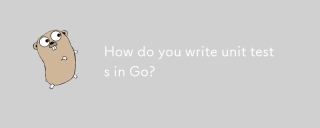
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
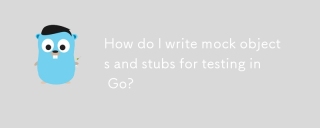
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
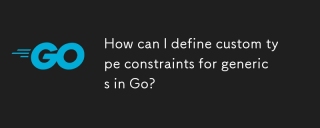
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
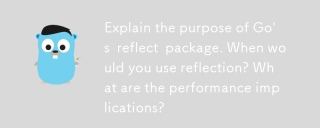
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
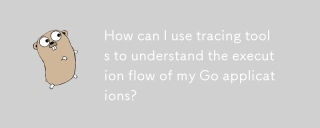
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
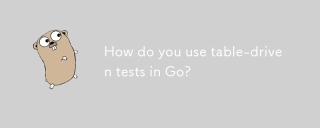
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
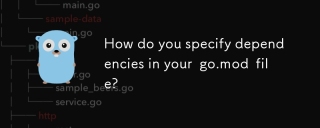
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
