When writing network applications, you often encounter situations where you need to set a timeout mechanism. The timeout mechanism refers to waiting for an operation to be completed within a certain period of time, such as waiting for a remote request response or waiting for an event to occur. As an efficient language, Go language also provides a relatively simple and easy-to-use timeout mechanism implementation. This article will introduce how to use Golang to implement the timeout mechanism.
What is the timeout mechanism?
Before understanding the timeout mechanism, let’s first take a look at what blocking operations are. A blocking operation means that an operation is stalled for some reason and cannot continue. For example, waiting for a response to a network request, waiting for a response from an I/O device, etc.
The timeout mechanism is to specify a time period when performing a blocking operation. If the operation is not completed within the specified time, the operation will be actively ended and an error message will be returned. The advantage of this is that in some cases, we need to avoid blocking operations for too long, which will lead to problems such as the user interface hanging or the client not receiving a response for a long time.
Golang’s timeout mechanism implementation
In Golang, we can implement the timeout mechanism through Goroutines and Channel. These two methods will be introduced below.
Goroutine implements timeout
Goroutine in Go language is equivalent to a lightweight thread that can coordinate concurrent tasks. When a timeout mechanism is needed, we can use Goroutine to implement it.
The following is a sample code that uses Goroutine to implement the timeout mechanism:
package main import ( "fmt" "time" ) func main() { data := make(chan int) done := make(chan struct{}) go func() { for { select { case d := <p>The above code uses Goroutine to implement the timeout mechanism. When receiving data (d := </p><p>When the program is running, monitor between data and timer through the select statement. When data is received, select will jump out; when timeout occurs, information will be printed and done is closed. After receiving 5 pieces of data, the program closes done and prints Done to indicate the end of the timeout mechanism. </p><h3 id="Channel-implements-timeout">Channel implements timeout</h3><p>Channel in Go language is a communication method that can coordinate concurrent tasks. In operations that require timeout, we can also use Channel to implement it. </p><p>The following is a sample code that uses Channel to implement the timeout mechanism: </p><pre class="brush:php;toolbar:false">package main import ( "fmt" "time" ) func main() { data := make(chan int, 1) timeOut := make(chan bool, 1) go func() { time.Sleep(time.Second * 2) timeOut <p>The above code uses Channel to implement the timeout mechanism, sends data to the data pipeline (capacity is 1), and then in the select statement Monitor. After reading the data in the channel, the operation of printing the data can be triggered. And if it times out, it will no longer block and wait, but trigger the timeout branch. </p><p>Since the capacities of timeOut and data pipes are both 1, data writing will be blocked until a reader reads the data or times out. TimeOut writes data after two seconds of timeout, triggering a read operation. </p><p>The method of implementing the timeout mechanism through Channel is more straightforward than Goroutine, but it should be noted that the data buffer size must be limited to 1, otherwise the expected timeout effect will not be achieved. </p><h2 id="Summary">Summary</h2><p>This article introduces two ways to use Golang to implement the timeout mechanism: using Goroutine and Channel. Both methods can implement the timeout mechanism well, which method to choose depends on the actual needs. When using the timeout mechanism, we need to make different choices based on different scenarios to improve the robustness and security of the application. </p>
The above is the detailed content of How to implement timeout mechanism using Golang. For more information, please follow other related articles on the PHP Chinese website!
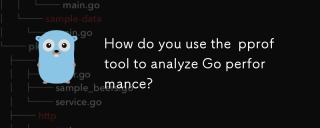
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
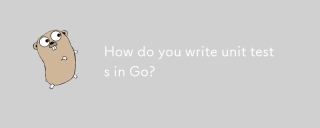
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
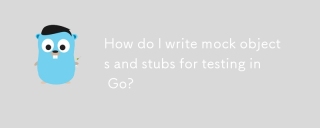
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
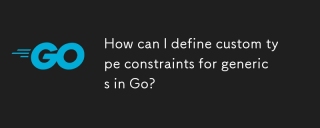
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
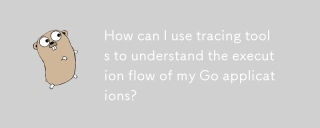
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
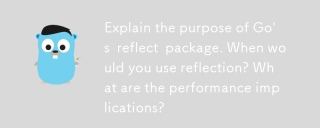
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
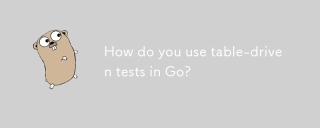
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
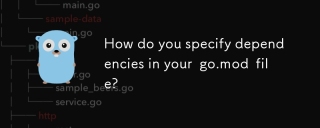
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
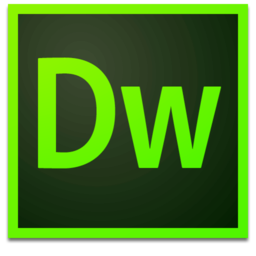
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
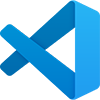
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
